Modifying Cluster Specifications
Function
This API is used to modify the specifications of a cluster.
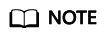
-
The URL for cluster management is in the format of https://Endpoint/uri, in which uri indicates the resource path, that is, the path for API access.
-
For details about constraints, see Changing Cluster Scale.
Calling Method
For details, see Calling APIs.
URI
POST /api/v3/projects/{project_id}/clusters/{cluster_id}/operation/resize
Parameter |
Mandatory |
Type |
Description |
---|---|---|---|
project_id |
Yes |
String |
Details: Project ID. For details about how to obtain the value, see How to Obtain Parameters in the API URI. Constraints: None Options: Project IDs of the account Default value: N/A |
cluster_id |
Yes |
String |
Details: Cluster ID. For details about how to obtain the value, see How to Obtain Parameters in the API URI. Constraints: None Options: Cluster IDs Default value: N/A |
Request Parameters
Parameter |
Mandatory |
Type |
Description |
---|---|---|---|
Content-Type |
Yes |
String |
Details: Request body type or format Constraints: The GET method is not verified. Options:
Default value: N/A |
X-Auth-Token |
Yes |
String |
Details: Requests for calling an API can be authenticated using either a token or AK/SK. If token-based authentication is used, this parameter is mandatory and must be set to a user token. For details, see Obtaining a User Token. Constraints: None Options: N/A Default value: N/A |
Parameter |
Mandatory |
Type |
Description |
---|---|---|---|
flavorResize |
Yes |
String |
The target flavor to be changed. You can only adjust the maximum number of worker nodes in a cluster. The number of master nodes is fixed and cannot be altered, and the cluster flavors cannot be downgraded. Suppose the original cluster flavor is cce.s2.medium. In that case, you can only upgrade it to cce.s2.large or higher, but you cannot downgrade it to cce.s2.small or cce.s1.medium.
NOTE:
The fields in the parameters are described as follows:
|
extendParam |
No |
extendParam object |
Extended field for changing cluster specifications |
Parameter |
Mandatory |
Type |
Description |
---|---|---|---|
decMasterFlavor |
No |
String |
Specifications of the master node in the dedicated hybrid cluster |
isAutoPay |
No |
String |
Whether auto payment is enabled.
NOTE:
This field is valid for yearly/monthly clusters. If not specified, auto payment is not enabled. |
Response Parameters
Status code: 201
Parameter |
Type |
Description |
---|---|---|
jobID |
String |
ID of the job |
orderID |
String |
ID of the order for modifying the specifications of a yearly/monthly billed cluster |
Example Requests
-
Modifying the specifications of the yearly/monthly cluster (automatic payment)
POST /api/v3/projects/{project_id}/clusters/{cluster_id}/operation/resize { "flavorResize" : "cce.s1.medium", "extendParam" : { "isAutoPay" : "true" } }
-
Modifying the specifications of the pay-per-use cluster
POST /api/v3/projects/{project_id}/clusters/{cluster_id}/operation/resize { "flavorResize" : "cce.s1.medium" }
Example Responses
Status code: 201
The job for modifying the specifications of the pay-per-use cluster is delivered.
{ "jobID" : "13b8d958-8fcf-11ed-aef3-0255ac1001bd" }
SDK Sample Code
The SDK sample code is as follows.
-
Modifying the specifications of the yearly/monthly cluster (automatic payment)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54
package com.huaweicloud.sdk.test; import com.huaweicloud.sdk.core.auth.ICredential; import com.huaweicloud.sdk.core.auth.BasicCredentials; import com.huaweicloud.sdk.core.exception.ConnectionException; import com.huaweicloud.sdk.core.exception.RequestTimeoutException; import com.huaweicloud.sdk.core.exception.ServiceResponseException; import com.huaweicloud.sdk.cce.v3.region.CceRegion; import com.huaweicloud.sdk.cce.v3.*; import com.huaweicloud.sdk.cce.v3.model.*; public class ResizeClusterSolution { public static void main(String[] args) { // The AK and SK used for authentication are hard-coded or stored in plaintext, which has great security risks. It is recommended that the AK and SK be stored in ciphertext in configuration files or environment variables and decrypted during use to ensure security. // In this example, AK and SK are stored in environment variables for authentication. Before running this example, set environment variables CLOUD_SDK_AK and CLOUD_SDK_SK in the local environment String ak = System.getenv("CLOUD_SDK_AK"); String sk = System.getenv("CLOUD_SDK_SK"); String projectId = "{project_id}"; ICredential auth = new BasicCredentials() .withProjectId(projectId) .withAk(ak) .withSk(sk); CceClient client = CceClient.newBuilder() .withCredential(auth) .withRegion(CceRegion.valueOf("<YOUR REGION>")) .build(); ResizeClusterRequest request = new ResizeClusterRequest(); request.withClusterId("{cluster_id}"); ResizeClusterRequestBody body = new ResizeClusterRequestBody(); ResizeClusterRequestBodyExtendParam extendParambody = new ResizeClusterRequestBodyExtendParam(); extendParambody.withIsAutoPay("true"); body.withExtendParam(extendParambody); body.withFlavorResize("cce.s1.medium"); request.withBody(body); try { ResizeClusterResponse response = client.resizeCluster(request); System.out.println(response.toString()); } catch (ConnectionException e) { e.printStackTrace(); } catch (RequestTimeoutException e) { e.printStackTrace(); } catch (ServiceResponseException e) { e.printStackTrace(); System.out.println(e.getHttpStatusCode()); System.out.println(e.getRequestId()); System.out.println(e.getErrorCode()); System.out.println(e.getErrorMsg()); } } }
-
Modifying the specifications of the pay-per-use cluster
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51
package com.huaweicloud.sdk.test; import com.huaweicloud.sdk.core.auth.ICredential; import com.huaweicloud.sdk.core.auth.BasicCredentials; import com.huaweicloud.sdk.core.exception.ConnectionException; import com.huaweicloud.sdk.core.exception.RequestTimeoutException; import com.huaweicloud.sdk.core.exception.ServiceResponseException; import com.huaweicloud.sdk.cce.v3.region.CceRegion; import com.huaweicloud.sdk.cce.v3.*; import com.huaweicloud.sdk.cce.v3.model.*; public class ResizeClusterSolution { public static void main(String[] args) { // The AK and SK used for authentication are hard-coded or stored in plaintext, which has great security risks. It is recommended that the AK and SK be stored in ciphertext in configuration files or environment variables and decrypted during use to ensure security. // In this example, AK and SK are stored in environment variables for authentication. Before running this example, set environment variables CLOUD_SDK_AK and CLOUD_SDK_SK in the local environment String ak = System.getenv("CLOUD_SDK_AK"); String sk = System.getenv("CLOUD_SDK_SK"); String projectId = "{project_id}"; ICredential auth = new BasicCredentials() .withProjectId(projectId) .withAk(ak) .withSk(sk); CceClient client = CceClient.newBuilder() .withCredential(auth) .withRegion(CceRegion.valueOf("<YOUR REGION>")) .build(); ResizeClusterRequest request = new ResizeClusterRequest(); request.withClusterId("{cluster_id}"); ResizeClusterRequestBody body = new ResizeClusterRequestBody(); body.withFlavorResize("cce.s1.medium"); request.withBody(body); try { ResizeClusterResponse response = client.resizeCluster(request); System.out.println(response.toString()); } catch (ConnectionException e) { e.printStackTrace(); } catch (RequestTimeoutException e) { e.printStackTrace(); } catch (ServiceResponseException e) { e.printStackTrace(); System.out.println(e.getHttpStatusCode()); System.out.println(e.getRequestId()); System.out.println(e.getErrorCode()); System.out.println(e.getErrorMsg()); } } }
-
Modifying the specifications of the yearly/monthly cluster (automatic payment)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39
# coding: utf-8 import os from huaweicloudsdkcore.auth.credentials import BasicCredentials from huaweicloudsdkcce.v3.region.cce_region import CceRegion from huaweicloudsdkcore.exceptions import exceptions from huaweicloudsdkcce.v3 import * if __name__ == "__main__": # The AK and SK used for authentication are hard-coded or stored in plaintext, which has great security risks. It is recommended that the AK and SK be stored in ciphertext in configuration files or environment variables and decrypted during use to ensure security. # In this example, AK and SK are stored in environment variables for authentication. Before running this example, set environment variables CLOUD_SDK_AK and CLOUD_SDK_SK in the local environment ak = os.environ["CLOUD_SDK_AK"] sk = os.environ["CLOUD_SDK_SK"] projectId = "{project_id}" credentials = BasicCredentials(ak, sk, projectId) client = CceClient.new_builder() \ .with_credentials(credentials) \ .with_region(CceRegion.value_of("<YOUR REGION>")) \ .build() try: request = ResizeClusterRequest() request.cluster_id = "{cluster_id}" extendParambody = ResizeClusterRequestBodyExtendParam( is_auto_pay="true" ) request.body = ResizeClusterRequestBody( extend_param=extendParambody, flavor_resize="cce.s1.medium" ) response = client.resize_cluster(request) print(response) except exceptions.ClientRequestException as e: print(e.status_code) print(e.request_id) print(e.error_code) print(e.error_msg)
-
Modifying the specifications of the pay-per-use cluster
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
# coding: utf-8 import os from huaweicloudsdkcore.auth.credentials import BasicCredentials from huaweicloudsdkcce.v3.region.cce_region import CceRegion from huaweicloudsdkcore.exceptions import exceptions from huaweicloudsdkcce.v3 import * if __name__ == "__main__": # The AK and SK used for authentication are hard-coded or stored in plaintext, which has great security risks. It is recommended that the AK and SK be stored in ciphertext in configuration files or environment variables and decrypted during use to ensure security. # In this example, AK and SK are stored in environment variables for authentication. Before running this example, set environment variables CLOUD_SDK_AK and CLOUD_SDK_SK in the local environment ak = os.environ["CLOUD_SDK_AK"] sk = os.environ["CLOUD_SDK_SK"] projectId = "{project_id}" credentials = BasicCredentials(ak, sk, projectId) client = CceClient.new_builder() \ .with_credentials(credentials) \ .with_region(CceRegion.value_of("<YOUR REGION>")) \ .build() try: request = ResizeClusterRequest() request.cluster_id = "{cluster_id}" request.body = ResizeClusterRequestBody( flavor_resize="cce.s1.medium" ) response = client.resize_cluster(request) print(response) except exceptions.ClientRequestException as e: print(e.status_code) print(e.request_id) print(e.error_code) print(e.error_msg)
-
Modifying the specifications of the yearly/monthly cluster (automatic payment)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46
package main import ( "fmt" "github.com/huaweicloud/huaweicloud-sdk-go-v3/core/auth/basic" cce "github.com/huaweicloud/huaweicloud-sdk-go-v3/services/cce/v3" "github.com/huaweicloud/huaweicloud-sdk-go-v3/services/cce/v3/model" region "github.com/huaweicloud/huaweicloud-sdk-go-v3/services/cce/v3/region" ) func main() { // The AK and SK used for authentication are hard-coded or stored in plaintext, which has great security risks. It is recommended that the AK and SK be stored in ciphertext in configuration files or environment variables and decrypted during use to ensure security. // In this example, AK and SK are stored in environment variables for authentication. Before running this example, set environment variables CLOUD_SDK_AK and CLOUD_SDK_SK in the local environment ak := os.Getenv("CLOUD_SDK_AK") sk := os.Getenv("CLOUD_SDK_SK") projectId := "{project_id}" auth := basic.NewCredentialsBuilder(). WithAk(ak). WithSk(sk). WithProjectId(projectId). Build() client := cce.NewCceClient( cce.CceClientBuilder(). WithRegion(region.ValueOf("<YOUR REGION>")). WithCredential(auth). Build()) request := &model.ResizeClusterRequest{} request.ClusterId = "{cluster_id}" isAutoPayExtendParam:= "true" extendParambody := &model.ResizeClusterRequestBodyExtendParam{ IsAutoPay: &isAutoPayExtendParam, } request.Body = &model.ResizeClusterRequestBody{ ExtendParam: extendParambody, FlavorResize: "cce.s1.medium", } response, err := client.ResizeCluster(request) if err == nil { fmt.Printf("%+v\n", response) } else { fmt.Println(err) } }
-
Modifying the specifications of the pay-per-use cluster
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41
package main import ( "fmt" "github.com/huaweicloud/huaweicloud-sdk-go-v3/core/auth/basic" cce "github.com/huaweicloud/huaweicloud-sdk-go-v3/services/cce/v3" "github.com/huaweicloud/huaweicloud-sdk-go-v3/services/cce/v3/model" region "github.com/huaweicloud/huaweicloud-sdk-go-v3/services/cce/v3/region" ) func main() { // The AK and SK used for authentication are hard-coded or stored in plaintext, which has great security risks. It is recommended that the AK and SK be stored in ciphertext in configuration files or environment variables and decrypted during use to ensure security. // In this example, AK and SK are stored in environment variables for authentication. Before running this example, set environment variables CLOUD_SDK_AK and CLOUD_SDK_SK in the local environment ak := os.Getenv("CLOUD_SDK_AK") sk := os.Getenv("CLOUD_SDK_SK") projectId := "{project_id}" auth := basic.NewCredentialsBuilder(). WithAk(ak). WithSk(sk). WithProjectId(projectId). Build() client := cce.NewCceClient( cce.CceClientBuilder(). WithRegion(region.ValueOf("<YOUR REGION>")). WithCredential(auth). Build()) request := &model.ResizeClusterRequest{} request.ClusterId = "{cluster_id}" request.Body = &model.ResizeClusterRequestBody{ FlavorResize: "cce.s1.medium", } response, err := client.ResizeCluster(request) if err == nil { fmt.Printf("%+v\n", response) } else { fmt.Println(err) } }
For SDK sample code of more programming languages, see the Sample Code tab in API Explorer. SDK sample code can be automatically generated.
Status Codes
Status Code |
Description |
---|---|
201 |
The job for modifying the specifications of the pay-per-use cluster is delivered. |
Error Codes
See Error Codes.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot