Readiness Probe
After a pod is created, the Service can immediately select it and forward requests to it. However, it takes time to start a pod. If the pod is not ready (it takes time to load the configuration or data, or a preheating program may need to be executed), the pod cannot process requests, and the requests will fail.
Kubernetes solves this problem by adding a readiness probe to pods. A pod with containers reporting that they are not ready does not receive traffic through Kubernetes Services.
A readiness probe periodically detects a pod and determines whether the pod is ready based on its response. Similar to Liveness Probe, there are three types of readiness probes.
- Exec: kubelet executes a command in the target container. If the command succeeds, it returns 0, and kubelet considers the container to be ready.
- HTTP GET: The probe sends an HTTP GET request to IP:port of the container. If the probe receives a 2xx or 3xx status code, the container is considered to be ready.
- TCP Socket: The kubelet attempts to establish a TCP connection with the container. If it succeeds, the container is considered ready.
How Readiness Probes Work
Endpoints can be used as a readiness probe. When a pod is not ready, the IP:port of the pod is deleted from the Endpoint and is added to the Endpoint after the pod is ready, as shown in the following figure.
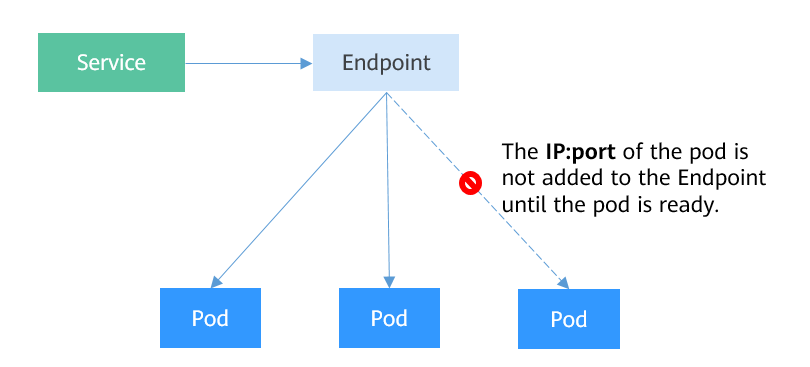
Exec
The Exec mode is the same as the HTTP GET mode. As shown below, the probe runs the ls /ready command. If the file exists, 0 is returned, indicating that the pod is ready. Otherwise, a non-zero status code is returned.
apiVersion: apps/v1 kind: Deployment metadata: name: nginx spec: replicas: 3 selector: matchLabels: app: nginx template: metadata: labels: app: nginx spec: containers: - image: nginx:alpine name: container-0 resources: limits: cpu: 100m memory: 200Mi requests: cpu: 100m memory: 200Mi readinessProbe: # Readiness Probe exec: # Define the ls /ready command. command: - ls - /ready imagePullSecrets: - name: default-secret
Save the definition of the Deployment to the deploy-read.yaml file, delete the previously created Deployment, and use the deploy-read.yaml file to recreate the Deployment.
# kubectl delete deploy nginx deployment.apps "nginx" deleted # kubectl create -f deploy-read.yaml deployment.apps/nginx created
The nginx image does not contain the /ready file. Therefore, the container is not in the Ready status after the creation, as shown below. Note that the values in the READY column are 0/1, indicating that the containers are not ready.
# kubectl get po NAME READY STATUS RESTARTS AGE nginx-7955fd7786-686hp 0/1 Running 0 7s nginx-7955fd7786-9tgwq 0/1 Running 0 7s nginx-7955fd7786-bqsbj 0/1 Running 0 7s
Create a Service.
apiVersion: v1 kind: Service metadata: name: nginx spec: selector: app: nginx ports: - name: service0 targetPort: 80 port: 8080 protocol: TCP type: ClusterIP
Check the Service. If there are no values in the Endpoints line, no Endpoints are found.
$ kubectl describe svc nginx Name: nginx ...... Endpoints: ......
If a /ready file is created in the container to make the readiness probe succeed, the container is in the Ready status. Check the pod and endpoints. It is found that the container for which the /ready file is created is ready and an endpoint is added.
# kubectl exec nginx-7955fd7786-686hp -- touch /ready # kubectl get po -o wide NAME READY STATUS RESTARTS AGE IP nginx-7955fd7786-686hp 1/1 Running 0 10m 192.168.93.169 nginx-7955fd7786-9tgwq 0/1 Running 0 10m 192.168.166.130 nginx-7955fd7786-bqsbj 0/1 Running 0 10m 192.168.252.160 # kubectl get endpoints NAME ENDPOINTS AGE nginx 192.168.93.169:80 14d
HTTP GET
The configuration of a readiness probe is the same as that of a livness probe, which is also in the containers field of the pod description template. As shown below, the readiness probe sends an HTTP request to the pod. If the probe receives 2xx or 3xx, the pod is ready.
apiVersion: apps/v1 kind: Deployment metadata: name: nginx spec: replicas: 3 selector: matchLabels: app: nginx template: metadata: labels: app: nginx spec: containers: - image: nginx:alpine name: container-0 resources: limits: cpu: 100m memory: 200Mi requests: cpu: 100m memory: 200Mi readinessProbe: # readinessProbe httpGet: # HTTP GET definition path: /read port: 80 imagePullSecrets: - name: default-secret
TCP Socket
The following example shows how to define a TCP Socket-type probe.
apiVersion: apps/v1 kind: Deployment metadata: name: nginx spec: replicas: 3 selector: matchLabels: app: nginx template: metadata: labels: app: nginx spec: containers: - image: nginx:alpine name: container-0 resources: limits: cpu: 100m memory: 200Mi requests: cpu: 100m memory: 200Mi readinessProbe: # readinessProbe tcpSocket: # TCP Socket definition port: 80 imagePullSecrets: - name: default-secret
Advanced Settings of a Readiness Probe
Similar to a liveness probe, a readiness probe also has the same advanced configuration items. The output of the describe command of the nginx pod is as follows:
Readiness: exec [ls /var/ready] delay=0s timeout=1s period=10s #success=1 #failure=3
This is the detailed configuration information of the readiness probe.
- delay=0s indicates that the probe starts immediately after the container is started.
- timeout=1s indicates that the container must respond to the probe within 1s. Otherwise, it is considered as a failure.
- period=10s indicates that the probe is performed every 10s.
- #success=1 indicates that the probe is considered successful as long as the probe succeeds once.
- #failure=3 indicates that the probe is considered failed if it fails for three consecutive times.
These are the default configurations when the probe is created. You can customize them as follows:
readinessProbe: # Readiness Probe exec: # Define the ls /readiness/ready command command: - ls - /readiness/ready initialDelaySeconds: 10 # Readiness probes are initiated after the container has started for 10s. timeoutSeconds: 2 # The container must respond within 2s. Otherwise, it is considered as a failure. periodSeconds: 30 # The probe is performed every 30s. successThreshold: 1 # The container is considered ready as long as the probe succeeds once. failureThreshold: 3 # The probe is considered to be failed after three consecutive failures.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot