RBAC
RBAC Resources
In Kubernetes, the RBAC mechanism is used for authorization. RBAC authorization uses four types of resources for configuration.
- Role: defines a set of rules for accessing Kubernetes resources in a namespace.
- RoleBinding: defines the relationship between users and roles.
- ClusterRole: defines a set of rules for accessing Kubernetes resources in a cluster (including all namespaces).
- ClusterRoleBinding: defines the relationship between users and cluster roles.
Role and ClusterRole specify actions that can be performed on specific resources. RoleBinding and ClusterRoleBinding bind roles to specific users, user groups, or ServiceAccounts. See the following figure.
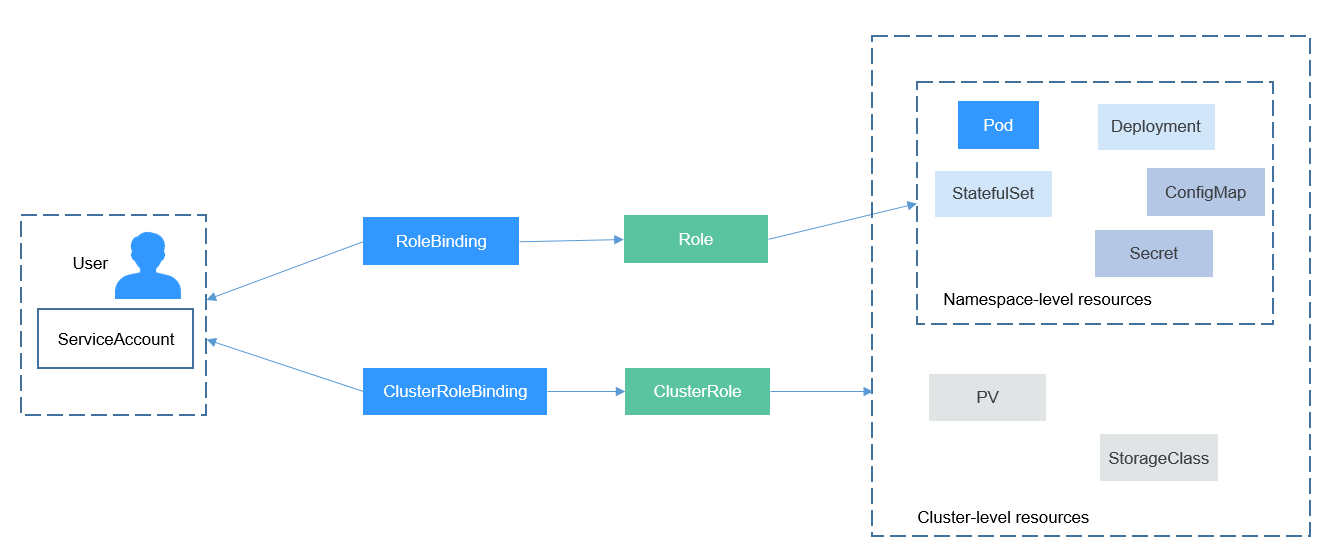
Creating a Role
The procedure for creating a Role is very simple. To be specific, specify a namespace and then define rules. The rules in the following example are to allow GET and LIST operations on pods in the default namespace.
kind: Role apiVersion: rbac.authorization.k8s.io/v1 metadata: namespace: default # Namespace name: role-example rules: - apiGroups: [""] resources: ["pods"] # The pod can be accessed. verbs: ["get", "list"] # The GET and LIST operations can be performed.
Creating a RoleBinding
After creating a Role, you can bind the Role to a specific user, which is called RoleBinding. The following is an example.
kind: RoleBinding apiVersion: rbac.authorization.k8s.io/v1 metadata: name: rolebinding-example namespace: default subjects: # Specified user - kind: User # Common user name: user-example apiGroup: rbac.authorization.k8s.io - kind: ServiceAccount # ServiceAccount name: sa-example namespace: default roleRef: # Specified Role kind: Role name: role-example apiGroup: rbac.authorization.k8s.io
The subjects is used to bind the Role to a user. The user can be an external common user or a ServiceAccount. For details about the two user types, see ServiceAccounts. The following figure shows the binding relationship.
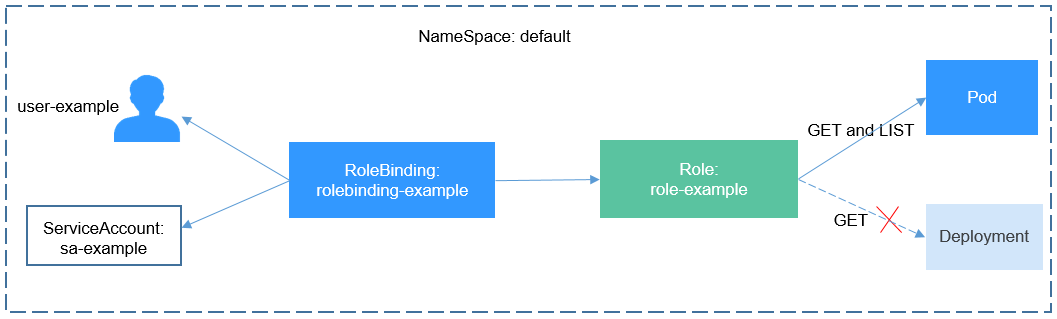
Then check whether the authorization takes effect.
In Using a ServiceAccount, a pod is created and the ServiceAccount sa-example is used. The Role role-example is bound to sa-example. Access the pod and run the curl command to access resources through the API Server to check whether the permission takes effect.
Use ca.crt and token corresponding to sa-example for authentication and query all pod resources (LIST in Creating a Role) in the default namespace.
$ kubectl exec -it sa-example -- /bin/sh # export CURL_CA_BUNDLE=/var/run/secrets/kubernetes.io/serviceaccount/ca.crt # TOKEN=$(cat /var/run/secrets/kubernetes.io/serviceaccount/token) # curl -H "Authorization: Bearer $TOKEN" https://kubernetes/api/v1/namespaces/default/pods { "kind": "PodList", "apiVersion": "v1", "metadata": { "selfLink": "/api/v1/namespaces/default/pods", "resourceVersion": "10377013" }, "items": [ { "metadata": { "name": "sa-example", "namespace": "default", "selfLink": "/api/v1/namespaces/default/pods/sa-example", "uid": "c969fb72-ad72-4111-a9f1-0a8b148e4a3f", "resourceVersion": "10362903", "creationTimestamp": "2020-07-15T06:19:26Z" }, "spec": { ...
If the returned result is normal, sa-example has permission to list pods. Query the Deployment again. If the following information is displayed, you do not have the permission to access the Deployment.
# curl -H "Authorization: Bearer $TOKEN" https://kubernetes/api/v1/namespaces/default/deployments ... "status": "Failure", "message": "deployments is forbidden: User \"system:serviceaccount:default:sa-example\" cannot list resource \"deployments\" in API group \"\" in the namespace \"default\"", ...
Role and RoleBinding apply to namespaces and can isolate permissions to some extent. As shown in the following figure, role-example defined above cannot access resources in the kube-system namespace.
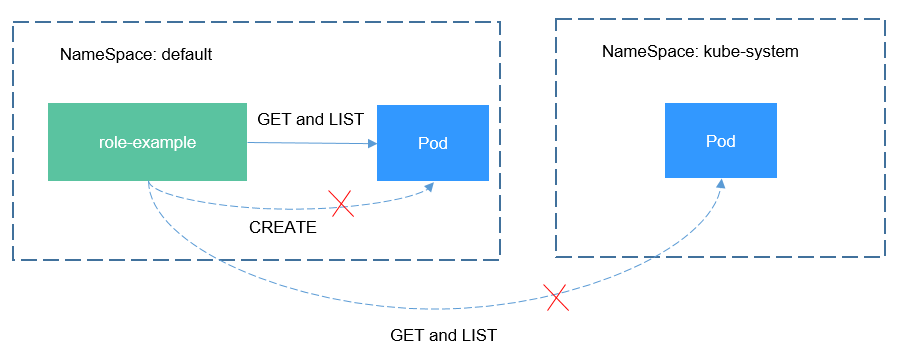
Continue to access the pod. If the following information is displayed, you do not have the permission.
# curl -H "Authorization: Bearer $TOKEN" https://kubernetes/api/v1/namespaces/kube-system/pods ... "status": "Failure", "message": "pods is forbidden: User \"system:serviceaccount:default:sa-example\" cannot list resource \"pods\" in API group \"\" in the namespace \"kube-system\"", "reason": "Forbidden", ...
In RoleBinding, you can also bind the ServiceAccounts of other namespaces by adding them under the subjects field.
subjects: # Specified user - kind: ServiceAccount # ServiceAccount name: kube-sa-example namespace: kube-system
Then the ServiceAccount kube-sa-example in kube-system can perform GET and LIST operations on pods in the default namespace, as shown in the following figure.
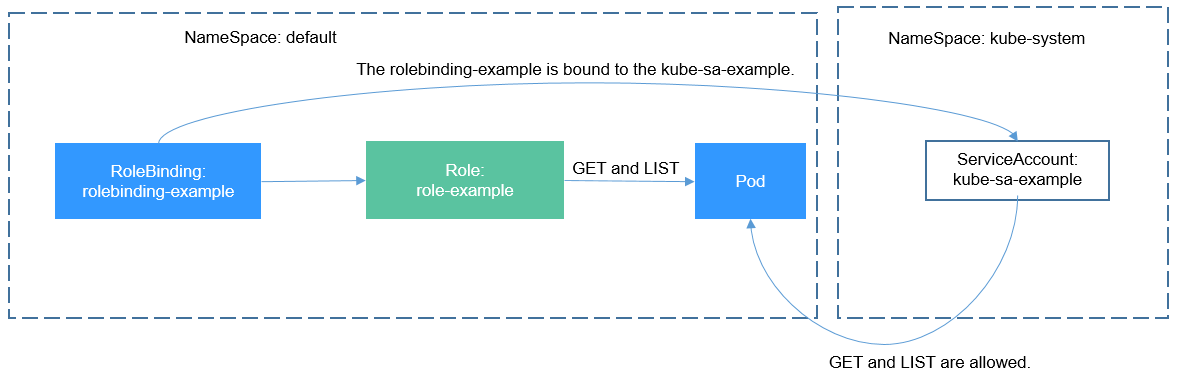
ClusterRole and ClusterRoleBinding
Compared with Role and RoleBinding, ClusterRole and ClusterRoleBinding have the following differences:
- ClusterRole and ClusterRoleBinding do not need to define the namespace field.
- ClusterRole can define cluster-level resources.
You can see that ClusterRole and ClusterRoleBinding control cluster-level permissions.
In Kubernetes, many ClusterRoles and ClusterRoleBindings are defined by default.
$ kubectl get clusterroles NAME AGE admin 30d cceaddon-prometheus-kube-state-metrics 6d3h cluster-admin 30d coredns 30d custom-metrics-resource-reader 6d3h custom-metrics-server-resources 6d3h edit 30d prometheus 6d3h system:aggregate-customedhorizontalpodautoscalers-admin 6d2h system:aggregate-customedhorizontalpodautoscalers-edit 6d2h system:aggregate-customedhorizontalpodautoscalers-view 6d2h .... view 30d $ kubectl get clusterrolebindings NAME AGE authenticated-access-network 30d authenticated-packageversion 30d auto-approve-csrs-for-group 30d auto-approve-renewals-for-nodes 30d auto-approve-renewals-for-nodes-server 30d cceaddon-prometheus-kube-state-metrics 6d3h cluster-admin 30d cluster-creator 30d coredns 30d csrs-for-bootstrapping 30d system:basic-user 30d system:ccehpa-rolebinding 6d2h system:cluster-autoscaler 6d1h ...
The most important and commonly used ClusterRoles are as follows:
- view: has the permission to view namespace resources.
- edit: has the permission to modify namespace resources.
- admin: has all permissions on the namespace.
- cluster-admin: has all permissions on the cluster.
Run the kubectl describe clusterrole command to view the permissions of each rule.
Generally, the four ClusterRoles are bound to users to isolate permissions. Note that Roles (rules and permissions) are separated from users. You can flexibly control permissions by combining the two through RoleBinding.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot