Deployment
Deployment
A pod is the smallest and simplest unit that you create or deploy in Kubernetes. It is designed to be an ephemeral, one-off entity. A pod can be evicted when node resources are insufficient and disappears along with a cluster node failure. Kubernetes provides controllers to manage pods. Controllers can create and manage pods, and provide replica management, rolling upgrade, and self-healing capabilities. The most commonly used controller is Deployment.
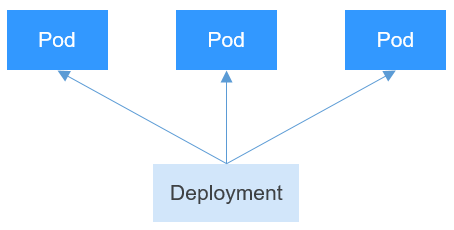
A Deployment can contain one or more pods. These pods have the same role. Therefore, the system automatically distributes requests to multiple pods of a Deployment.
A Deployment integrates a lot of functions, including online deployment, rolling upgrade, replica creation, and restoration of online jobs. To some extent, Deployments can be used to realize unattended rollout, which greatly reduces difficulties and operation risks in the rollout process.
Creating a Deployment
In the following example, a Deployment named nginx is created, and two pods are created from the nginx:latest image. Each pod occupies 100m CPU and 200 MiB memory.
apiVersion: apps/v1 # Note the difference with a pod. It is apps/v1 instead of v1 for a Deployment. kind: Deployment # The resource type is Deployment. metadata: name: nginx # Name of the Deployment spec: replicas: 2 # Number of pods. The Deployment ensures that two pods are running. selector: # Label Selector matchLabels: app: nginx template: # Definition of a pod, which is used to create pods. It is also known as pod template. metadata: labels: app: nginx spec: containers: - image: nginx:latest name: container-0 resources: limits cpu: 100m memory: 200Mi requests: cpu: 100m memory: 200Mi imagePullSecrets: - name: default-secret
In this definition, the name of the Deployment is nginx, and spec.replicas defines the number of pods. That is, the Deployment controls two pods. spec.selector is a label selector, indicating that the Deployment selects the pod whose label is app=nginx. spec.template is the definition of the pod and is the same as that defined in Pods.
Save the definition of the Deployment to deployment.yaml and use kubectl to create the Deployment.
Run kubectl get to view the Deployment and pods. In the following example, the value of READY is 2/2. The first 2 indicates that two pods are running, and the second 2 indicates that two pods are expected in this Deployment. The value 2 of AVAILABLE indicates that two pods are available.
$ kubectl create -f deployment.yaml deployment.apps/nginx created $ kubectl get deploy NAME READY UP-TO-DATE AVAILABLE AGE nginx 2/2 2 2 4m5s
How Does the Deployment Control Pods?
Continue to query pods, as shown below.
$kubectl get pods NAME READY STATUS RESTARTS AGE nginx-7f98958cdf-tdmqk 1/1 Running 0 13s nginx-7f98958cdf-txckx 1/1 Running 0 13s
If you delete a pod, a new pod is immediately created, as shown below. As mentioned above, the Deployment ensures that there are two pods running. If a pod is deleted, the Deployment creates a new pod. If a pod becomes faulty, the Deployment automatically restarts the pod.
$ kubectl get pods NAME READY STATUS RESTARTS AGE nginx-7f98958cdf-tdmqk 1/1 Running 0 21s nginx-7f98958cdf-tesqr 1/1 Running 0 1s
You see two pods, nginx-7f98958cdf-tdmqk and nginx-7f98958cdf-tesqr. nginx is the name of the Deployment. -7f98958cdf-tdmqk and -7f98958cdf-tesqr are the suffixes randomly generated by Kubernetes.
You may notice that the two suffixes share the same content 7f98958cdf in the first part. This is because the Deployment does not control the pods directly, but through a controller named ReplicaSet. You can run the following command to query the ReplicaSet. In the command, rs is the abbreviation of ReplicaSet.
$ kubectl get rs NAME DESIRED CURRENT READY AGE nginx-7f98958cdf 2 2 2 1m
The ReplicaSet is named nginx-7f98958cdf, in which the suffix -7f98958cdf is generated randomly.
As shown in Figure 2, the Deployment controls the ReplicaSet, which then controls pods.
If you run the kubectl describe command to view the details of the Deployment, you can see the ReplicaSet (NewReplicaSet: nginx-7f98958cdf (2/2 replicas created)). In Events, the number of pods of the ReplicaSet is scaled out to 2. In practice, you may not operate ReplicaSet directly, but understanding that a Deployment controls a pod by controlling a ReplicaSet helps you locate problems.
$ kubectl describe deploy nginx Name: nginx Namespace: default CreationTimestamp: Sun, 16 Dec 2018 19:21:58 +0800 Labels: app=nginx ... NewReplicaSet: nginx-7f98958cdf (2/2 replicas created) Events: Type Reason Age From Message ---- ------ ---- ---- ------- Normal ScalingReplicaSet 5m deployment-controller Scaled up replica set nginx-7f98958cdf to 2
Upgrade
In actual applications, upgrade is a common operation. A Deployment can easily support application upgrade.
You can set different upgrade policies for a Deployment:
- RollingUpdate: New pods are created gradually and then old pods are deleted. This is the default policy.
- Recreate: The current pods are deleted and then new pods are created.
The Deployment can be upgraded in a declarative mode. That is, you only need to modify the YAML definition of the Deployment. For example, you can run the kubectl edit command to change the Deployment image to nginx:alpine. After the modification, query the ReplicaSet and pod. The query result shows that a new ReplicaSet is created and the pod is re-created.
$ kubectl edit deploy nginx $ kubectl get rs NAME DESIRED CURRENT READY AGE nginx-6f9f58dffd 2 2 2 1m nginx-7f98958cdf 0 0 0 48m $ kubectl get pods NAME READY STATUS RESTARTS AGE nginx-6f9f58dffd-tdmqk 1/1 Running 0 1m nginx-6f9f58dffd-tesqr 1/1 Running 0 1m
The Deployment can use the maxSurge and maxUnavailable parameters to control the proportion of pods to be re-created during the upgrade, which is useful in many scenarios. The configuration is as follows:
spec: strategy: rollingUpdate: maxSurge: 1 maxUnavailable: 0 type: RollingUpdate
- maxSurge specifies the maximum number of pods that can exist over spec.replicas in the Deployment. The default value is 25%. For example, if spec.replicas is set to 4, no more than 5 pods can exist during the upgrade process, that is, the upgrade step is 1. The absolute number is calculated from the percentage by rounding up. The value can also be set to an absolute number.
- maxUnavailable: specifies the maximum number of pods that can be unavailable during the update process. The default value is 25%. For example, if spec.replicas is set to 4, at least 3 pods exist during the upgrade process, that is, the deletion step is 1. The value can also be set to an absolute number.
In the preceding example, the value of spec.replicas is 2. If both maxSurge and maxUnavailable are the default value 25%, maxSurge allows a maximum of three pods to exist (2 x 1.25 = 2.5, rounded up to 3), and maxUnavailable does not allow a maximum of two pods to be unavailable (2 x 0.75 = 1.5, rounded up to 2). That is, during the upgrade process, there will always be two pods running. Each time a new pod is created, an old pod is deleted, until all pods are new.
Rollback
Rollback is to roll an application back to the earlier version when a fault occurs during the upgrade. A Deployment can be easily rolled back to the earlier version.
For example, if the upgraded image is faulty, you can run the kubectl rollout undo command to roll back the Deployment.
$ kubectl rollout undo deployment nginx deployment.apps/nginx rolled back
A Deployment can be easily rolled back because it uses a ReplicaSet to control a pod. After the upgrade, the previous ReplicaSet still exists. The Deployment is rolled back by using the previous ReplicaSet to re-create the pod. The number of ReplicaSets stored in a Deployment can be restricted by the revisionHistoryLimit parameter. The default value is 10.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot