Bucket Quota
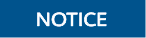
If you have any questions during development, post them on the Issues page of GitHub.
Setting a Bucket Quota
You can use the set_bucket_quota function to set the bucket quota. The following table describes the parameters.
Field |
Type |
Mandatory or Optional |
Description |
---|---|---|---|
option |
The context of the bucket. For details, see Configuring option. |
Mandatory |
Bucket parameter |
storage_quota |
uint64_t |
Mandatory |
Quota size, in bytes. |
handler |
obs_response_handler * |
Mandatory |
Callback function |
callback_data |
void * |
Optional |
Callback data |
static void test_set_bucket_quota( char *bucket_name) { obs_status ret_status = OBS_STATUS_BUTT; uint64_t bucketquota = 104857600; // Create and initialize option. obs_options option; init_obs_options(&option); option.bucket_options.host_name = "<your-endpoint>"; option.bucket_options.bucket_name = "<Your bucketname>"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. option.bucket_options.access_key = getenv("ACCESS_KEY_ID"); option.bucket_options.secret_access_key = getenv("SECRET_ACCESS_KEY"); // Set response callback function. obs_response_handler response_handler = { 0, &response_complete_callback }; // Set bucket quota. set_bucket_quota(&option, bucketquota, &response_handler, &ret_status); if (OBS_STATUS_OK == ret_status) { printf("set bucket quota successfully. \n"); } else { printf("set bucket quota failed(%s).\n", obs_get_status_name(ret_status)); } }

A bucket quota must be a non-negative integer expressed in bytes. The maximum value is 263 - 1.
Obtaining a Bucket Quota
You can use the get_bucket_quot function to obtain bucket quotas. The following table describes the parameters.
Field |
Type |
Mandatory or Optional |
Description |
---|---|---|---|
option |
The context of the bucket. For details, see Configuring option. |
Mandatory |
Bucket parameter |
storagequota_return |
uint64_t * |
Mandatory |
The obtained quota size, in bytes. |
handler |
obs_response_handler * |
Mandatory |
Callback function |
callback_data |
void * |
Optional |
Callback data |
Sample code:
static void test_get_bucket_quota( char *bucket_name) { obs_status ret_status = OBS_STATUS_BUTT; // Create and initialize option. obs_options option; init_obs_options(&option); option.bucket_options.host_name = "<your-endpoint>"; option.bucket_options.bucket_name = "<Your bucketname>"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. option.bucket_options.access_key = getenv("ACCESS_KEY_ID"); option.bucket_options.secret_access_key = getenv("SECRET_ACCESS_KEY"); // Set response callback function. obs_response_handler response_handler = { 0, &response_complete_callback }; // Obtain the bucket quota. uint64_t bucketquota = 0; get_bucket_quota(&option, &bucketquota, &response_handler, &ret_status); if (OBS_STATUS_OK == ret_status) { printf("Bucket=%s Quota=%lu \n get bucket quota successfully. \n ", bucket_name, bucketquota); } else { printf("get bucket quota failed(%s).\n", obs_get_status_name(ret_status)); } }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot