Log Initialization (SDK for Python)
Function
You can enable the SDK log function to record log information generated during API calling into log files for subsequent data analysis or fault location. The procedure is as follows:
- Find file log.conf in the OBS Python SDK (obtained from GitHub). The content format is as follows:
[LOGCONF] #Configure log file dir LogFileDir = ./logs #Configure log file name LogFileName = eSDK-OBS-PYTHON.log #Configure log file size, unit:MB LogFileSize = 30 #Configure max log file numbers LogFileNumber = 5 #Configure log level for log file (DEBUG | INFO | WARNING | ERROR) LogFileLevel = INFO #Configure whether to print log to console (Yes:1 No:0) PrintLogToConsole = 0 #Configure log level for console (DEBUG | INFO | WARNING | ERROR) PrintLogLevel = WARNING
- Modify parameters in the log.conf file as needed.
- Call ObsClient.initLog to enable the logging function.
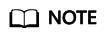
- The logging function is disabled by default. You need to enable it manually.
- For details about SDK logs, see Log Analysis.
- You can change the log file permissions in the system based on your actual needs.
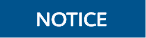
The log module of the OBS Python SDK is thread secure but not process secure. If ObsClient is used in multi-process scenarios, you must configure an independent log path for each instance of ObsClient to prevent conflicts when multiple processes write logs concurrently.
Method
obsClient.initLog( log_config='*** Your Log Configuration Parameters ***', log_name='*** Your Log Name ***' )
Constructor Parameter Description
Parameter |
Type |
Mandatory (Yes/No) |
Description |
---|---|---|---|
log_config |
Yes |
Log configuration parameter |
|
log_name |
str |
No |
Log name |
Code Examples
# Import the module. from obs import ObsClient # Obtain an AK and SK pair using environment variables or import the AK and SK pair in other ways. Using hard coding may result in leakage. # Obtain an AK and SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. ak = os.getenv("AccessKeyID") sk = os.getenv("SecretAccessKey") # (Optional) If you use a temporary AK and SK pair and a security token to access OBS, obtain them from environment variables. security_token = os.getenv("SecurityToken") # Set server to the endpoint corresponding to the bucket. CN-Hong Kong is used here as an example. Replace it with the one currently in use. server = "https://obs.ap-southeast-1.myhuaweicloud.com" # Create an obsClient instance. # If you use a temporary AK and SK pair and a security token to access OBS, you must specify security_token when creating an instance. obsClient = ObsClient(access_key_id=ak, secret_access_key=sk, server=server) # Import the log module. from obs import LogConf # Specify the path to the log configuration file and initialize logs of ObsClient. obsClient.initLog(LogConf('./log.conf'), '*** Your Log Name ***') # Use ObsClient to access OBS. # Disable ObsClient logging. obsClient.close()
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot