Managing Object ACLs

If you have any questions during the development, post them on the Issues page of GitHub. For details about parameters and usage of each API, see the API Reference.
Object ACLs, similar to bucket ACLs, support pre-defined access control policies and direct configuration. For details, see Managing Bucket ACLs.
An object ACL can be configured in any of the following ways:
- Specify a pre-defined access control policy during object upload.
- Call ObsClient.SetObjectAcl to specify a pre-defined access control policy.
- Call ObsClient.SetObjectAcl to set the ACL directly.
Specifying a Pre-defined Access Control Policy During Object Upload
Sample code:
// Initialize configuration parameters. ObsConfig config = new ObsConfig(); config.Endpoint = "https://your-endpoint"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables AccessKeyID and SecretAccessKey. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. string accessKey= Environment.GetEnvironmentVariable("AccessKeyID", EnvironmentVariableTarget.Machine); string secretKey= Environment.GetEnvironmentVariable("SecretAccessKey", EnvironmentVariableTarget.Machine); // Create an instance of ObsClient. ObsClient client = new ObsClient(accessKey, secretKey, config); // Specify a pre-defined access control policy for the to-be-uploaded object. try { PutObjectRequest request = new PutObjectRequest { BucketName = "bucketname", ObjectKey = "objectname", // Set the ACL to public-read-write. CannedAcl = CannedAclEnum.PublicReadWrite, }; PutObjectResponse response = client.PutObject(request); Console.WriteLine("Set object ac response: {0}", response.StatusCode); } catch (ObsException ex) { Console.WriteLine("ErrorCode: {0}", ex.ErrorCode); Console.WriteLine("ErrorMessage: {0}", ex.ErrorMessage); }
Setting a Pre-defined Access Control Policy for the Object
Sample code:
// Initialize configuration parameters. ObsConfig config = new ObsConfig(); config.Endpoint = "https://your-endpoint"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables AccessKeyID and SecretAccessKey. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. string accessKey= Environment.GetEnvironmentVariable("AccessKeyID", EnvironmentVariableTarget.Machine); string secretKey= Environment.GetEnvironmentVariable("SecretAccessKey", EnvironmentVariableTarget.Machine); // Create an instance of ObsClient. ObsClient client = new ObsClient(accessKey, secretKey, config); // Set a pre-defined access control policy for the object. try { SetObjectAclRequest request = new SetObjectAclRequest(); request.BucketName = "bucketname"; request.ObjectKey = "objectname"; request.CannedAcl = CannedAclEnum.PublicRead; SetObjectAclResponse response = client.SetObjectAcl(request); Console.WriteLine("Set object acl response: {0}", response.StatusCode); } catch (ObsException ex) { Console.WriteLine("ErrorCode: {0}", ex.ErrorCode); Console.WriteLine("ErrorMessage: {0}", ex.ErrorMessage); }
Directly Setting the Object ACL
Sample code:
// Initialize configuration parameters. ObsConfig config = new ObsConfig(); config.Endpoint = "https://your-endpoint"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables AccessKeyID and SecretAccessKey. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. string accessKey= Environment.GetEnvironmentVariable("AccessKeyID", EnvironmentVariableTarget.Machine); string secretKey= Environment.GetEnvironmentVariable("SecretAccessKey", EnvironmentVariableTarget.Machine); // Create an instance of ObsClient. ObsClient client = new ObsClient(accessKey, secretKey, config); // Set the object ACL directly. try { SetObjectAclRequest request = new SetObjectAclRequest(); request.BucketName = "bucketname"; request.ObjectKey = "objectname"; request.AccessControlList = new AccessControlList(); Owner owner = new Owner(); owner.Id = "owerid"; request.AccessControlList.Owner = owner; Grant item = new Grant(); item.Permission = PermissionEnum.FullControl; item.Grantee = new GroupGrantee(GroupGranteeEnum.AllUsers); request.AccessControlList.Grants.Add(item); SetObjectAclResponse response = client.SetObjectAcl(request); Console.WriteLine("Set object acl response: {0}", response.StatusCode); } catch (ObsException ex) { Console.WriteLine("ErrorCode: {0}", ex.ErrorCode); Console.WriteLine("ErrorMessage: {0}", ex.ErrorMessage); }
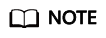
The owner or grantee ID needed in the ACL indicates the account ID, which can be viewed on the My Credentials page of OBS Console.
Obtaining an Object ACL
// Initialize configuration parameters. ObsConfig config = new ObsConfig(); config.Endpoint = "https://your-endpoint"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables AccessKeyID and SecretAccessKey. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. string accessKey= Environment.GetEnvironmentVariable("AccessKeyID", EnvironmentVariableTarget.Machine); string secretKey= Environment.GetEnvironmentVariable("SecretAccessKey", EnvironmentVariableTarget.Machine); // Create an instance of ObsClient. ObsClient client = new ObsClient(accessKey, secretKey, config); // Obtain the object ACL. try { GetObjectAclRequest request = new GetObjectAclRequest(); request.BucketName = "bucketname"; request.ObjectKey = "objectname"; GetObjectAclResponse response = client.GetObjectAcl(request); Console.WriteLine("Get bucket acl response: {0}", response.StatusCode); foreach(Grant grant in response.AccessControlList.Grants) { if(grant.Grantee is CanonicalGrantee) { Console.WriteLine("Grantee id: {0}", (grant.Grantee as CanonicalGrantee).Id); }else if(grant.Grantee is GroupGrantee) { Console.WriteLine("Grantee type: {0}", (grant.Grantee as GroupGrantee).GroupGranteeType); } Console.WriteLine("Grant permission: {0}", grant.Permission); } } catch (ObsException ex) { Console.WriteLine("ErrorCode: {0}", ex.ErrorCode); Console.WriteLine("ErrorMessage: {0}", ex.ErrorMessage); }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot