Performing an Appendable Upload
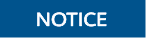
If you have any questions during development, post them on the Issues page of GitHub. For details about parameters and usage of each API, see the API Reference.
Appendable upload allows you to upload an object in appendable mode and then append data to the object. You can call ObsClient.appendObject to perform an appendable upload. Sample code is as follows:
// Create an instance of ObsClient. var obsClient = new ObsClient({ // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables AccessKeyID and SecretAccessKey. // The front-end code does not have the process environment variable, so you need to use a module bundler like webpack to define the process variable. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. access_key_id: process.env.AccessKeyID, secret_access_key: process.env.SecretAccessKey, // Replace the example endpoint with the actual one in your case. server: 'https://obs.ap-southeast-1.myhuaweicloud.com' }); // Create an appendable object. The start position must be 0. obsClient.appendObject({ Bucket:'bucketname', Key:'objectname', Position : 0, Body : 'Hello OBS' }).then(function(result){ console.log('Status-->' + result.CommonMsg.Status); if(result.CommonMsg.Status < 300 && result.InterfaceResult){ console.log('NextPosition-->' + result.InterfaceResult.NextPosition); } // Append data to the appendable object. obsClient.appendObject({ Bucket:'bucketname', Key:'objectname', Position : result.InterfaceResult.NextPosition, Body : 'Hello OBS Again' }, function(err, result2){ if(err){ console.error('Error-->' + err); }else{ console.log('Status-->' + result2.CommonMsg.Status); if(result2.CommonMsg.Status < 300 && result2.InterfaceResult){ console.log('NextPosition-->' + result2.InterfaceResult.NextPosition); } } }); // Use the API for obtaining object properties to get the start position for next appending. obsClient.getObjectMetadata({ Bucket:'bucketname', Key:'objectname', }).then(function(result3){ console.log('Status-->' + result3.CommonMsg.Status); if(result3.CommonMsg.Status < 300 && result3.InterfaceResult){ console.log('RequestId-->' + result3.InterfaceResult.RequestId); console.log('NextPosition-->' + result3.InterfaceResult.NextPosition); } }).catch(function(err){ console.error('err:' + err); }); }).catch(function(err){ console.error('err:' + err); });
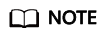
- Use the Position parameter to specify the start position for next appending and set it to 0 when you create an appendable object.
- Objects uploaded using ObsClient.putObject, referred to as normal objects, can overwrite objects uploaded using ObsClient.appendObject, referred to as appendable objects. Data cannot be appended to an appendable object anymore once the object has been overwritten by a normal object.
- When you upload an object for the first time in appendable mode, an exception will be thrown (status code 409) if a normal object with the same name exists.
- The ETag returned for an appendable upload is the ETag for the uploaded content, rather than that of the whole object.
- Data appended each time can be up to 5 GB, and 10000 times of appendable uploads can be performed on a single object.
- After an appendable upload is complete successfully, you can use InterfaceResult.NextPosition obtained from the returned result or call ObsClient.getObjectMetadata, to get the location for next appending.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot