Configuring Lifecycle Management
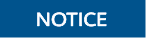
If you have any questions during development, post them on the Issues page of GitHub. For details about parameters and usage of each API, see the API Reference.
When uploading an object or initializing a multipart upload, you can directly set the expiration time for the object. Sample code is as follows:
// Create an instance of ObsClient. var obsClient = new ObsClient({ // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables AccessKeyID and SecretAccessKey. // The front-end code does not have the process environment variable, so you need to use a module bundler like webpack to define the process variable. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. access_key_id: process.env.AccessKeyID, secret_access_key: process.env.SecretAccessKey, // Replace the example endpoint with the actual one in your case. server: 'https://obs.ap-southeast-1.myhuaweicloud.com' }); // When uploading an object, set the object to expire after 30 days. obsClient.putObject({ Bucket : 'bucketname', Key : 'objectname', Body : 'Hello OBS', Expires : 30 }, function(err, result){ if(err){ console.error('Error-->' + err); }else{ console.log('Status-->' + result.CommonMsg.Status); } }); // When initializing a multipart upload, set the object to expire 60 days after combination. obsClient.initiateMultipartUpload({ Bucket : 'bucketname', Key : 'objectname', ContentType : 'text/plain', Expires : 60 }, function(err, result) { if(err){ console.error('Error-->' + err); }else{ console.log('Status-->' + result.CommonMsg.Status); if(result.CommonMsg.Status < 300 && result.InterfaceResult){ console.log('UploadId-->' + result.InterfaceResult.UploadId); } } });
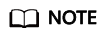
- Use the Expires parameter to specify expiration time for the object.
- The previous mode specifies the time duration in days after which an object will expire. The OBS server automatically clears expired objects.
- The object expiration time set in the preceding method takes precedence over the bucket lifecycle rule.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot