Setting or Obtaining a Versioning Object ACL

If you have any questions during the development, post them on the Issues page of GitHub. For details about parameters and usage of each API, see the API Reference.
Directly Setting a Versioning Object ACL
You can call ObsClient.SetObjectAcl to pass the version ID (VersionId) to set the ACL for a versioning object. Sample code is as follows:
// Initialize configuration parameters. ObsConfig config = new ObsConfig(); config.Endpoint = "https://your-endpoint"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables AccessKeyID and SecretAccessKey. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. string accessKey= Environment.GetEnvironmentVariable("AccessKeyID", EnvironmentVariableTarget.Machine); string secretKey= Environment.GetEnvironmentVariable("SecretAccessKey", EnvironmentVariableTarget.Machine); // Create an instance of ObsClient. ObsClient client = new ObsClient(accessKey, secretKey, config); // Set the ACL for a versioning object. try { SetObjectAclRequest request = new SetObjectAclRequest(); request.BucketName = "bucketname"; request.ObjectKey = "objectname"; request.VersionId = "versionId"; request.AccessControlList = new AccessControlList(); Owner owner = new Owner(); owner.Id = "ownerid"; request.AccessControlList.Owner = owner; Grant item = new Grant(); item.Permission = PermissionEnum.FullControl; item.Grantee = new GroupGrantee(GroupGranteeEnum.AllUsers); request.AccessControlList.Grants.Add(item); SetObjectAclResponse response = client.SetObjectAcl(request); Console.WriteLine("Set object acl response: {0}", response.StatusCode); } catch (ObsException ex) { Console.WriteLine("ErrorCode: {0}", ex.ErrorCode); Console.WriteLine("ErrorMessage: {0}", ex.ErrorMessage); }
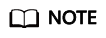
The owner or grantee ID needed in the ACL indicates the account ID, which can be viewed on the My Credentials page of OBS Console.
Obtaining a Versioning Object ACL
You can call ObsClient.GetObjectAcl to pass the version ID (VersionId) to obtain the ACL of a versioning object. Sample code is as follows:
// Initialize configuration parameters. ObsConfig config = new ObsConfig(); config.Endpoint = "https://your-endpoint"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables AccessKeyID and SecretAccessKey. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. string accessKey= Environment.GetEnvironmentVariable("AccessKeyID", EnvironmentVariableTarget.Machine); string secretKey= Environment.GetEnvironmentVariable("SecretAccessKey", EnvironmentVariableTarget.Machine); // Create an instance of ObsClient. ObsClient client = new ObsClient(accessKey, secretKey, config); // Obtain the ACL of a versioning object. try { GetObjectAclRequest request = new GetObjectAclRequest(); request.BucketName = "bucketname"; request.ObjectKey = "objectname"; request.VersionId = "versionId"; GetObjectAclResponse response = client.GetObjectAcl(request); Console.WriteLine("GetObjectAcl grant account: {0}", response.AccessControlList.Grants.Count); Console.WriteLine("GetObjectAcl owner id: {0}", response.AccessControlList.Owner.Id); foreach (Grant grant in response.AccessControlList.Grants) { if(grant.Grantee is CanonicalGrantee) { Console.WriteLine("Grantee id: {0}", (grant.Grantee as CanonicalGrantee).Id); }else if(grant.Grantee is GroupGrantee) { Console.WriteLine("Grantee type: {0}", (grant.Grantee as GroupGrantee).GroupGranteeType); } Console.WriteLine("Grant permission: {0}", grant.Permission); } } } catch (ObsException ex) { Console.WriteLine("ErrorCode: {0}", ex.ErrorCode); Console.WriteLine("ErrorMessage: {0}", ex.ErrorMessage); }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot