Renaming an Object or Directory
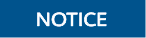
If you have any questions during development, post them on the Issues page of GitHub.
You can call rename_object to change the object name and directory name. This API applies only to buckets used as parallel file systems.
Parameter Description
Field |
Type |
Mandatory or Optional |
Description |
---|---|---|---|
option |
Request for the context of the bucket, see Configuring option |
Mandatory |
Bucket parameter |
key |
char * |
Mandatory |
Name of the object or directory to be renamed |
new_object_name |
char * |
Mandatory |
New name |
handler |
obs_response_handler * |
Mandatory |
Callback function |
callback_data |
void * |
Optional |
Callback data |
Sample Code
The following code shows how to rename an object:
static void test_rename_object() { obs_status ret_status = OBS_STATUS_BUTT; // Name of the object or directory to be renamed char *key = "put_buffer_test"; // New name char *new_key_name = "put_buffer_test_new"; // Create and initialize option. obs_options option; init_obs_options(&option); option.bucket_options.host_name = "<your-endpoint>"; option.bucket_options.bucket_name = "<Your bucketname>"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. option.bucket_options.access_key = getenv("ACCESS_KEY_ID"); option.bucket_options.secret_access_key = getenv("SECRET_ACCESS_KEY"); // Set response callback function. obs_response_handler response_handler = { &response_properties_callback, &response_complete_callback }; // Rename an object. rename_object(&option, key, new_key_name, &response_handler,&ret_status); if (OBS_STATUS_OK == ret_status) { printf("rename_object successfully. \n"); } else { printf("rename_object failed.\n", obs_get_status_name(ret_status)); } }

- rename_object applies only to buckets used as parallel file systems.
- If the object to be renamed does not exist, an error is reported with the HTTP status code 404.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot