Using a Temporary URL for Authorized Access

If you have any questions during development, post them on the Issues page of GitHub. For details about parameters and usage of each API, see the API Reference
ObsClient allows you to create a URL whose Query parameters are carried with authentication information by specifying the AK and SK, HTTP method, and request parameters. You can provide other users with this URL for temporary access. When generating a URL, you need to specify the validity period of the URL to restrict the access duration of visitors.
If you want to grant other users the permission to perform other operations on buckets or objects (for example, upload or download objects), generate a URL with the corresponding request (for example, to upload an object using the URL that generates the PUT request) and provide the URL for other users.
The following table lists operations can be performed through a signed URL.
Operation |
HTTP Request Method (Value in OBS Android SDK) |
Special Operator (Value in OBS Android SDK) |
Bucket Name Required |
Object Name Required |
---|---|---|---|---|
PUT Bucket |
HttpMethodEnum.PUT |
N/A |
Yes |
No |
GET Buckets |
HttpMethodEnum.GET |
N/A |
No |
No |
DELETE Bucket |
HttpMethodEnum.DELETE |
N/A |
Yes |
No |
GET Objects |
HttpMethodEnum.GET |
N/A |
Yes |
No |
GET Object versions |
HttpMethodEnum.GET |
SpecialParamEnum.VERSIONS |
Yes |
No |
List Multipart uploads |
HttpMethodEnum.GET |
SpecialParamEnum.UPLOADS |
Yes |
No |
Obtain Bucket Metadata |
HttpMethodEnum.HEAD |
N/A |
Yes |
No |
GET Bucket location |
HttpMethodEnum.GET |
SpecialParamEnum.LOCATION |
Yes |
No |
GET Bucket storageinfo |
HttpMethodEnum.GET |
SpecialParamEnum.STORAGEINFO |
Yes |
No |
PUT Bucket quota |
HttpMethodEnum.PUT |
SpecialParamEnum.QUOTA |
Yes |
No |
GET Bucket quota |
HttpMethodEnum.GET |
SpecialParamEnum.QUOTA |
Yes |
No |
Set Bucket storagePolicy |
HttpMethodEnum.PUT |
SpecialParamEnum.STORAGEPOLICY |
Yes |
No |
GET Bucket storagePolicy |
HttpMethodEnum.GET |
SpecialParamEnum.STORAGEPOLICY |
Yes |
No |
PUT Bucket acl |
HttpMethodEnum.PUT |
SpecialParamEnum.ACL |
Yes |
No |
GET Bucket acl |
HttpMethodEnum.GET |
SpecialParamEnum.ACL |
Yes |
No |
PUT Bucket logging |
HttpMethodEnum.PUT |
SpecialParamEnum.LOGGING |
Yes |
No |
GET Bucket logging |
HttpMethodEnum.GET |
SpecialParamEnum.LOGGING |
Yes |
No |
PUT Bucket policy |
HttpMethodEnum.PUT |
SpecialParamEnum.POLICY |
Yes |
No |
GET Bucket policy |
HttpMethodEnum.GET |
SpecialParamEnum.POLICY |
Yes |
No |
DELETE Bucket policy |
HttpMethodEnum.DELETE |
SpecialParamEnum.POLICY |
Yes |
No |
PUT Bucket lifecycle |
HttpMethodEnum.PUT |
SpecialParamEnum.LIFECYCLE |
Yes |
No |
GET Bucket lifecycle |
HttpMethodEnum.GET |
SpecialParamEnum.LIFECYCLE |
Yes |
No |
DELETE Bucket lifecycle |
HttpMethodEnum.DELETE |
SpecialParamEnum.LIFECYCLE |
Yes |
No |
PUT Bucket website |
HttpMethodEnum.PUT |
SpecialParamEnum.WEBSITE |
Yes |
No |
GET Bucket website |
HttpMethodEnum.GET |
SpecialParamEnum.WEBSITE |
Yes |
No |
DELETE Bucket website |
HttpMethodEnum.DELETE |
SpecialParamEnum.WEBSITE |
Yes |
No |
PUT Bucket versioning |
HttpMethodEnum.PUT |
SpecialParamEnum.VERSIONING |
Yes |
No |
GET Bucket versioning |
HttpMethodEnum.GET |
SpecialParamEnum.VERSIONING |
Yes |
No |
PUT Bucket cors |
HttpMethodEnum.PUT |
SpecialParamEnum.CORS |
Yes |
No |
GET Bucket cors |
HttpMethodEnum.GET |
SpecialParamEnum.CORS |
Yes |
No |
DELETE Bucket cors |
HttpMethodEnum.DELETE |
SpecialParamEnum.CORS |
Yes |
No |
PUT Bucket tagging |
HttpMethodEnum.PUT |
SpecialParamEnum.TAGGING |
Yes |
No |
GET Bucket tagging |
HttpMethodEnum.GET |
SpecialParamEnum.TAGGING |
Yes |
No |
DELETE Bucket tagging |
HttpMethodEnum.DELETE |
SpecialParamEnum.TAGGING |
Yes |
No |
PUT Object |
HttpMethodEnum.PUT |
N/A |
Yes |
Yes |
Append Object |
HttpMethodEnum.POST |
SpecialParamEnum.APPEND |
Yes |
Yes |
GET Object |
HttpMethodEnum.GET |
N/A |
Yes |
Yes |
PUT Object - Copy |
HttpMethodEnum.PUT |
N/A |
Yes |
Yes |
DELETE Object |
HttpMethodEnum.DELETE |
N/A |
Yes |
Yes |
DELETE Objects |
HttpMethodEnum.POST |
SpecialParamEnum.DELETE |
Yes |
Yes |
Obtain Object Metadata |
HttpMethodEnum.HEAD |
N/A |
Yes |
Yes |
PUT Object acl |
HttpMethodEnum.PUT |
SpecialParamEnum.ACL |
Yes |
Yes |
GET Object acl |
HttpMethodEnum.GET |
SpecialParamEnum.ACL |
Yes |
Yes |
Initiate Multipart Upload |
HttpMethodEnum.POST |
SpecialParamEnum.UPLOADS |
Yes |
Yes |
PUT Part |
HttpMethodEnum.PUT |
N/A |
Yes |
Yes |
PUT Part - Copy |
HttpMethodEnum.PUT |
N/A |
Yes |
Yes |
List Parts |
HttpMethodEnum.GET |
N/A |
Yes |
Yes |
Complete Multipart Upload |
HttpMethodEnum.POST |
N/A |
Yes |
Yes |
DELETE Multipart upload |
HttpMethodEnum.DELETE |
N/A |
Yes |
Yes |
POST Object restore |
HttpMethodEnum.POST |
SpecialParamEnum.RESTORE |
Yes |
Yes |
To access OBS using a temporary URL generated by the OBS Android SDK, perform the following steps:
- Call ObsClient.createTemporarySignature to generate a signed URL.
- Use any HTTP library to make an HTTP/HTTPS request to OBS.
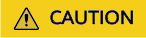
If a CORS or signature mismatch error occurs, refer to the following steps to troubleshoot the issue:
- If CORS has not been configured, you need to first configure CORS rules on the console. For details, see Configuring CORS.
- If the signatures do not match, check whether signature parameters are correct by referring to Authentication of Signature in a URL. For example, during an object upload, the backend uses Content-Type to calculate the signature and generate an authorized URL, but if Content-Type is not set or set wrongly when the frontend uses the authorized URL, there will be a CORS error. To address this issue, ensure that Content-Type fields at the frontend and backend are consistent.
The following content provides examples of accessing OBS using a temporary URL, including bucket creation, as well as object upload, download, listing, and deletion.
Creating a Bucket
// Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY_ID. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. String ak = System.getenv("ACCESS_KEY_ID"); String sk = System.getenv("SECRET_ACCESS_KEY_ID"); String endPoint = "https://your-endpoint"; // Create an ObsClient instance. ObsClient obsClient = new ObsClient(ak, sk, endPoint); // Specify the validity period of the URL to 3600 seconds. long expireSeconds = 3600L; TemporarySignatureRequest request = new TemporarySignatureRequest(HttpMethodEnum.PUT, expireSeconds); request.setBucketName("bucketname"); TemporarySignatureResponse response = obsClient.createTemporarySignature(request); Log.i("CreateTemporarySignature", "Creating bucket using temporary signature url:"); Log.i("CreateTemporarySignature", "\t" + response.getSignedUrl()); Request.Builder builder = new Request.Builder(); for (Map.Entry<String, String> entry : response.getActualSignedRequestHeaders().entrySet()) { builder.header(entry.getKey(), entry.getValue()); } // Make a PUT request to create a bucket. String location = "your bucket location"; Request httpRequest = builder.url(response.getSignedUrl()).put(RequestBody.create(null, "<CreateBucketConfiguration><LocationConstraint>" + location + "</LocationConstraint></CreateBucketConfiguration>".getBytes())).build(); OkHttpClient httpClient = new OkHttpClient.Builder().followRedirects(false).retryOnConnectionFailure(false) .cache(null).build(); Call c = httpClient.newCall(httpRequest); Response res = c.execute(); Log.i("CreateTemporarySignature", "\tStatus:" + res.code()); if (res.body() != null) { Log.i("CreateTemporarySignature", "\tContent:" + res.body().string() + "\n"); } res.close();
Uploading an Object
// Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY_ID. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. String ak = System.getenv("ACCESS_KEY_ID"); String sk = System.getenv("SECRET_ACCESS_KEY_ID"); String endPoint = "https://your-endpoint"; // Create an ObsClient instance. ObsClient obsClient = new ObsClient(ak, sk, endPoint); // Specify the validity period of the URL to 3600 seconds. long expireSeconds = 3600L; Map<String, String> headers = new HashMap<String, String>(); String contentType = "text/plain"; headers.put("Content-Type", contentType); TemporarySignatureRequest request = new TemporarySignatureRequest(HttpMethodEnum.PUT, expireSeconds); request.setBucketName("bucketname"); request.setObjectKey("objectname"); request.setHeaders(headers); TemporarySignatureResponse response = obsClient.createTemporarySignature(request); Log.i("CreateTemporarySignature", "Creating object using temporary signature url:"); Log.i("CreateTemporarySignature", "\t" + response.getSignedUrl()); Request.Builder builder = new Request.Builder(); for (Map.Entry<String, String> entry : response.getActualSignedRequestHeaders().entrySet()) { builder.header(entry.getKey(), entry.getValue()); } //Make a PUT request to upload an object. Request httpRequest = builder.url(response.getSignedUrl()).put(RequestBody.create(MediaType.parse(contentType), "Hello OBS".getBytes("UTF-8"))).build(); OkHttpClient httpClient = new OkHttpClient.Builder().followRedirects(false).retryOnConnectionFailure(false) .cache(null).build(); Call c = httpClient.newCall(httpRequest); Response res = c.execute(); Log.i("CreateTemporarySignature", "\tStatus:" + res.code()); if (res.body() != null) { Log.i("CreateTemporarySignature", "\tContent:" + res.body().string() + "\n"); } res.close();
Downloading an Object
// Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY_ID. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. String ak = System.getenv("ACCESS_KEY_ID"); String sk = System.getenv("SECRET_ACCESS_KEY_ID"); String endPoint = "https://your-endpoint"; // Create an ObsClient instance. ObsClient obsClient = new ObsClient(ak, sk, endPoint); // Specify the validity period of the URL to 3600 seconds. long expireSeconds = 3600L; TemporarySignatureRequest request = new TemporarySignatureRequest(HttpMethodEnum.GET, expireSeconds); request.setBucketName("bucketname"); request.setObjectKey("objectname"); TemporarySignatureResponse response = obsClient.createTemporarySignature(request); Log.i("CreateTemporarySignature", "Getting object using temporary signature url:"); Log.i("CreateTemporarySignature", "\t" + response.getSignedUrl()); Request.Builder builder = new Request.Builder(); for (Map.Entry<String, String> entry : response.getActualSignedRequestHeaders().entrySet()) { builder.header(entry.getKey(), entry.getValue()); } //Make a GET request to download an object. Request httpRequest = builder.url(response.getSignedUrl()).get().build(); OkHttpClient httpClient = new OkHttpClient.Builder().followRedirects(false).retryOnConnectionFailure(false) .cache(null).build(); Call c = httpClient.newCall(httpRequest); Response res = c.execute(); Log.i("CreateTemporarySignature", "\tStatus:" + res.code()); if (res.body() != null) { Log.i("CreateTemporarySignature", "\tContent:" + res.body().string() + "\n"); } res.close();
Listing Objects
// Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY_ID. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. String ak = System.getenv("ACCESS_KEY_ID"); String sk = System.getenv("SECRET_ACCESS_KEY_ID"); String endPoint = "https://your-endpoint"; // Create an ObsClient instance. ObsClient obsClient = new ObsClient(ak, sk, endPoint); // Specify the validity period of the URL to 3600 seconds. long expireSeconds = 3600L; TemporarySignatureRequest request = new TemporarySignatureRequest(HttpMethodEnum.GET, expireSeconds); request.setBucketName("bucketname"); TemporarySignatureResponse response = obsClient.createTemporarySignature(request); Log.i("CreateTemporarySignature", "Getting object list using temporary signature url:"); Log.i("CreateTemporarySignature", "\t" + response.getSignedUrl()); Request.Builder builder = new Request.Builder(); for (Map.Entry<String, String> entry : response.getActualSignedRequestHeaders().entrySet()) { builder.header(entry.getKey(), entry.getValue()); } //Make a GET request to obtain the object list. Request httpRequest = builder.url(response.getSignedUrl()).get().build(); OkHttpClient httpClient = new OkHttpClient.Builder().followRedirects(false).retryOnConnectionFailure(false) .cache(null).build(); Call c = httpClient.newCall(httpRequest); Response res = c.execute(); Log.i("CreateTemporarySignature", "\tStatus:" + res.code()); if (res.body() != null) { Log.i("CreateTemporarySignature", "\tContent:" + res.body().string() + "\n"); } res.close();
Deleting an Object
// Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY_ID. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. String ak = System.getenv("ACCESS_KEY_ID"); String sk = System.getenv("SECRET_ACCESS_KEY_ID"); String endPoint = "https://your-endpoint"; // Create an ObsClient instance. ObsClient obsClient = new ObsClient(ak, sk, endPoint); // Specify the validity period of the URL to 3600 seconds. long expireSeconds = 3600L; TemporarySignatureRequest request = new TemporarySignatureRequest(HttpMethodEnum.DELETE, expireSeconds); request.setBucketName("bucketname"); request.setObjectKey("objectname"); TemporarySignatureResponse response = obsClient.createTemporarySignature(request); Log.i("CreateTemporarySignature", "Deleting object using temporary signature url:"); Log.i("CreateTemporarySignature", "\t" + response.getSignedUrl()); Request.Builder builder = new Request.Builder(); for (Map.Entry<String, String> entry : response.getActualSignedRequestHeaders().entrySet()) { builder.header(entry.getKey(), entry.getValue()); } //Make a DELETE request to delete an object. Request httpRequest = builder.url(response.getSignedUrl()).delete().build(); OkHttpClient httpClient = new OkHttpClient.Builder().followRedirects(false).retryOnConnectionFailure(false) .cache(null).build(); Call c = httpClient.newCall(httpRequest); Response res = c.execute(); Log.i("CreateTemporarySignature", "\tStatus:" + res.code()); if (res.body() != null) { Log.i("CreateTemporarySignature", "\tContent:" + res.body().string() + "\n"); } res.close();

HttpMethodEnum is an enumeration function defined in OBS Android SDK, whose value indicates the request method types.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot