Creating a Bucket
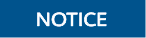
If you have any questions during development, post them on the Issues page of GitHub.
You can call create_bucket or create_bucket_with_params to create a bucket for object storage and use create_pfs_bucket to create a bucket used as a parallel file system.
Parameter Description
Field |
Type |
Mandatory or Optional |
Description |
---|---|---|---|
option |
The context of the bucket. For details, see Configuring option. |
Mandatory |
Bucket parameter |
canned_acl |
obs_canned_acl. See Managing Bucket ACLs. |
Mandatory |
Access control policy |
location_constraint |
char * |
Optional |
Location where a bucket will be created. If the used endpoint is obs.myhuaweicloud.com, this parameter is not required. If any other endpoint is used, this parameter is required. |
handler |
obs_response_handler * |
Mandatory |
Callback function |
callback_data |
void * |
Optional |
Callback data |
param |
obs_create_bucket_params * |
Mandatory |
This field is used only in the create_bucket_with_params interface. The body contains:
|
Sample Code
The following code shows how to create a bucket for object storage: static void test_create_bucket(obs_canned_acl canned_acl, char *bucket_name) { // Create and initialize option. obs_options option; obs_status ret_status = OBS_STATUS_BUTT; init_obs_options(&option); option.bucket_options.host_name = "<your-endpoint; option.bucket_options.bucket_name = "<Your bucketname>"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. option.bucket_options.access_key = getenv("ACCESS_KEY_ID"); option.bucket_options.secret_access_key = getenv("SECRET_ACCESS_KEY"); // Set the response callback function. obs_response_handler response_handler = { 0, &response_complete_callback }; // Create a bucket. For details about the predefined access policy, see section "Managing Bucket ACLs". create_bucket(&option, "<bucket ACL>", NULL, &response_handler, &ret_status); if (ret_status == OBS_STATUS_OK) { printf("create bucket successfully. \n"); } else { printf("create bucket failed(%s).\n", obs_get_status_name(ret_status)); } } The following code shows how to create a 3-AZ bucket: static void test_create_3az_bucket(obs_canned_acl canned_acl, char *bucket_name) { // Create and initialize option. obs_options option; obs_status ret_status = OBS_STATUS_BUTT; init_obs_options(&option); option.bucket_options.host_name = "<your-endpoint; option.bucket_options.bucket_name = "<Your bucketname>"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY. // Obtain an AK/SK pair on the management console. option.bucket_options.access_key = getenv("ACCESS_KEY_ID"); option.bucket_options.secret_access_key = getenv("SECRET_ACCESS_KEY"); // Set response callback function. obs_response_handler response_handler = { 0, &response_complete_callback }; // Create a bucket. For details about the predefined access policy, see section "Managing Bucket ACLs". obs_create_bucket_params create_param; create_param.canned_acl = canned_acl; create_param.location_constraint = "Region name that support 3AZ"; create_param.az_redundancy = OBS_REDUNDANCY_3AZ; create_bucket_with_params(&option, &create_param, &response_handler, &ret_status); if (ret_status == OBS_STATUS_OK) { printf("create bucket with params successfully. \n"); } else { printf("create bucket with params failed(%s).\n", obs_get_status_name(ret_status)); } } The following code shows how to create a bucket used as a parallel file system: static void test_create_pfs_bucket(obs_canned_acl canned_acl, char *bucket_name) { // Create and initialize option. obs_options option; obs_status ret_status = OBS_STATUS_BUTT; init_obs_options(&option); option.bucket_options.host_name = "<your-endpoint; option.bucket_options.bucket_name = "<Your bucketname>"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html.. option.bucket_options.access_key = getenv("ACCESS_KEY_ID"); option.bucket_options.secret_access_key = getenv("SECRET_ACCESS_KEY"); // Set response callback function. obs_response_handler response_handler = { 0, &response_complete_callback }; // Create a bucket. For details about the predefined access policy, see section "Managing Bucket ACLs". create_pfs_bucket(&option, "<bucket ACL>", NULL, &response_handler, &ret_status); if (ret_status == OBS_STATUS_OK) { printf("create bucket successfully. \n"); } else { printf("create bucket failed(%s).\n", obs_get_status_name(ret_status)); } }

Bucket names are globally unique. Ensure that the bucket you create is named differently from any other bucket. A bucket name must comply with the following rules:
- Contains 3 to 63 characters, starts with a digit or letter, and supports only lowercase letters, digits, hyphens (-), and periods (.)
- Cannot be an IP address.
- Cannot start or end with a hyphen (-) or period (.).
- Cannot contain two consecutive periods (.), for example, my..bucket.
- Cannot contain periods (.) and hyphens (-) adjacent to each other, for example, my-.bucket or my.-bucket.
- If you create buckets of the same name in a region, no error will be reported and the bucket properties comply with those set in the first creation request.
The bucket created in the previous example is of the default ACL (private), in the OBS Standard storage class, and in the default location where the global domain resides.
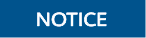
- During bucket creation, if the endpoint you use corresponds to the default region CN North-Beijing1 (cn-north-1), specifying a region is not a must. If the endpoint you use corresponds to any other region, except the default one, you must set the region to the one that the used endpoint corresponds to. To view the valid regions, see Regions and Endpoints. For example, if the endpoint used for initialization is obs.ap-southeast-1.myhuaweicloud.com, you must set Location to ap-southeast-1 when you create a bucket. Otherwise, status code 400 will be returned.
Creating a Bucket with Parameters Specified
When creating a bucket, you can specify the ACL, storage class, and location for the bucket. OBS provides three storage classes for buckets. For details, see Storage Class. Sample codes are as follows:
obs_status ret_status = OBS_STATUS_BUTT; // Create and initialize option. obs_options option; init_obs_options(&option); option.bucket_options.host_name = "<your-endpoint>"; option.bucket_options.bucket_name = "<Your bucketname>"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. option.bucket_options.access_key = getenv("ACCESS_KEY_ID"); option.bucket_options.secret_access_key = getenv("SECRET_ACCESS_KEY"); // Set response callback function. obs_response_handler response_handler = { 0, &response_complete_callback }; // Set the storage class for a bucket. option.bucket_options.storage_class = "<Your bucket storage policy>"; // Create a bucket. The predefined access policy and location of the bucket can be set for the input parameter. create_bucket(&option, "<bucket ACL>", "<Your bucket location>", &response_handler, &ret_status); if (ret_status == OBS_STATUS_OK) { printf("create bucket successfully. \n"); } else { printf("create bucket failed(%s).\n", obs_get_status_name(ret_status)); }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot