Performing a Resumable Download

If you have any questions during development, post them on the Issues page of GitHub. For details about parameters and usage of each API, see the API Reference.
Downloading large files often fails due to poor network conditions or program breakdowns. It is a waste of resources to restart the download process upon a download failure, and the restarted download process may still suffer from the unstable network. To resolve such issues, you can use the API for resumable download, whose working principle is to divide the to-be-downloaded file into multiple parts and download them separately. The download result of each part is recorded in a checkpoint file in real time. Only when all parts are successfully downloaded, the result indicating a successful download will be returned. Otherwise, an error is returned in callback function to remind you of calling the API again for re-downloading. Based on the download status of each part recorded in the checkpoint file, the re-downloading will download the parts failed to be downloaded previously, instead of downloading all parts. By virtue of this, resources are saved and efficiency is improved.
You can call ObsClient.downloadFile to perform a resumable download. The following table describes the main parameters involved in this API.
Parameter |
Description |
---|---|
Bucket |
(Mandatory) Bucket name |
Key |
(Mandatory) Object name |
DownloadFile |
Full path of the local directory to which the object is downloaded. If this parameter is null, the downloaded object is saved in the directory where the program is executed. |
PartSize |
Part size, in bytes. The value ranges from 100 KB to 5 GB and defaults to 5 MB. |
TaskNum |
Maximum number of threads that can be concurrently executed for download. The default value is 20. |
EnableCheckpoint |
Whether to enable the resumable download mode. The default value is false, which indicates that this mode is disabled. |
CheckpointFile |
File used to record the download progress. This parameter is effective only in the resumable download mode. If this parameter is null, the file will be in the same local directory as the downloaded object. |
VersionId |
Object version |
IfModifiedSince |
Returns the object if it has been modified since the specified time; otherwise, an error is returned. |
IfUnmodifiedSince |
Returns the object if it has not been modified since the specified time; otherwise, an error is returned. |
IfMatch |
Returns the source object if its ETag is the same as the one specified by this parameter; otherwise, an error code is returned. |
IfNoneMatch |
Returns the source object if its ETag is different from the one specified by this parameter; otherwise, an error code is returned. |
Sample code:
// Import the OBS library. // Use npm to install the client. var ObsClient = require('esdk-obs-nodejs'); // Use the source code to install the client. // var ObsClient = require('./lib/obs'); // Create an instance of ObsClient. var obsClient = new ObsClient({ //Obtain an AK/SK pair using environment variables or import the AK/SK pair in other ways. Using hard coding may result in leakage. //Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. access_key_id: process.env.ACCESS_KEY_ID, secret_access_key: process.env.SECRET_ACCESS_KEY, server : 'https://your-endpoint' }); obsClient.downloadFile({ Bucket : 'bucketname', Key : 'objectname', // Set the local path to which the object is downloaded. DownloadFile : 'localfile', // Set the part size to 10 MB. PartSize : 10 * 1024 * 1024, // Enable the resumable download mode. EnableCheckpoint : true }, (err, result) => { if(err){ console.error('Error-->' + err); }else{ console.log('RequestId-->' + result.InterfaceResult.RequestId); console.log('LastModified-->' + result.InterfaceResult.LastModified); console.log('Metadata-->' + JSON.stringify(result.InterfaceResult.Metadata)); } });
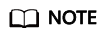
- The API for resumable download, which is implemented based on partial download, is an encapsulated and enhanced version of partial download.
- This API saves resources and improves efficiency upon the re-download, and speeds up the download process by concurrently downloading parts. Because this API is transparent to users, users are free from concerns about internal service details, such as the creation and deletion of checkpoint files, division of objects, and concurrent download of parts.
- The default value of the EnableCheckpoint parameter is false, which indicates that the resumable download mode is disabled. In such cases, this API degrades to the simple encapsulation of partial download, and no checkpoint file will be generated.
- CheckpointFile is effective only when EnableCheckpoint is true.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot