Notable Issues
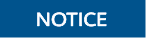
If you have any questions during development, post them on the Issues page of GitHub. For details about parameters and usage of each API, see the API Reference.
SignatureDoesNotMatch
Status-->403 Code-->SignatureDoesNotMatch
This error occurs if the SK input into ObsClient initialization is incorrect. Solution: Make sure that the SK is correct.
MethodNotAllowed
Status-->405 Code-->MethodNotAllowed
This error occurs because a feature on which the ObsClient API depends has not been rolled out on the requested OBS server. Contact the OBS O&M team for further confirmation.
Network Error
Error: Network Error
Possible causes are as follows:
- The endpoint input into ObsClient initialization is incorrect. Solution: Verify to make sure that the endpoint is correct.
- The network between the OBS client and OBS server is abnormal. Solution: Check the health status of the network.
- The OBS domain name resolved by DNS is inaccessible. Solution: Contact the OBS O&M team.
- The SDK depends on the compatibility of the underlying library Axios. Solution: Perform a browser-based upload or contact the OBS O&M team.
Request Timeout
timeout of xxx exceeded
Possible causes are as follows:
- The network latency between the OBS client and OBS server is too long. Solution: Check the health status of the network.
- The network between the OBS client and OBS server is abnormal. Solution: Check the health status of the network.
Cross-Origin Requests are Blocked
Access to XMLHttpRequest at 'xxx' from origin 'xxx' has been blocked by CORS policy: Response to preflight request doesn't pass access control check: No 'Access-Control-Allow-Origin' header is present on the requested resource.
This error occurs because the bucket does not have the CORS rule or the CORS rule is invalid. Solution: Reconfigure the bucket CORS by referring to Configuring the Bucket CORS.
The Request Is Successful, but Some Fields Are Missing in the Response
Possible causes are as follows:
- The Expose Header configuration in the CORS rule of a bucket is incomplete. For example, ETag is not configured, which makes the ETag value unable to be obtained after an object is successfully uploaded; or x-obs-request-id is not configured, which makes the request ID returned by the OBS server unable to be obtained after a request is complete. Solution: Reconfigure the CORS rule for the bucket by referring to Configuring CORS for a Bucket.
- SDK of an earlier version is used. Solution: Upgrade the SDK to the latest version. Download the latest version.
A Loading Error Occurs After the SDK Is Integrated into the Internet Explorer
SCRIPT5009: 'Promise' is not defined.
The cause is that the Internet Explorer does not support the Promise object (ES6). The solutions are as follows:
- Use esdk-obs-browserjs-x.x.x.min.js as the SDK library file instead of esdk-obs-browserjs-without-polyfill-x.x.x.min.js in the program.
- Introduce a third-party library to the Internet Explorer to supplement ES6. For example, introduce the babel-polyfill library.
- Use browsers such as Chrome and Firefox which support ES6.
Failed to Obtain the ETag Value after Upload
After a file is successfully uploaded by calling ObsClient.putObject or ObsClient.uploadPart, the returned result does not contain the ETag value. The possible causes are as follows:
- The configuration of ExposeHeader in the CORS rule of a bucket does not contain the ETag header field. Solution: Complete the full configuration of the CORS rule for the bucket by referring to Configuring CORS for a Bucket.
- The configuration of ExposeHeader in the CORS rule of a bucket contains the ETag header field, but the ETag header field is shielded in the result returned by the browser. This problem usually occurs on the browser of an earlier version. Solution: Upgrade the browser to a later version that supports HTML5.
ObsClient Is Not Defined
Uncaught ReferenceError: ObsClient is not defined
Possible causes are as follows:
- The SDK is not correctly introduced to the program. Solution: Check whether the esdk-obs-browserjs-x.x.x.min.js or esdk-obs-browserjs-without-polyfill-x.x.x.min.js file is correctly introduced by checking the method of introducing the SDK.
- The AMD modular components, such as require.js, are introduced to the program. Solution: Create an instance of ObsClient according to AMD.
- A component introduced in the program conflicts with the SDK dependent library (this scenario seldom occurs). Solution: Contact the OBS O&M team.
Unable to Upload Files Using a Browser that Does Not Support window.File
Error: source file must be an instance of window.File or window.Blob
The SDK depends on window.File provided by HTML5 to upload files. For browsers that do not support window.File, such as IE8 and IE9, files cannot be uploaded by calling ObsClient.putObject or ObsClient.uploadFile. Solution: Perform a browser-based upload. The procedure is as follows:
- Check whether the browser supports window.File. The code example is as follows:
function getBrowserInfo() { var agent = navigator.userAgent.toLowerCase(); var regStr_ie = /msie [\d.]+;/gi; var regStr_ff = /firefox\/[\d.]+/gi var regStr_chrome = /chrome\/[\d.]+/gi; var regStr_saf = /safari\/[\d.]+/gi; var isIE = agent.indexOf('compatible') > -1 && agent.indexOf('msie' > -1); var isEdge = agent.indexOf('edge') > -1 && !isIE; var isIE11 = agent.indexOf('trident') > -1 && agent.indexOf('rv:11.0') > -1; if (isIE) { var reIE = new RegExp('msie (\\d+\\.\\d+);'); reIE.test(agent); var fIEVersion = parseFloat(RegExp['$1']); if (fIEVersion == 7) { return 'IE/7'; } else if (fIEVersion == 8) { return 'IE/8'; } else if (fIEVersion == 9) { return 'IE/9'; } else if (fIEVersion == 10) { return 'IE/10'; } } // isIE end if (isIE11) { return 'IE/11'; } // Firefox if (agent.indexOf('firefox') > 0) { return agent.match(regStr_ff); } // Safari if (agent.indexOf('safari') > 0 && agent.indexOf('chrome') < 0) { return agent.match(regStr_saf); } // Chrome if (agent.indexOf('chrome') > 0) { return agent.match(regStr_chrome); } return ''; } var browserInfo = getBrowserInfo(); // Check whether the browser supports window.File. var isSupportFileApi = browserInfo !== 'IE/7' && browserInfo !== 'IE/8' && browserInfo !== 'IE/9' && window.File;
- Select a proper upload method based on the result in step 1. The code example is as follows:
function postObject(){ // Use JS code to submit a form for the browser-based upload. } if(isSupportFileApi){ // Upload files in browser-based mode. return postObject(); } // Create an ObsClient instance. var obsClient = new ObsClient({ // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables AccessKeyID and SecretAccessKey. // The front-end code does not have the process environment variable, so you need to use a module bundler like webpack to define the process variable. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. access_key_id: process.env.AccessKeyID, secret_access_key: process.env.SecretAccessKey, // Replace the example endpoint with the actual one in your case. server: 'https://obs.ap-southeast-1.myhuaweicloud.com' }); // Use the resumable upload API of the SDK to upload files. obsClient.uploadFile({ // Transfer the request parameter. }, function (err, result) { // Process the callback function. });
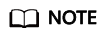
The size of a file for browser-based upload cannot exceed 5 GB.
Undefinition Caused by the Introduction of the CommonJS Specification
Uncaught (in promise) TypeError: Cannot read property 'CancelToken' of undefined
The cause is that a modular component of the CommonJS specification is introduced to the program, such as webpack. Solution: Upgrade the SDK to 3.19.5 or a later version.
Undefinition Caused by the Introduction of Mock.js
Uncaught TypeError: request.upload.addEventListener in not a function
The cause is that the Mock.js component is used to stub XHR in the program. The solutions are as follows:
- Workaround: Disable the progress bar function when the SDK is used for upload, download, and resumable upload.
- Replace: Replace the Mock.js component with a component that can simulate all XHR interfaces.
- Extend: Extend the Mock.js component by supplementing the Mock.js interfaces that do not support XHR.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot