Listing Versioning Objects
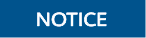
If you have any questions during development, post them on the Issues page of GitHub.
You can call list_versions to list versioning objects in a bucket.
The following table describes the parameters involved in this API.
Field |
Type |
Mandatory or Optional |
Description |
---|---|---|---|
option |
The context of the bucket. For details, see Configuring option. |
Mandatory |
Bucket parameter |
prefix |
char * |
Optional |
Name prefix that the objects to be listed must contain |
key_marker |
char * |
Optional |
Versioning object name to start with when listing versioning objects in a bucket. All versioning objects are listed in the lexicographical order. |
delimiter |
char * |
Optional |
Character used to group object names. If the object name contains the delimiter parameter, the character string from the first character to the first delimiter in the object name is grouped under a single result element, common_prefixes. (If a prefix is specified in the request, the prefix must be removed from the object name.) |
version_id_marker |
char * |
Optional |
Version ID to start with when listing objects in a bucket. All objects are listed in the lexicographical order by object name and version ID. This parameter must be used together with key_marker. |
maxkeys |
int |
Mandatory |
Maximum number of objects listed in the response body. The value ranges from 1 to 1000. If the value is not in this range, 1000 objects are returned by default. |
handler |
obs_list_versions_handler * |
Mandatory |
Callback function |
callback_data |
void * |
Optional |
Callback data |

- If the value of version_id_marker is not a version ID specified by key_marker, version_id_marker is ineffective.
- The returned result of list_versions includes the versioning objects and delete markers.
Sample code:
static void test_list_versions() { char *prefix = "o"; char *key_marker = "obj"; char *delimiter = "/"; int maxkeys = 10; // Create and initialize option. obs_options option; init_obs_options(&option); option.bucket_options.host_name = "<your-endpoint>"; option.bucket_options.bucket_name = "<Your bucketname>"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. option.bucket_options.access_key = getenv("ACCESS_KEY_ID"); option.bucket_options.secret_access_key = getenv("SECRET_ACCESS_KEY"); // Set response callback function. obs_list_versions_handler list_versions_handler = { { &response_properties_callback, &list_versions_complete_callback }, &listVersionsCallback }; // Create and Initialize callback data. list_versions_callback_data data; char* version_id_marker = NULL; memset(&data, 0, sizeof(list_bucket_callback_data)); data.ret_status = OBS_STATUS_BUTT; snprintf(data.next_key_marker, sizeof(data.next_key_marker), "%s", key_marker); if (version_id_marker) { snprintf(data.next_versionId_marker, sizeof(data.next_versionId_marker), "%s", version_id_marker); } data.keyCount = 0; data.allDetails = 1; data.is_truncated = 0; // When listing versioning objects, you can list objects in paging mode by specifying the object prefix using prefix and specifying the number using maxkeys. delimiter specifies the character in the group listing. To group in folders, set delimiter to forward slash (/), use key_marker to specify the start position of the versioning, and use version_id_marker to specify the version IDs. list_versions(&option, prefix, key_marker, delimiter, maxkeys, version_id_marker, &list_versions_handler, &data); if (OBS_STATUS_OK == data.ret_status) { printf("list versions successfully. \n"); } else { printf("list versions failed(%s).\n", obs_get_status_name(data.ret_status)); } }

- Information about a maximum of 1000 versioning objects can be listed each time. If a bucket contains more than 1000 objects and is_truncated is true in the returned result, not all versioning objects are listed. In such cases, you can use next_key_marker and next_versionId_marker to obtain the start position for next listing.
- If you want to obtain all versioning objects in a specified bucket, you can use the paging mode for listing objects.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot