Setting or Obtaining a Versioning Object ACL
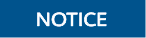
If you have any questions during development, post them on the Issues page of GitHub.
Directly Setting a Versioning Object ACL
You can call set_object_acl to input the version ID (version_id) to set the ACL for a versioning object. Sample code is as follows:
static void test_set_object_acl_version() { obs_status ret_status = OBS_STATUS_BUTT; // Create and initialize option. obs_options option; init_obs_options(&option); option.bucket_options.host_name = "<your-endpoint>"; option.bucket_options.bucket_name = "<Your bucketname>"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. option.bucket_options.access_key = getenv("ACCESS_KEY_ID"); option.bucket_options.secret_access_key = getenv("SECRET_ACCESS_KEY"); // Set response callback function. obs_response_handler response_handler = { 0, &response_complete_callback }; // Initialize the ACL information and specify the object name and version ID. manager_acl_info aclinfo; init_acl_info(&aclinfo); aclinfo.object_info.key = key; aclinfo.object_info.version_id = version_id; // Set the object ACL. set_object_acl(&option, &aclinfo, &response_handler, &ret_status); if (OBS_STATUS_OK == ret_status) { printf("set object acl successfully. \n"); } else { printf("set object acl failed(%s).\n", obs_get_status_name(ret_status)); } // Free memory. deinitialize_acl_info(&aclinfo); }

The owner or grantee ID required in the ACL indicates an account ID, which can be viewed on the My Credentials page of OBS Console.
Obtaining a Versioning Object ACL
You can call get_object_acl to pass the version ID (version_id) to obtain the ACL for a versioning object. Sample code is as follows:
static void test_get_object_acl_version() { obs_status ret_status = OBS_STATUS_BUTT; // Create and initialize option. obs_options option; init_obs_options(&option); option.bucket_options.host_name = "<your-endpoint>"; option.bucket_options.bucket_name = "<Your bucketname>"; //Read the AK/SK from environment variables. option.bucket_options.access_key = getenv("ACCESS_KEY_ID"); option.bucket_options.secret_access_key = getenv("SECRET_ACCESS_KEY"); // Set response callback function. obs_response_handler response_handler = { 0, &response_complete_callback }; // Create an ACL structure and specify the object name and version ID. manager_acl_info *aclinfo = malloc_acl_info(); aclinfo->object_info.key = key; aclinfo->object_info.version_id = version_id; // Obtain the object ACL. get_object_acl(&option, aclinfo, &response_handler, &ret_status); if (OBS_STATUS_OK == ret_status) { printf("get object acl: -------------"); printf("%s %s %s %s\n", aclinfo->owner_id, aclinfo->owner_display_name, aclinfo->object_info.key, aclinfo->object_info.version_id); if (aclinfo->acl_grant_count_return) { print_grant_info(*aclinfo->acl_grant_count_return, aclinfo->acl_grants); } } else { printf("get object acl failed(%s).\n", obs_get_status_name(ret_status)); } // Free memory. free_acl_info(&aclinfo); }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot