How Do I Specify Content-SHA256? (SDK for Python)
You can upload the x-obs-content-sha256 header when uploading an object or a part. The value of this header is the hexadecimal value converted from the SHA256 value of the request body and is calculated from Hex(SHA256Hash(<payload>). The server calculates and verifies the SHA256 value of the message body in the request with x-obs-content-sha256 included, which may make performance deteriorate, but is recommended for security purposes. Sample code for uploading an object is as follows:
# -*- coding:utf-8 -*- # This example uploads a file with content-sha256 specified. from obs import ObsClient import os import traceback import hashlib # Obtain an AK and SK pair using environment variables or import the AK and SK pair in other ways. Using hard coding may result in leakage. # Obtain an AK and SK pair from the management console by referring to https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. ak = os.getenv("AccessKeyID") sk = os.getenv("SecretAccessKey") # (Optional) If you use a temporary AK and SK pair and a security token to access OBS, obtain them from environment variables. security_token = os.getenv("SecurityToken") # Set server to the endpoint corresponding to the bucket. CN-Hong Kong is used here as an example. Replace it with the one currently in use. server = "https://obs.ap-southeast-1.myhuaweicloud.com" # Calculate SHA256 of the file. def getSha256(file_path): # Read the file content in binary mode. with open(file_path, 'rb') as f: # Create an SHA256 hash object. sha256_hash = hashlib.sha256() # Read the file content cyclically and update the hash value. while True: data = f.read(65536) # Read 64 KB each time. if not data: break sha256_hash.update(data) # Return the hexadecimal representation of the hash value. sha256 = sha256_hash.hexdigest() f.close() return sha256 # Create an obsClient instance. # If you use a temporary AK and SK pair and a security token to access OBS, you must specify security_token when creating an instance. obsClient = ObsClient(access_key_id=ak, secret_access_key=sk, server=server) bucketName = 'examplebucket' objectKey = 'example/objectname1' file_path = 'D:\\example.txt' # Specify the user-defined header. extensionHeaders = {'x-obs-content-sha256': getSha256(file_path)} try: # Upload a file. resp = obsClient.putFile(bucketName, objectKey, file_path, extensionHeaders=extensionHeaders) # If status code 2xx is returned, the API is called successfully. Otherwise, the API call fails. if resp.status < 300: print('Put File Succeeded') print('requestId:', resp.requestId) print('etag:', resp.body.etag) print('versionId:', resp.body.versionId) else: print('Put File Failed') print('requestId:', resp.requestId) print('errorCode:', resp.errorCode) print('errorMessage:', resp.errorMessage) except: print(traceback.format_exc())
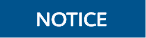
Python SDK supports integrity check with both MD5 and SHA256 (recommended for security purposes).
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot