Setting Website Hosting
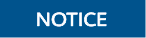
If you have any questions during development, post them on the Issues page of GitHub.
You can call set_bucket_website_configuration to set website hosting on a bucket.
Configuring the Default Homepage, Error Pages and Redirection Rules
The following code shows how to configure the default home page, error pages, and redirection rules. The following table describes the parameters.
Field |
Type |
Mandatory or Optional |
Description |
---|---|---|---|
option |
The context of the bucket. For details, see Configuring option. |
Mandatory |
Bucket parameter |
set_bucket_redirect_all |
obs_set_bucket_redirect_all_conf * |
Mandatory |
Indicates the redirection configuration. |
set_bucket_redirect_all->host_name |
const char * |
Mandatory |
Indicates the name of the host where requests will be redirected. |
set_bucket_redirect_all->protocol |
const char * |
Optional |
The HTTP or HTTPS protocol used in redirecting requests. The default protocol is HTTP. |
set_bucket_website_conf |
obs_set_bucket_website_conf * |
Mandatory |
Describes the configuration of the website element in the redirection rules. |
set_bucket_website_conf->suffix |
const char * |
Mandatory |
Suffix that is appended to a request initiated for a directory on the website endpoint. For example, if the suffix is index.html and you request for samplebucket/images/, the data that is returned will be the object with the key name images/index.html in the samplebucket bucket. Suffix cannot be empty or contain slashes (/). |
set_bucket_website_conf->key |
const char * |
Optional |
Indicates the object name that is used when a 4XX error occurs. This element identifies the page that is returned when a 4XX error occurs. |
set_bucket_website_conf->routingrule_info |
bucket_website_routingrule * |
Optional |
For details about redirection rules, see the following table. |
set_bucket_website_conf->routingrule_count |
int |
Optional |
Total size of set_bucket_website_conf.routingrule_info |
handler |
obs_response_handler* |
Mandatory |
Callback function |
callback_data |
void * |
Optional |
Callback data |
The following table describes the redirection rule structure bucket_website_routingrule.
Field |
Type |
Mandatory or Optional |
Description |
---|---|---|---|
key_prefix_equals |
const char * |
Optional |
Indicates the object name prefix when the redirection is applied. |
http_errorcode_returned_equals |
const char * |
Optional |
Indicates HTTP error codes when the redirection takes effect. The specified redirection is applied only when the error code returned equals this value. |
protocol |
const char * |
Optional |
Indicates protocol used in the redirection request. |
host_name |
const char * |
Optional |
Indicates the host name used in the redirection request. |
replace_key_prefix_with |
const char * |
Optional |
Indicates the object name prefix used in the redirection request. |
replace_key_with |
const char * |
Optional |
Indicates the object name used in the redirection request. |
http_redirect_code |
const char * |
Optional |
Indicates the HTTP status code returned after the redirection request. |
static void test_set_bucket_website_configuration() { obs_status ret_status = OBS_STATUS_BUTT; // Create and initialize option. obs_options option; init_obs_options(&option); option.bucket_options.host_name = "<your-endpoint>"; option.bucket_options.bucket_name = "<Your bucketname>"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. option.bucket_options.access_key = getenv("ACCESS_KEY_ID"); option.bucket_options.secret_access_key = getenv("SECRET_ACCESS_KEY"); obs_set_bucket_website_conf set_bucket_website_conf; // Configure the default homepage. set_bucket_website_conf.suffix = "index.html"; // Configure the error pages. set_bucket_website_conf.key = "Error.html"; // Define redirection rules. set_bucket_website_conf.routingrule_count = 2; bucket_website_routingrule temp[2]; memset(&temp[0], 0, sizeof(bucket_website_routingrule)); memset(&temp[1], 0, sizeof(bucket_website_routingrule)); set_bucket_website_conf.routingrule_info = temp; temp[0].key_prefix_equals = "key_prefix1"; temp[0].replace_key_prefix_with = "replace_key_prefix1"; temp[0].http_errorcode_returned_equals="404"; temp[0].http_redirect_code = NULL; temp[0].host_name = "www.example.com"; temp[0].protocol = "http"; temp[1].key_prefix_equals = "key_prefix2"; temp[1].replace_key_prefix_with = "replace_key_prefix2"; temp[1].http_errorcode_returned_equals="404"; temp[1].http_redirect_code = NULL; temp[1].host_name = "www.example.com"; temp[1].protocol = "http"; // Set response callback function. obs_response_handler response_handler = { 0, &response_complete_callback }; // Set redirection rules. set_bucket_website_configuration(&option, NULL, &set_bucket_website_conf, &response_handler, &ret_status); if (OBS_STATUS_OK == ret_status) { printf("set bucket website conf successfully. \n"); } else { printf("set bucket website conf failed(%s).\n", obs_get_status_name(ret_status)); } }
Configuring Redirection for All Requests
Sample code:
static void test_set_bucket_website_all() { obs_status ret_status = OBS_STATUS_BUTT; // Create and initialize option. obs_options option; init_obs_options(&option); option.bucket_options.host_name = "<your-endpoint>"; option.bucket_options.bucket_name = "<Your bucketname>"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. option.bucket_options.access_key = getenv("ACCESS_KEY_ID"); option.bucket_options.secret_access_key = getenv("SECRET_ACCESS_KEY"); // Set response callback function. obs_response_handler response_handler = { 0, &response_complete_callback }; // Configure redirection for all requests. obs_set_bucket_redirect_all_conf set_bucket_redirect_all; set_bucket_redirect_all.host_name = "www.example.com"; set_bucket_redirect_all.protocol = "https"; set_bucket_website_configuration(&option, &set_bucket_redirect_all, NULL, &response_handler, &ret_status); if (OBS_STATUS_OK == ret_status) { printf("set bucket website all successfully. \n"); } else { printf("set bucket website all failed(%s).\n", obs_get_status_name(ret_status)); } }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot