Downloading an Object
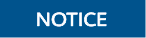
If you have any questions during development, post them on the Issues page of GitHub.
You can use the get_object function to download an object.
Parameter Description
Field |
Type |
Mandatory or Optional |
Description |
---|---|---|---|
option |
The context of the bucket. For details, see Configuring option. |
Mandatory |
Bucket parameter |
object_info |
obs_object_info * |
Mandatory |
Object name and version number. For non-multi-version objects, set version to 0. |
get_conditions |
obs_get_conditions * |
Mandatory |
Sets filter conditions of the object and read range. |
encryption_params |
server_side_encryption_params * |
Optional |
Obtains the decryption settings of an object. |
handler |
obs_get_object_handler * |
Mandatory |
Callback function |
callback_data |
void * |
Optional |
Callback data |
Sample Code
static void test_get_object() { char *file_name = "./test"; obs_object_info object_info; // Initialize option. obs_options option; init_obs_options(&option); option.bucket_options.host_name = "<your-endpoint>"; option.bucket_options.bucket_name = "<Your bucketname>"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. option.bucket_options.access_key = getenv("ACCESS_KEY_ID"); option.bucket_options.secret_access_key = getenv("SECRET_ACCESS_KEY"); // Set the object to be downloaded. memset(&object_info, 0, sizeof(obs_object_info)); object_info.key = "<object key>"; object_info.version_id = "<object version ID>"; // Set the structure for storing downloaded object data based on service requirements. get_object_callback_data data; data.ret_status = OBS_STATUS_BUTT; data.outfile = write_to_file(file_name); // Define range download parameters. obs_get_conditions getcondition; memset(&getcondition, 0, sizeof(obs_get_conditions)); init_get_properties(&getcondition); getcondition.start_byte = "<start byte>"; // Download length. The default value is 0, indicating that the object end is read. getcondition.byte_count = "<byte count>"; // Customize callback function for download. obs_get_object_handler get_object_handler = { { &response_properties_callback, &get_object_complete_callback}, &get_object_data_callback }; get_object(&option, &object_info, &getcondition, 0, &get_object_handler, &data); if (OBS_STATUS_OK == data.ret_status) { printf("get object successfully. \n"); } else { printf("get object faied(%s).\n", obs_get_status_name(data.ret_status)); } fclose(data.outfile); }

- You can perform operations on the input streams of an object to read and write the object contents to a local file or to the memory.
- getcondition can be left empty, indicating that the entire object is downloaded.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot