Managing Object ACLs
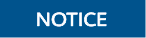
If you have any questions during development, post them on the Issues page of GitHub.
Object ACLs, similar to bucket ACLs, support predefined ACLs (For details, see Managing Bucket ACLs) and direct configurations.
An object ACL can be configured in any of the following ways:
- Specify a pre-defined access control policy during object upload.
- Call set_object_acl_by_head to specify a pre-defined access control policy.
- Call set_object_acl to set the ACL directly.
Specifying a Pre-defined Access Control Policy During Object Upload
Sample code:
static void test_put_object_acl() { // Create and initialize option. obs_options option; init_obs_options(&option); option.bucket_options.host_name = "<your-endpoint>"; option.bucket_options.bucket_name = "<Your bucketname>"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. option.bucket_options.access_key = getenv("ACCESS_KEY_ID"); option.bucket_options.secret_access_key = getenv("SECRET_ACCESS_KEY"); // Initialize put_properties which can be used to set object properties. obs_put_properties put_properties; init_put_properties(&put_properties); // Specify a pre-defined access control policy. put_properties.canned_acl = OBS_CANNED_ACL_PUBLIC_READ_WRITE; // Callback data put_file_object_callback_data data; memset(&data, 0, sizeof(put_file_object_callback_data)); // Read the file to be uploaded to the callback data. data.infile = 0; data.noStatus = 1; content_length = read_bytes_from_file("<Uploaded filename>", &data); // Callback function obs_put_object_handler putobjectHandler = { { &response_properties_callback, &response_complete_callback }, &put_buffer_object_data_callback }; // Upload data streams. put_object(&option,"<object key>", content_length, &put_properties, 0, &putobjectHandler, &data); if (OBS_STATUS_OK == data.ret_status) { printf("put object from file successfully. \n"); } else { printf("put object failed(%s).\n", obs_get_status_name(data.ret_status)); } }
Setting a Pre-defined Access Control Policy for an Object
You can use set_object_acl_by_head to set object properties. The following table describes the parameters.
Field |
Type |
Mandatory or Optional |
Description |
---|---|---|---|
option |
The context of the bucket. For details, see Configuring option. |
Mandatory |
Bucket parameter |
object_info |
obs_object_info * |
Mandatory |
Object name and version number. For non-multi-version objects, set version to 0. |
canned_acl |
obs_canned_acl |
Mandatory |
For details about how to manage bucket access rights, see Table 1 in Managing Bucket ACLs. |
handler |
obs_response_handler * |
Mandatory |
Callback function |
callback_data |
void * |
Optional |
Callback data |
Sample code:
void test_set_object_acl_byhead() { obs_status ret_status = OBS_STATUS_BUTT; // Create and initialize option. obs_options option; init_obs_options(&option); option.bucket_options.host_name = "<your-endpoint>"; option.bucket_options.bucket_name = "<Your bucketname>"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. option.bucket_options.access_key = getenv("ACCESS_KEY_ID"); option.bucket_options.secret_access_key = getenv("SECRET_ACCESS_KEY"); // Set response callback function. obs_response_handler response_handler = { 0, &response_complete_callback }; obs_canned_acl canned_acl = OBS_CANNED_ACL_PUBLIC_READ_WRITE; obs_object_info object_info; object_info.key = "<object key>"; object_info.version_id = "<object version ID>"; //Set a pre-defined access control policy for an object. set_object_acl_by_head(&option, &object_info, canned_acl, &response_handler, &ret_status); if (ret_status == OBS_STATUS_OK) { printf("set bucket acl by head successfully. \n"); } else { printf("set bucket acl by head failed(%s).\n", obs_get_status_name(ret_status)); } }
Directly Setting an Object ACL
You can use set_object_acl to set object properties. The following table describes the parameters.
Field |
Type |
Mandatory or Optional |
Description |
---|---|---|---|
option |
The context of the bucket. For details, see Configuring option. |
Mandatory |
Bucket parameter |
aclinfo |
manager_acl_info * |
Mandatory |
Structure for managing ACL permission information |
aclinfo->object_info |
obs_object_info * |
Mandatory |
Object name and version number. For non-multi-version objects, set version to 0. |
aclinfo->owner_id |
char * |
Optional |
User account ID |
aclinfo->acl_grant_count_return |
int * |
Mandatory |
Pointer to the number of returned aclinfo->acl_grants |
aclinfo->acl_grants |
obs_acl_grant * |
Mandatory |
Pointer to the permission information structure. For details, see Table 2 in Managing Bucket ACLs. |
aclinfo->object_delivered |
obs_object_delivered |
Optional |
Indicates whether the object ACL inherits the ACL of the bucket. Valid values are OBJECT_DELIVERED_TRUE and OBJECT_DELIVERED_FALSE. The default value is OBJECT_DELIVERED_TRUE. |
handler |
obs_response_handler * |
Mandatory |
Callback function |
callback_data |
void * |
Optional |
Callback data |
Sample code:
void test_set_object_acl() { obs_status ret_status = OBS_STATUS_BUTT; // Create and initialize option. obs_options option; init_obs_options(&option); option.bucket_options.host_name = "<your-endpoint>"; option.bucket_options.bucket_name = "<Your bucketname>"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. option.bucket_options.access_key = getenv("ACCESS_KEY_ID"); option.bucket_options.secret_access_key = getenv("SECRET_ACCESS_KEY"); // Set response callback function. obs_response_handler response_handler = { 0, &response_complete_callback }; // Define the object ACL manager_acl_info aclinfo; init_acl_info(&aclinfo); aclinfo.object_info.key = "<object key>"; aclinfo.object_info.version_id = "<object version ID>"; // Set the object ACL. set_object_acl(&option, &aclinfo, &response_handler, &ret_status); if (OBS_STATUS_OK == ret_status) { printf("set object acl successfully. \n"); } else { printf("set object acl failed(%s).\n", obs_get_status_name(ret_status)); } // Destroy the memory deinitialize_acl_info(&aclinfo); }

The owner or grantee ID needed in the ACL indicates the account ID, which can be viewed on the My Credentials page of OBS Console.
Obtaining the Object ACL
You can call get_object_acl to obtain an object ACL. Sample code:
void test_get_object_acl() { obs_status ret_status = OBS_STATUS_BUTT; // Create and initialize option. obs_options option; init_obs_options(&option); option.bucket_options.host_name = "<your-endpoint>"; option.bucket_options.bucket_name = "<Your bucketname>"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. option.bucket_options.access_key = getenv("ACCESS_KEY_ID"); option.bucket_options.secret_access_key = getenv("SECRET_ACCESS_KEY"); // Set response callback function. obs_response_handler response_handler = { 0, &response_complete_callback }; manager_acl_info *aclinfo = malloc_acl_info(); aclinfo->object_info.key = "<object key>"; aclinfo->object_info.version_id = "<object version ID>"; // Obtain the object ACL. get_object_acl(&option, aclinfo, &response_handler, &ret_status); if (OBS_STATUS_OK == ret_status) { printf("get object acl: -------------"); printf("%s %d %s %s\n", aclinfo->owner_id, aclinfo->object_delivered, aclinfo->object_info.key, aclinfo->object_info.version_id); if (aclinfo->acl_grant_count_return) { print_grant_info(*aclinfo->acl_grant_count_return, aclinfo->acl_grants); } } else { printf("get object acl failed(%s).\n", obs_get_status_name(ret_status)); } // Destroy the memory free_acl_info(&aclinfo); }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot