Enabling Bucket Logging
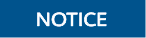
If you have any questions during development, post them on the Issues page of GitHub.
You can call set_bucket_logging_configuration_obs to enable bucket logging.
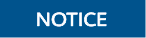
The source bucket and target bucket of logging must be in the same region.

If the bucket is in the OBS Infrequent Access or Archive storage class, it cannot be used as the target bucket.
Sample code:
Parameter Description
Field |
Type |
Mandatory or Optional |
Description |
---|---|---|---|
option |
The context of the bucket. For details, see Configuring option. |
Mandatory |
Bucket parameter |
target_bucket |
char * |
Mandatory |
When enabling the logging function, the owner of the bucket being logged can specify a target bucket to store the generated log files. Log files generated for multiple buckets can be stored in the same target bucket. If you do so, you need to specify different target_prefix to classify logs for different buckets. |
target_prefix |
char * |
Mandatory |
You can specify a prefix using this element so that log files are named with this prefix. |
agency |
char * |
This parameter is mandatory when enabling bucket logging. Do not select this parameter when disabling bucket logging. |
Name of the agency created by the owner of the logging bucket for uploading log files by OBS. |
acl_group |
obs_acl_group * |
Optional |
Structure of the permission information group |
acl_group->acl_grant_count |
int |
Optional |
The number of returned obs_acl_grant. |
acl_group->acl_grants |
obs_acl_grant * |
Optional |
Pointer to the permission information structure. For details, see Table 2 in Managing Bucket ACLs. |
handler |
obs_response_handler* |
Mandatory |
Callback function |
callback_data |
void * |
Optional |
Callback data |
Sample Code
static void test_set_bucket_logging_configuration() { // Grant the WRITE and READ_ACP permissions on the target bucket to the log delivery group. set_log_delivery_acl(bucket_name_target); obs_status ret_status = OBS_STATUS_BUTT; // Create and initialize option. obs_options option; init_obs_options(&option); option.bucket_options.host_name = "<your-endpoint>"; option.bucket_options.bucket_name = "<Your bucketname>"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. option.bucket_options.access_key = getenv("ACCESS_KEY_ID"); option.bucket_options.secret_access_key = getenv("SECRET_ACCESS_KEY"); // Set response callback function. obs_response_handler response_handler = { 0, &response_complete_callback }; // Configure bucket logging. set_bucket_logging_configuration_obs(&option, bucket_name_target, "prefix-log", "Your agency", NULL, &response_handler, &ret_status); if (ret_status == OBS_STATUS_OK) { printf("set bucket(%s) logging successfully. \n",bucket_name_src); } else { printf("set bucket logging faied(%s).\n",obs_get_status_name(ret_status)); } }
Setting ACLs for Objects to Be Logged
Sample code:
static void test_set_bucket_logging_configuration_acl() { // Grant the WRITE and READ_ACP permissions on the target bucket to the log delivery group. set_log_delivery_acl(bucket_name_target); obs_status ret_status = OBS_STATUS_BUTT; // Create and initialize option. obs_options option; init_obs_options(&option); option.bucket_options.host_name = "<your-endpoint>"; option.bucket_options.bucket_name = "<Your bucketname>"; //Read the AK/SK from environment variables. option.bucket_options.access_key = getenv("ACCESS_KEY_ID"); option.bucket_options.secret_access_key = getenv("SECRET_ACCESS_KEY"); // Set response callback function. obs_response_handler response_handler = { 0, &response_complete_callback }; int aclGrantCount = 2; obs_acl_grant acl_grants[2] = {0}; // Grant the FULL_CONTROL permission on logs to the authorized users. acl_grants[0].grantee_type = OBS_GRANTEE_TYPE_CANONICAL_USER; strcpy(acl_grants[0].grantee.canonical_user.id, "userid1"); strcpy(acl_grants[0].grantee.canonical_user.display_name, "dis1"); acl_grants[0].permission = OBS_PERMISSION_READ ; // Grant the READ permission on the objects to be logged to all users. acl_grants[1].grantee_type = OBS_GRANTEE_TYPE_ALL_OBS_USERS; acl_grants[1].permission = OBS_PERMISSION_READ ; obs_acl_group g; g.acl_grants = acl_grants; g.acl_grant_count = aclGrantCount; // Configure bucket logging. set_bucket_logging_configuration_obs(&option, bucket_name_target, "prefix-log", "Your agency", &g, &response_handler, &ret_status); if (ret_status == OBS_STATUS_OK) { printf("set bucket(%s) logging successfully. \n", bucket_name_src); } else { printf("set bucket logging faied(%s).\n",obs_get_status_name(ret_status)); } }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot