Performing a Modification
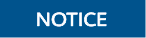
If you have any questions during development, post them on the Issues page of GitHub.
The modification API modify_object applies only to buckets used as parallel file systems. This API shares the same parameters and usage as that of put_object. The only difference is that the start position of the write object is added.
Parameter Description
Field |
Type |
Mandatory or Optional |
Description |
---|---|---|---|
option |
Request for the context of the bucket. For details, see Configuring option. |
Mandatory |
Bucket parameter |
key |
char * |
Mandatory |
Object name |
content_length |
uint64_t |
Mandatory |
Length of the object content |
position |
char * |
Mandatory |
Start position of the object to be modified |
put_properties |
obs_put_properties* |
Mandatory |
Properties of the uploaded object |
encryption_params |
server_side_encryption_params * |
Optional |
Encryption setting of the uploaded object |
handler |
obs_modify_object_handler * |
Mandatory |
Callback function |
callback_data |
void * |
Optional |
Callback data |
Sample Code
static void test_modify_object_from_buffer() { // Buffer to be uploaded char *buffer = "abcdefg"; // Length of the buffer to be uploaded int buffer_size = strlen(buffer); // Name of an object to be uploaded char *key = "put_buffer_test"; char * position = "0"; // Initialize option. obs_options option; init_obs_options(&option); option.bucket_options.host_name = "<your-endpoint>"; option.bucket_options.bucket_name = "<Your bucketname>"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. option.bucket_options.access_key = getenv("ACCESS_KEY_ID"); option.bucket_options.secret_access_key = getenv("SECRET_ACCESS_KEY"); option.bucket_options.certificate_info = "<Your certificate>"; // Initialize the properties of an object to be uploaded. obs_put_properties put_properties; init_put_properties(&put_properties); // Initialize the structure for storing uploaded data. put_buffer_object_callback_data data; memset(&data, 0, sizeof(put_buffer_object_callback_data)); // Assign the buffer value to the structure. data.put_buffer = buffer; // Set buffersize. data.buffer_size = buffer_size; // Set callback function. obs_modify_object_handler modify0bjectHandler = { { &response_properties_callback, &put_buffer_complete_callback }, &put_buffer_data_callback }; modify_object(&option, key, buffer_size, position, &put_properties, 0, &modify0bjectHandler, &data); if (OBS_STATUS_OK == data.ret_status) { printf("modify object from buffer successfully. \n"); } else { printf("modify object from buffer failed(%s).\n", obs_get_status_name(data.ret_status)); } }

- modify_object applies only to buckets used as parallel file systems.
- When you call modify_object for the first time, if the object to be modified does not exist, an error (HTTP status code: 404) will be reported.
- The sixth parameter in modify_object is used to provide the server-side encryption. For details, see section Server-Side Encryption.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot