Enabling Bucket Logging

If you have any questions during the development, post them on the Issues page of GitHub. For details about parameters and usage of each API, see the API Reference.
You can call ObsClient.SetBucketLogging to enable bucket logging

The source bucket and target bucket of logging must be in the same region.
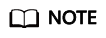
If the bucket is in the OBS Infrequent Access or Archive storage class, it cannot be used as the target bucket.
The Agency field indicates the name of the IAM agency for OBS created by the owner of the target bucket. For details about how to create an IAM agency, see the IAM User Guide.
Enabling Bucket Logging
Sample code:
// Initialize configuration parameters. ObsConfig config = new ObsConfig(); config.Endpoint = "https://your-endpoint"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables AccessKeyID and SecretAccessKey. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. string accessKey= Environment.GetEnvironmentVariable("AccessKeyID", EnvironmentVariableTarget.Machine); string secretKey= Environment.GetEnvironmentVariable("SecretAccessKey", EnvironmentVariableTarget.Machine); // Create an instance of ObsClient. ObsClient client = new ObsClient(accessKey, secretKey, config); try { // Set the source bucket logging. SetBucketLoggingRequest putrequest = new SetBucketLoggingRequest(); putrequest.BucketName = "bucketname"; putrequest.Configuration = new LoggingConfiguration(); putrequest.Configuration.TargetBucketName = "targetbucketname"; putrequest.Configuration.TargetPrefix = "access-log."; putrequest.Configuration.Agency= "your agency"; SetBucketLoggingResponse putresponse = client.SetBucketLogging(putrequest); Console.WriteLine("Set bucket logging response: {0}", putresponse.StatusCode); } catch (ObsException ex) { Console.WriteLine("ErrorCode: {0}", ex.ErrorCode); Console.WriteLine("ErrorMessage: {0}", ex.ErrorMessage); }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot