Performing a Multipart Upload

If you have any questions during development, post them on the Issues page of GitHub. For details about parameters and usage of each API, see the API Reference.
To upload a large file, multipart upload is recommended. Multipart upload is applicable to many scenarios, including:
- Files to be uploaded are larger than 100 MB.
- The network condition is poor. Connection to the OBS server is constantly down.
- Sizes of files to be uploaded are uncertain.
Multipart upload consists of three phases:
- Initiate a multipart upload (ObsClient.initiateMultipartUpload).
- Upload parts one by one or concurrently (ObsClient.uploadPart).
- Combine parts (ObsClient.completeMultipartUpload) or abort the multipart upload (ObsClient.abortMultipartUpload).
Initiating a Multipart Upload
Before using a multipart upload, you need to first initiate it. This operation will return an upload ID (globally unique identifier) created by the OBS server to identify the multipart upload. You can use this upload ID to initiate related operations, such as aborting the multipart upload, listing multipart uploads, and listing uploaded parts.
You can call ObsClient.initiateMultipartUpload to initiate a multipart upload.
// Import the OBS library. // Use npm to install the client. var ObsClient = require('esdk-obs-nodejs'); // Use the source code to install the client. // var ObsClient = require('./lib/obs'); // Create an ObsClient instance. var obsClient = new ObsClient({ //Obtain an AK/SK pair using environment variables or import the AK/SK pair in other ways. Using hard coding may result in leakage. //Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. access_key_id: process.env.ACCESS_KEY_ID, secret_access_key: process.env.SECRET_ACCESS_KEY, server : 'https://your-endpoint' }); obsClient.initiateMultipartUpload({ Bucket : 'bucketname', Key : 'objectname', ContentType : 'text/plain', Metadata : {'property' : 'property-value'} }, (err, result) => { if(err){ console.error('Error-->' + err); }else{ console.log('Status-->' + result.CommonMsg.Status); if(result.CommonMsg.Status < 300 && result.InterfaceResult){ console.log('UploadId-->' + result.InterfaceResult.UploadId); } } });
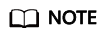
- When initiating a multipart upload, you can use the ContentType and Metadata parameters to respectively set the MIME type and custom metadata of an object.
- After the API for initiating a multipart upload is successfully called, an upload ID will be returned. This ID will be used in follow-up operations.
Uploading a Part
After initiating a multipart upload, you can specify the object name and upload ID to upload a part. Each upload part has a part number (ranging from 1 to 10000). For parts with the same upload ID, their part numbers are unique and identify their relative location in the object. If you use the same part number to upload two parts, the latter one uploaded will overwrite the former one. The last part uploaded ranges from 0 to 5 GB in size, and each of the other parts ranges from 100 KB to 5 GB. Parts can be uploaded in random order, or even through different processes or machines. OBS will combine them into the final object based on their part numbers.
You can call ObsClient.uploadPart to upload a part.
// Import the OBS library. // Use npm to install the client. var ObsClient = require('esdk-obs-nodejs'); // Use the source code to install the client. // var ObsClient = require('./lib/obs'); // Create an ObsClient instance. var obsClient = new ObsClient({ //Obtain an AK/SK pair using environment variables or import the AK/SK pair in other ways. Using hard coding may result in leakage. //Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. access_key_id: process.env.ACCESS_KEY_ID, secret_access_key: process.env.SECRET_ACCESS_KEY, server : 'https://your-endpoint' }); obsClient.uploadPart({ Bucket:'bucketname', Key:'objectname', // Set the part number, which ranges from 1 to 10000. PartNumber:1, // Set the upload ID. UploadId:'upload id from initiateMultipartUpload', // Set the large file to be uploaded. localfile is the path of the local file to be uploaded. You need to specify the file name. SourceFile: 'localfile', // Set the part size. PartSize: 5 * 1024 * 1024, // Set the start offset. Offset: 0 }, (err, result) => { if(err){ console.log('Error-->' + err); }else{ console.log('Status-->' + result.CommonMsg.Status); if(result.CommonMsg.Status < 300 && result.InterfaceResult){ console.log('ETag-->' + result.InterfaceResult.ETag); } } });
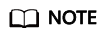
- Use the PartNumber parameter to specify the part number, the UploadId parameter to specify the globally unique ID, the SourceFile parameter to specify the to-be-uploaded file, the PartSize parameter to set the part size, and the Offset parameter to set the start offset of the file.
- Except the part last uploaded, other parts must be larger than 100 KB. Part sizes will not be verified during upload because which one is last uploaded is not identified until parts are combined.
- OBS will return ETags (MD5 values) of the received parts to users.
- You can use the ContentMD5 parameter to set the MD5 value for the object to be uploaded, which can be provided to the OBS server for data integrity verification.
- Part numbers range from 1 to 10000. If a part number exceeds this range, OBS will return a 400 Bad Request error.
- The minimum part size supported by an OBS 3.0 bucket is 100 KB, and the minimum part size supported by an OBS 2.0 bucket is 5 MB. You are advised to perform multipart upload to OBS 3.0 buckets.
Combining Parts
After all parts are uploaded, call the API for combining parts to generate the object. Before this operation, valid part numbers and ETags of all parts must be sent to OBS. After receiving this information, OBS verifies the validity of each part one by one. After all parts pass the verification, OBS combines these parts to form the final object.
You can call ObsClient.completeMultipartUpload to combine parts.
// Import the OBS library. // Use npm to install the client. var ObsClient = require('esdk-obs-nodejs'); // Use the source code to install the client. // var ObsClient = require('./lib/obs'); // Create an ObsClient instance. var obsClient = new ObsClient({ //Obtain an AK/SK pair using environment variables or import the AK/SK pair in other ways. Using hard coding may result in leakage. //Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. access_key_id: process.env.ACCESS_KEY_ID, secret_access_key: process.env.SECRET_ACCESS_KEY, server : 'https://your-endpoint' }); obsClient.completeMultipartUpload({ Bucket:'bucketname', Key:'objectname', // Set the upload ID. UploadId:'upload id from initiateMultipartUpload', Parts: [{'PartNumber':1,'ETag':'etag value from uploadPart'}] }, (err, result) => { if(err){ console.log('Error-->' + err); }else{ console.log('Status-->' + result.CommonMsg.Status); } });
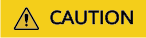
- If the size of a part other than the last part is smaller than 100 KB, OBS returns 400 Bad Request.
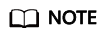
- Use the UploadId parameter to specify the upload ID for the multipart upload and the Parts parameter to specify the list of part numbers and ETags. Content in the list is displayed in the ascending order by part number.
- Part numbers can be inconsecutive.
Concurrently Uploading Parts
// Import the OBS library. // Use npm to install the client. const ObsClient = require('esdk-obs-nodejs'); // Use the source code to install the client. // var ObsClient = require('./lib/obs'); const fs = require('fs'); // Create an ObsClient instance. var obsClient = new ObsClient({ //Obtain an AK/SK pair using environment variables or import the AK/SK pair in other ways. Using hard coding may result in leakage. //Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. access_key_id: process.env.ACCESS_KEY_ID, secret_access_key: process.env.SECRET_ACCESS_KEY, server : 'https://your-endpoint' }); const Bucket = 'bucketname' const Key = 'objectname' // Specify the part size. const DEFAULT_PART_SIZE = 9 * 1024 * 1024; // Set the number of concurrent parts to 20. const CONCURRENCY = 20 // Prepare multipart upload parameters. const preparePartParams = (Bucket, Key, UploadId) => (sampleFile, partSize = DEFAULT_PART_SIZE) => { try { const fileSize = fs.lstatSync(sampleFile).size; const partCount = fileSize % partSize === 0 ? Math.floor(fileSize / partSize) : Math.floor(fileSize / partSize) + 1; const uploadPartParams = []; // Specify the concurrent upload. for (let i = 0; i < partCount; i++) { // Start position of parts in the file let Offset = i * partSize; // Part size let currPartSize = (i + 1 === partCount) ? fileSize - Offset : partSize; // Part number let PartNumber = i + 1; uploadPartParams.push({ Bucket, Key, PartNumber, UploadId, Offset, SourceFile: sampleFile, PartSize: currPartSize, }); } return ({ uploadPartParams, fileSize }); } catch (error) { console.log(error) } } /** * uploadSuccessSize: Size of the parts that have been uploaded * uploadSuccessCount: Number of the parts that have been uploaded * concurrency: Current concurrency */ let uploadSuccessSize = 0; let uploadSuccessCount = 0; let concurrency = 0 const parts = []; const uploadPart = (uploadPartParam, otherUploadPartInfo) => { const partCount = otherUploadPartInfo.partCount; const fileSize = otherUploadPartInfo.fileSize; concurrency++; return obsClient .uploadPart(uploadPartParam) .then(result => { const { PartNumber, PartSize } = uploadPartParam; if (result.CommonMsg.Status < 300) { uploadSuccessCount++; uploadSuccessSize += PartSize; console.log(`the current concurrent count is ${concurrency} | uploaded segment: ${uploadSuccessCount}/${partCount}. the progress is ${((uploadSuccessSize / fileSize) * 100).toFixed(2)}% | the partNumber ${PartNumber} upload successed.`) parts.push({ PartNumber, ETag: result.InterfaceResult.ETag }); } else { console.log(result.CommonMsg.Code, parts) } concurrency--; }).catch(function (err) { console.log(err); throw err; }) } const uploadFile = (sourceFile) => { obsClient.initiateMultipartUpload({ Bucket, Key }).then(res => { const Status = res.CommonMsg.Status; const UploadId = res.InterfaceResult.UploadId; if (typeof Status === 'number' && Status > 300) { console.log(`initiateMultipartUpload failed! Status:${Status}`); return; } const partParams = preparePartParams(Bucket, Key, UploadId)(sourceFile) const uploadPartParams = partParams.uploadPartParams; const fileSize = partParams.fileSize; const partCount = uploadPartParams.length; const otherUploadPartInfo = { fileSize, partCount } // Call the parallel upload function. parallelFunc(uploadPartParams, (param) => uploadPart(param, otherUploadPartInfo), CONCURRENCY) .then(() => { obsClient.completeMultipartUpload({ Bucket, Key, UploadId, Parts: parts.sort((a, b) => a.PartNumber - b.PartNumber) }, (err, result) => { if (err) { console.log('Error-->' + err); } else { console.log('Status-->' + result.CommonMsg.Status); } }); }) }).catch(function (err) { console.log(err) }) } /** * Implement the parallel execution. * @param {Array} params Parameter array of the callback function * @param {Promise} promiseFn Callback function * @param {number} limit Number of parallel parts */ const parallelFunc = (params, promiseFn, limit) => { return new Promise((resolve) => { let concurrency = 0; let finished = 0; const count = params.length; const run = (param) => { concurrency++; promiseFn(param) .then(() => { concurrency--; drainQueue(); finished++ if (finished === count) { resolve() } }); } const drainQueue = () => { while (params.length > 0 && concurrency < limit) { var param = params.shift(); run(param); } } drainQueue(); }) } uploadFile('localfile');
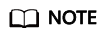
When uploading a large file in multipart mode, you need to use the Offset and PartSize parameters to set the start and end positions of each part in the file.
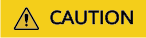
If there are too many concurrent parts, a timeout error may occur due to network instability or other causes. In such case, you need to limit the number of concurrent parts.
Aborting a Multipart Upload
After a multipart upload is aborted, you cannot use its upload ID to perform any operation and the uploaded parts will be deleted by OBS.
When an object is being uploaded in multi-part mode or an object fails to be uploaded, parts generated in the bucket. These parts occupy your storage space. You can cancel the multi-part uploading task to delete unnecessary parts, thereby saving the storage space.
You can call ObsClient.abortMultipartUpload to abort a multipart upload.
// Import the OBS library. // Use npm to install the client. var ObsClient = require('esdk-obs-nodejs'); // Use the source code to install the client. // var ObsClient = require('./lib/obs'); // Create an ObsClient instance. var obsClient = new ObsClient({ //Obtain an AK/SK pair using environment variables or import the AK/SK pair in other ways. Using hard coding may result in leakage. //Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. access_key_id: process.env.ACCESS_KEY_ID, secret_access_key: process.env.SECRET_ACCESS_KEY, server : 'https://your-endpoint' }); obsClient.abortMultipartUpload({ Bucket:'bucketname', Key:'objectname', // Set the upload ID. UploadId:'upload id from initiateMultipartUpload', }, (err, result) => { if(err){ console.log('Error-->' + err); }else{ console.log('Status-->' + result.CommonMsg.Status); } });
Listing Uploaded Parts
You can call ObsClient.listParts to list successfully uploaded parts of a multipart upload.
The following table describes the parameters involved in this API.
Parameter |
Description |
---|---|
UploadId |
Upload ID, which globally identifies a multipart upload. The value is in the returned result of ObsClient.initiateMultipartUpload. |
MaxParts |
Maximum number of parts that can be listed per page. |
PartNumberMarker |
Part number after which listing uploaded parts begins. Only parts whose part numbers are larger than this value will be listed. |
- Listing parts in simple mode
// Import the OBS library. // Use npm to install the client. var ObsClient = require('esdk-obs-nodejs'); // Use the source code to install the client. // var ObsClient = require('./lib/obs'); // Create an ObsClient instance. var obsClient = new ObsClient({ //Obtain an AK/SK pair using environment variables or import the AK/SK pair in other ways. Using hard coding may result in leakage. //Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. access_key_id: process.env.ACCESS_KEY_ID, secret_access_key: process.env.SECRET_ACCESS_KEY, server : 'https://your-endpoint' }); // List uploaded parts. uploadId is obtained from initiateMultipartUpload. obsClient.listParts({ Bucket : 'bucketname', Key: 'objectname', UploadId : 'upload id from initiateMultipartUpload' }, (err, result) => { if(err){ console.log('Error-->' + err); }else{ console.log('Status-->' + result.CommonMsg.Status); if(result.CommonMsg.Status < 300 && result.InterfaceResult){ for(let i=0;i<result.InterfaceResult.Parts.length;i++){ console.log('Part['+ i +']:'); // Part number, specified during the upload console.log('PartNumber-->' + result.InterfaceResult.Parts[i]['PartNumber']); // Time when the part was last uploaded console.log('LastModified-->' + result.InterfaceResult.Parts[i]['LastModified']); // Part ETag console.log('ETag-->' + result.InterfaceResult.Parts[i]['ETag']); // Part size console.log('Size-->' + result.InterfaceResult.Parts[i]['Size']); } } } });
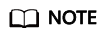
- A maximum of 1000 parts can be returned each time. If an upload of the specific upload ID contains more than 1000 parts and InterfaceResult.IsTruncated is true in the returned result, not all parts are returned. In such cases, you can use InterfaceResult.NextPartNumberMarker to obtain the start position for next listing.
- If you want to obtain all parts involved in a specific upload ID, you can use the paging mode for listing.
- Listing all parts
The following sample code lists more than 1,000 parts:
// Import the OBS library. // Use npm to install the client. var ObsClient = require('esdk-obs-nodejs'); // Use the source code to install the client. // var ObsClient = require('./lib/obs'); // Create an ObsClient instance. var obsClient = new ObsClient({ //Obtain an AK/SK pair using environment variables or import the AK/SK pair in other ways. Using hard coding may result in leakage. //Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. access_key_id: process.env.ACCESS_KEY_ID, secret_access_key: process.env.SECRET_ACCESS_KEY, server : 'https://your-endpoint' }); var listAll = (partNumberMarker) => { // List uploaded parts. uploadId is obtained from initiateMultipartUpload. obsClient.listParts({ Bucket : 'bucketname', Key: 'objectname', UploadId : 'upload id from initiateMultipartUpload', PartNumberMarker : partNumberMarker }, (err, result) => { if(err){ console.log('Error-->' + err); }else{ console.log('Status-->' + result.CommonMsg.Status); if(result.CommonMsg.Status < 300 && result.InterfaceResult){ for(let i=0;i<result.InterfaceResult.Parts.length;i++){ console.log('Part['+ i +']:'); // Part number, specified during the upload console.log('PartNumber-->' + result.InterfaceResult.Parts[i]['PartNumber']); // Time when the part was last uploaded console.log('LastModified-->' + result.InterfaceResult.Parts[i]['LastModified']); // Part ETag console.log('ETag-->' + result.InterfaceResult.Parts[i]['ETag']); // Part size console.log('Size-->' + result.InterfaceResult.Parts[i]['Size']); } if(result.InterfaceResult.IsTruncated === 'true'){ listAll(result.InterfaceResult.NextPartNumberMarker); } } } }); }; listAll();
Listing Multipart Uploads
You can call ObsClient.listMultipartUploads to list multipart uploads. The following table describes parameters involved in ObsClient.listMultipartUploads.
Parameter |
Description |
---|---|
Prefix |
Prefix that the object names in the multipart uploads to be listed must contain |
Delimiter |
Character used to group object names involved in multipart uploads. If the object name contains the Delimiter parameter, the character string from the first character to the first Delimiter parameter in the object name is grouped under a single result element, CommonPrefix. (If a prefix is specified in the request, the prefix must be removed from the object name.) |
MaxUploads |
Maximum number of multipart uploads listed in the response body. The value ranges from 1 to 1000. If the value exceeds 1000, only 1,000 multipart uploads are returned. |
KeyMarker |
Object name to start with when listing multipart uploads |
UploadIdMarker |
Upload ID after which the multipart upload listing begins. It is effective only when used with KeyMarker so that multipart uploads after UploadIdMarker of KeyMarker will be listed. |
- Listing multipart uploads in simple mode
// Import the OBS library. // Use npm to install the client. var ObsClient = require('esdk-obs-nodejs'); // Use the source code to install the client. // var ObsClient = require('./lib/obs'); // Create an ObsClient instance. var obsClient = new ObsClient({ //Obtain an AK/SK pair using environment variables or import the AK/SK pair in other ways. Using hard coding may result in leakage. //Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. access_key_id: process.env.ACCESS_KEY_ID, secret_access_key: process.env.SECRET_ACCESS_KEY, server : 'https://your-endpoint' }); obsClient.listMultipartUploads({ Bucket : 'bucketname' }, (err, result) => { if(err){ console.log('Error-->' + err); }else{ console.log('Status-->' + result.CommonMsg.Status); if(result.CommonMsg.Status < 300 && result.InterfaceResult){ for(let i=0;i<result.InterfaceResult.Uploads.length;i++){ console.log('Uploads[' + i + ']'); console.log('UploadId-->' + result.InterfaceResult.Uploads[i]['UploadId']); console.log('Key-->' + result.InterfaceResult.Uploads[i]['Key']); console.log('Initiated-->' + result.InterfaceResult.Uploads[i]['Initiated']); } } } });
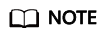
- Information about a maximum of 1000 multipart uploads can be returned each time. If a bucket contains more than 1000 multipart uploads and InterfaceResult.IsTruncated is true in the returned result, not all uploads will be returned. In such cases, you can use InterfaceResult.NextKeyMarker and InterfaceResult.NextUploadIdMarker to obtain the start position for next listing.
- If you want to obtain all multipart uploads in a bucket, you can use the paging listing.
- Listing all multipart uploads
// Import the OBS library. // Use npm to install the client. var ObsClient = require('esdk-obs-nodejs'); // Use the source code to install the client. // var ObsClient = require('./lib/obs'); // Create an ObsClient instance. var obsClient = new ObsClient({ //Obtain an AK/SK pair using environment variables or import the AK/SK pair in other ways. Using hard coding may result in leakage. //Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. access_key_id: process.env.ACCESS_KEY_ID, secret_access_key: process.env.SECRET_ACCESS_KEY, server : 'https://your-endpoint' }); var listAll = (keyMarker, uploadIdMarker) => { obsClient.listMultipartUploads({ Bucket : 'bucketname', KeyMarker : keyMarker, UploadIdMarker : uploadIdMarker }, (err, result) => { if(err){ console.log('Error-->' + err); }else{ console.log('Status-->' + result.CommonMsg.Status); if(result.CommonMsg.Status < 300 && result.InterfaceResult){ for(let i=0;i<result.InterfaceResult.Uploads.length;i++){ console.log('Uploads[' + i + ']'); console.log('UploadId-->' + result.InterfaceResult.Uploads[i]['UploadId']); console.log('Key-->' + result.InterfaceResult.Uploads[i]['Key']); console.log('Initiated-->' + result.InterfaceResult.Uploads[i]['Initiated']); } if(result.InterfaceResult.IsTruncated === 'true'){ listAll(result.InterfaceResult.NextKeyMarker, result.InterfaceResult.NextUploadIdMarker); } } } }); } listAll();
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot