Using a Temporary URL for Authorized Access
A temporarily authorized request is a URL temporarily authorized by specifying the AK and SK, request method, and related parameters. This URL contains authentication information and therefore you can use this URL to perform the specific operation in OBS. When the URL is being generated, you need to specify the validity period for it. All sub-classes inherited from OBSBaseRequest support temporary authentication.
The following table describes operations allowed for temporarily authorized requests.
Operation |
Class in OBS iOS SDK |
---|---|
PUT Bucket |
OBSCreateBucketRequest |
GET Buckets |
OBSListBucketsRequest |
DELETE Bucket |
OBSDeleteBucketRequest |
GET Objects |
OBSListObjectsRequest |
GET Object versions |
OBSListObjectsVersionsRequest |
List Multipart Uploads |
OBSListMultipartUploadsRequest |
Obtain Bucket Metadata |
OBSGetBucketMetaDataRequest |
GET Bucket location |
OBSGetBucketMetaDataRequest |
GET Bucket storageinfo |
OBSGetBucketStorageInfoRequest |
PUT Bucket quota |
OBSSetBucketQuotaRequest |
GET Bucket quota |
OBSGetBucketQuotaRequest |
PUT Bucket acl |
OBSSetBucketACLWithCannedACLRequest, OBSSetBucketACLWithPolicyRequest |
GET Bucket acl |
OBSGetBucketACLRequest |
PUT Bucket logging |
OBSSetBucketLoggingRequest |
GET Bucket logging |
OBSGetBucketLoggingRequest |
PUT Bucket policy |
OBSSetBucketPolicyRequest, OBSSetBucketPolicyWithStringRequest |
GET Bucket policy |
OBSGetBucketPolicyRequest |
DELETE Bucket policy |
OBSDeleteBucketPolicyRequest |
PUT Bucket lifecycle |
OBSSetBucketLifecycleRequest |
GET Bucket lifecycle |
OBSGetBucketLifecycleRequest |
DELETE Bucket lifecycle |
OBSDeleteBucketLifecycleRequest |
PUT Bucket website |
OBSSetBucketWebsiteRequest |
GET Bucket website |
OBSGetBucketWebsiteRequest |
DELETE Bucket website |
OBSDeleteBucketWebsiteRequest |
PUT Bucket versioning |
OBSSetBucketVersioningRequest |
GET Bucket versioning |
OBSGetBucketVersioningRequest |
PUT Bucket cors |
OBSSetBucketCORSRequest |
GET Bucket cors |
OBSGetBucketCORSRequest |
DELETE Bucket cors |
OBSDeleteBucketCORSRequest |
OPTIONS Bucket |
OBSOptionsBucketRequest |
PUT Bucket tagging |
OBSSetBucketTaggingRequest |
GET Bucket tagging |
OBSGetBucketTaggingRequest |
DELETE Bucket tagging |
OBSDeleteBucketTaggingRequest |
PUT Object |
OBSPutObjectWithDataRequest, OBSPutObjectWithFileRequest |
Append Object |
OBSAppendObjectWithFileRequest |
GET Object |
OBSGetObjectToDataRequest |
PUT Object - Copy |
OBSCopyObjectRequest |
DELETE Object |
OBSDeleteObjectRequest |
DELETE Objects |
OBSDeleteObjectsRequest |
Obtain Object Metadata |
OBSGetObjectMetaDataRequest |
PUT Object acl |
OBSSetObjectACLRequest |
GET Object acl |
OBSGetObjectACLRequest |
Initiate Multipart Upload |
OBSInitiateMultipartUploadRequest |
PUT Part |
OBSUploadPartWithDataRequest |
PUT Part - Copy |
OBSCopyPartRequest |
Listing Uploaded Parts |
OBSListPartsRequest |
Complete Multipart Upload |
OBSCompleteMultipartUploadRequest |
Abort Multipart Upload |
OBSAbortMultipartUploadRequest |
OPTIONS Object |
OBSOptionsObjectRequest |
POST Object restore |
OBSRestoreObjectRequest |
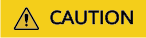
If a CORS or signature mismatch error occurs, refer to the following steps to troubleshoot the issue:
- If CORS is not configured, you need to configure CORS rules on OBS Console. For details, see Configuring CORS.
- If the signatures do not match, check whether signature parameters are correct by referring to Authentication of Signature in a URL. For example, during an object upload, the backend uses Content-Type to calculate the signature and generate an authorized URL, but if Content-Type is not set or is set to an incorrect value when the frontend uses the authorized URL, a CORS error occurs. To avoid this issue, ensure that Content-Type fields at both the frontend and backend are kept consistent.
You can call createV2PreSignedURL to create a temporary signed URL for an authorized request. Sample code is as follows:
Listing Objects
static OBSClient *client; NSString *endPoint = @"your-endpoint"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables AccessKeyID and SecretAccessKey. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. char* ak_env = getenv("AccessKeyID"); char* sk_env = getenv("SecretAccessKey"); NSString *AK = [NSString stringWithUTF8String:ak_env]; NSString *SK = [NSString stringWithUTF8String:sk_env]; // Initialize identity authentication. OBSStaticCredentialProvider *credentialProvider = [[OBSStaticCredentialProvider alloc] initWithAccessKey:AK secretKey:SK]; // Initialize service configuration. OBSServiceConfiguration *conf = [[OBSServiceConfiguration alloc] initWithURLString:endPoint credentialProvider:credentialProvider]; // Initialize an instance of OBSClient. client = [[OBSClient alloc] initWithConfiguration:conf]; OBSListObjectsRequest *request = [[OBSListObjectsRequest alloc] initWithBucketName:@"bucketname"]; // Create a V2 authorized access URL. [client createV2PreSignedURL:request expireAfter:3600 completionHandler:^(NSString *urlString, NSString *httpVerb, NSDictionary *signedHeaders) { NSLog(@"%@",urlString); }]
Obtaining an Object
static OBSClient *client; NSString *endPoint = @"your-endpoint"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables AccessKeyID and SecretAccessKey. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. char* ak_env = getenv("AccessKeyID"); char* sk_env = getenv("SecretAccessKey"); NSString *AK = [NSString stringWithUTF8String:ak_env]; NSString *SK = [NSString stringWithUTF8String:sk_env]; // Initialize identity authentication. OBSStaticCredentialProvider *credentialProvider = [[OBSStaticCredentialProvider alloc] initWithAccessKey:AK secretKey:SK]; // Initialize service configuration. OBSServiceConfiguration *conf = [[OBSServiceConfiguration alloc] initWithURLString:endPoint credentialProvider:credentialProvider]; // Initialize an instance of OBSClient. client = [[OBSClient alloc] initWithConfiguration:conf]; OBSGetObjectToDataRequest *request = [[OBSGetObjectToDataRequest alloc] initWithBucketName:@"bucketname" objectKey:@"objectkey"]; // Create a V2 authorized access URL. [client createV2PreSignedURL:request expireAfter:3600 completionHandler:^(NSString *urlString, NSString *httpVerb, NSDictionary *signedHeaders) { NSLog(@"%@",urlString); }]
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot