Setting Website Hosting

If you have any questions during development, post them on the Issues page of GitHub. For details about parameters and usage of each API, see the API Reference.
You can call ObsClient.setBucketWebsite to set website hosting for a bucket.
Configuring the Default Homepage and Error Pages
Sample code:
// Import the OBS library. // Use npm to install the client. var ObsClient = require('esdk-obs-nodejs'); // Use the source code to install the client. // var ObsClient = require('./lib/obs'); // Create an instance of ObsClient. const obsClient = new ObsClient({ //Obtain an AK/SK pair using environment variables or import the AK/SK pair in other ways. Using hard coding may result in leakage. //Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. access_key_id: process.env.ACCESS_KEY_ID, secret_access_key: process.env.SECRET_ACCESS_KEY, //CN-Hong Kong is used here as an example. Replace it with the one in your actual situation. server: 'https://obs.ap-southeast-1.myhuaweicloud.com' }); obsClient.setBucketWebsite({ Bucket: 'bucketname', // Configure the default homepage. IndexDocument:{Suffix:'index.html'}, // Configure the error pages. ErrorDocument:{Key:'error.html'} }, (err, result) => { if(err){ console.log('Error-->' + err); }else{ console.log('Status-->' + result.CommonMsg.Status); } });
Configuring the Redirection Rules
Sample code:
// Import the OBS library. // Use npm to install the client. var ObsClient = require('esdk-obs-nodejs'); // Use the source code to install the client. // var ObsClient = require('./lib/obs'); // Create an instance of ObsClient. const obsClient = new ObsClient({ //Obtain an AK/SK pair using environment variables or import the AK/SK pair in other ways. Using hard coding may result in leakage. //Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. access_key_id: process.env.ACCESS_KEY_ID, secret_access_key: process.env.SECRET_ACCESS_KEY, //CN-Hong Kong is used here as an example. Replace it with the one in your actual situation. server: 'https://obs.ap-southeast-1.myhuaweicloud.com' }); obsClient.setBucketWebsite({ Bucket: 'bucketname', // Configure the default homepage. IndexDocument:{Suffix:'index.html'}, // Configure the error pages. ErrorDocument:{Key:'error.html'}, // Configure the redirection rules. RoutingRules:[ { Condition:{HttpErrorCodeReturnedEquals:'404', KeyPrefixEquals: 'keyprefix'}, Redirect:{Protocol:'http',ReplaceKeyWith:'replacekeyprefix', HostName: 'www.example.com', HttpRedirectCode: '305'} } ] }, (err, result) => { if(err){ console.log('Error-->' + err); }else{ console.log('Status-->' + result.CommonMsg.Status); } });
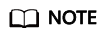
Use the RoutingRules parameter to set redirection rules for a bucket.
Configuring Redirection for All Requests
Sample code:
// Import the OBS library. // Use npm to install the client. var ObsClient = require('esdk-obs-nodejs'); // Use the source code to install the client. // var ObsClient = require('./lib/obs'); // Create an instance of ObsClient. const obsClient = new ObsClient({ //Obtain an AK/SK pair using environment variables or import the AK/SK pair in other ways. Using hard coding may result in leakage. //Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. access_key_id: process.env.ACCESS_KEY_ID, secret_access_key: process.env.SECRET_ACCESS_KEY, //CN-Hong Kong is used here as an example. Replace it with the one in your actual situation. server: 'https://obs.ap-southeast-1.myhuaweicloud.com' }); obsClient.setBucketWebsite({ Bucket: 'bucketname', RedirectAllRequestsTo : {HostName : 'www.example.com', Protocol : 'http'} }, (err, result) => { if(err){ console.log('Error-->' + err); }else{ console.log('Status-->' + result.CommonMsg.Status); } });
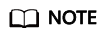
Use the RedirectAllRequestsTo parameter to set redirection rules for all requests for accessing a bucket.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot