Downloading an Archive Object
Before you can download an Archive object, you must restore it. Archive objects can be restored in either of the following ways.
Option |
Description |
Value in OBS iOS SDK |
---|---|---|
Expedited restore |
Data can be restored within 1 to 5 minutes. |
OBSRestoreTierExpedited |
Standard restore |
Data can be restored within 3 to 5 hours. This is the default option. |
OBSRestoreTierStandard |
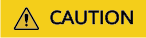
To prolong the validity period of the Archive data restored, you can repeatedly restore the Archive data, but you will be billed for each restore. After a second restore, the validity period of Standard object copies will be prolonged, and you need to pay for storing these copies during the prolonged period.
You can call OBSRestoreObjectRequest to restore Archive objects. Sample code is as follows:
static OBSClient *client; NSString *endPoint = @"your-endpoint"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables AccessKeyID and SecretAccessKey. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. char* ak_env = getenv("AccessKeyID"); char* sk_env = getenv("SecretAccessKey"); NSString *AK = [NSString stringWithUTF8String:ak_env]; NSString *SK = [NSString stringWithUTF8String:sk_env]; // Initialize identity authentication. OBSStaticCredentialProvider *credentialProvider = [[OBSStaticCredentialProvider alloc] initWithAccessKey:AK secretKey:SK]; //Initialize service configuration. OBSServiceConfiguration *conf = [[OBSServiceConfiguration alloc] initWithURLString:endPoint credentialProvider:credentialProvider]; // Initialize an instance of OBSClient. client = [[OBSClient alloc] initWithConfiguration:conf]; // Restore an object. OBSRestoreObjectRequest *request = [[OBSRestoreObjectRequest alloc]initWithBucketName:@"bucketname" objectKey:@"objectname" storeDays:[NSNumber numberWithInt:30]]; //1 to 30 request.restoreTier = OBSRestoreTierExpedited; OBSBFTask *task = [ self.client restoreObject:request completionHandler:^(OBSRestoreObjectResponse *response, NSError *error){ NSLog(@"%@",response); }]; // Wait for the object to be restored. sleep(6*60); // Download an object. NSString * outfilePath = [NSTemporaryDirectory() stringByAppendingString:@"filename"]; OBSGetObjectToFileRequest *request1 = [[OBSGetObjectToFileRequest alloc]initWithBucketName:@"bucketname" objectKey:@"objectname" downloadFilePath:outfilePath]; request1.downloadProgressBlock = ^(int64_t bytesWritten, int64_t totalBytesWritten, int64_t totalBytesExpectedToWrite) { NSLog(@"%0.1f%%",(float)floor(totalBytesWritten*10000/totalBytesExpectedToWrite)/100); }; [client getObject:request1 completionHandler:^(OBSGetObjectResponse *response, NSError *error){ NSLog(@"%@",response.etag); }];
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot