General Examples of ObsClient
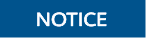
If you have any questions during development, post them on the Issues page of GitHub. For details about parameters and usage of each API, see the API Reference.
Result Returned via a Callback Function
ObsClient returns the results by using a callback function that contains two parameters in sequence: the exception information parameter and the SDK common result object parameter. If the exception information parameter in the callback function is not null, an error occurs during the API calling. Otherwise, the API calling is complete. In such conditions, you need to obtain the HTTP status code from the SDK common result object parameter to check whether the operation is successful. Sample code is as follows:
// Create an instance of ObsClient. var obsClient = new ObsClient({ // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables AccessKeyID and SecretAccessKey. // The front-end code does not have the process environment variable, so you need to use a module bundler like webpack to define the process variable. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. access_key_id: process.env.AccessKeyID, secret_access_key: process.env.SecretAccessKey, // Replace the example endpoint with the actual one in your case. server: 'https://obs.ap-southeast-1.myhuaweicloud.com' }); // Construct bucket request parameters. var requestParam1 = { Bucket : 'bucketname' // Other fields }; var callback1 = function (err, result){ // Handle the API calling result. }; // Call a bucket-related API, such as the API for obtaining the bucket location. obsClient.getBucketLocation(requestParam1, callback1); // Construct object request parameters. var requestParam2 = { Bucket : 'bucketname', Key : 'objectname' // Other fields. }; var callback2 = function (err, result){ // Handle the API calling result. }; // Call an object-related API, such as the API for downloading an object. obsClient.getObject(requestParam2, callback2);
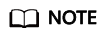
For bucket-related APIs, the Bucket field contained in the request object specifies the bucket name. For object-related APIs, the Bucket and Key fields contained in the request object specify the bucket name and object name, respectively.
Sample code:
// Create an instance of ObsClient. var obsClient = new ObsClient({ // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables AccessKeyID and SecretAccessKey. // The front-end code does not have the process environment variable, so you need to use a module bundler like webpack to define the process variable. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. access_key_id: process.env.AccessKeyID, secret_access_key: process.env.SecretAccessKey, // Replace the example endpoint with the actual one in your case. server: 'https://obs.ap-southeast-1.myhuaweicloud.com' }); // Call an API to perform related operation, for example, uploading an object. obsClient.putObject({ Bucket : 'bucketname', Key : 'objectname', Body : 'Hello OBS' }, function (err, result) { // If the exception information is not null, the API calling is abnormal. if(err){ console.log('Error-->' + err); }else{ //If the exception information is null, the API calling is complete and the HTTP status code will be checked. if(result.CommonMsg.Status < 300){// Operation succeeded if(result.InterfaceResult){ // Process the business logic after the operation is successful. } }else{// Obtain the exception details if the operation fails. console.log('Code-->' + result.CommonMsg.Code); console.log('Message-->' + result.CommonMsg.Message); console.log('HostId-->' + result.CommonMsg.HostId); console.log('RequestId-->' + result.CommonMsg.RequestId); } } });
Result Returned via the Promise Object
ObsClient supports results returned via the Promise object. If no exception is caught by the catch method of the Promise object, the API calling is complete. In such conditions, you need to obtain the HTTP status code from the SDK common result object to check whether the operation is successful. Sample code is as follows:
// Create an instance of ObsClient. var obsClient = new ObsClient({ // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables AccessKeyID and SecretAccessKey. // The front-end code does not have the process environment variable, so you need to use a module bundler like webpack to define the process variable. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. access_key_id: process.env.AccessKeyID, secret_access_key: process.env.SecretAccessKey, // Replace the example endpoint with the actual one in your case. server: 'https://obs.ap-southeast-1.myhuaweicloud.com' }); // Construct bucket request parameters. var requestParam1 = { Bucket : 'bucketname' // Other fields }; // Call a bucket-related API, such as the API for obtaining the bucket location. var promise1 = obsClient.getBucketLocation(requestParam1); promise1.then((result) => { // Handle the API calling result. }).catch((err)=>{ // Rectify the fault. }); // Construct object request parameters. var requestParam2 = { Bucket : 'bucketname', Key : 'objectname' // Other fields. }; // Call an object-related API, such as the API for downloading an object. var promise2 = obsClient.getObject(requestParam2, callback2); promise2.then((result) => { // Handle the API calling result. }).catch((err)=>{ // Rectify the fault. });
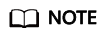
For bucket-related APIs, the Bucket field contained in the request object specifies the bucket name. For object-related APIs, the Bucket and Key fields contained in the request object specify the bucket name and object name, respectively.
Sample code:
// Import the OBS library. var ObsClient = require('./lib/obs'); // Create an instance of ObsClient. var obsClient = new ObsClient({ // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables AccessKeyID and SecretAccessKey. // The front-end code does not have the process environment variable, so you need to use a module bundler like webpack to define the process variable. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. access_key_id: process.env.AccessKeyID, secret_access_key: process.env.SecretAccessKey, // Replace the example endpoint with the actual one in your case. server: 'https://obs.ap-southeast-1.myhuaweicloud.com' }); // Call an API to perform related operation, for example, uploading an object. obsClient.putObject({ Bucket : 'bucketname', Key : 'objectname', Body : 'Hello OBS' }).then((result) => { // If no exception occurs and the API call is complete, check the HTTP status code. if(result.CommonMsg.Status < 300){// Operation succeeded if(result.InterfaceResult){ // Process the business logic after the operation is successful. } }else{// Obtain the exception details if the operation fails. console.log('Code-->' + result.CommonMsg.Code); console.log('Message-->' + result.CommonMsg.Message); console.log('HostId-->' + result.CommonMsg.HostId); console.log('RequestId-->' + result.CommonMsg.RequestId); } }).catch((err) => { // An exception occurred after the API is called. console.error('Error-->' + err); });
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot