Configuring CORS for a Bucket
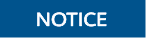
If you have any questions during development, post them on the Issues page of GitHub. For details about parameters and usage of each API, see the API Reference.
Parameter |
Value |
Description |
---|---|---|
Allowed Origin |
* |
Allows all request origins.
NOTE:
You can also configure a domain name or IP address. |
Allowed Method |
PUT, GET, POST, DELETE, HEAD |
Allows all HTTP methods. |
Allowed Header |
* |
Allows requests to carry any headers. |
Expose Header |
|
Allows the response to return the specified additional headers.
NOTICE:
This parameter specifies the additional headers that a browser can expose to the client. Take ETag as an example. It is not a standard response header, so to obtain its value, you need to add it in this parameter for the browser to return the value in the response. |
Configuring CORS on OBS Console
- Log in to OBS Console. In the bucket list, click the bucket you want. The bucket Overview page is displayed.
- In the Basic Configurations area, click CORS Rules. The CORS Rules page is displayed.
- Click Create. The Create CORS Rule dialog box is displayed. Set the parameters according to the preceding table. See the following figure.
- Click OK. On the CORS Rules page, view the configured rule.
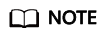
The CORS configuration of a bucket takes effect in two minutes. You can access the bucket using the OBS BrowserJS SDK only after the configuration takes effect.
Configuring CORS Using the OBS Java SDK
You can call ObsClient.setBucketCors of the OBS Java SDK to configure the CORS rule for a bucket. The sample code is as follows:
// Enter the endpoint corresponding to the bucket. CN North-Beijing4 is used here as an example. Replace it with the one in your actual situation. // Enter the endpoint corresponding to the bucket. CN-Hong Kong is used here as an example. Replace it with the one in your actual situation. // Enter the endpoint corresponding to the bucket. EU-Dublin is used here as an example. Replace it with the one in your actual situation. String endPoint = "https://obs.ap-southeast-1.myhuaweicloud.com"; String ak = System.getenv("ACCESS_KEY_ID"); String sk = System.getenv("SECRET_ACCESS_KEY_ID"); // Create an ObsClient instance. ObsClient obsClient = new ObsClient(ak, sk, endPoint); BucketCors cors = new BucketCors(); List<BucketCorsRule> rules = new ArrayList<BucketCorsRule>(); BucketCorsRule rule = new BucketCorsRule(); // Specify the origin of the cross-origin request. ArrayList<String> allowedOrigin = new ArrayList<String>(); allowedOrigin.add( "*"); rule.setAllowedOrigin(allowedOrigin); // Specify the methods of the cross-origin request. ArrayList<String> allowedMethod = new ArrayList<String>(); allowedMethod.add("GET"); allowedMethod.add("POST"); allowedMethod.add("PUT"); allowedMethod.add("DELETE"); allowedMethod.add("HEAD"); rule.setAllowedMethod(allowedMethod); // Specify the headers of the cross-origin request. ArrayList<String> allowedHeader = new ArrayList<String>(); allowedHeader.add("*"); rule.setAllowedHeader(allowedHeader); // Specify the additional headers in the response to the cross-origin request. ArrayList<String> exposeHeader = new ArrayList<String>(); exposeHeader.add("ETag"); exposeHeader.add("Content-Type"); exposeHeader.add("Content-Length"); exposeHeader.add("Cache-Control"); exposeHeader.add("Content-Disposition"); exposeHeader.add("Content-Encoding"); exposeHeader.add("Content-Language"); exposeHeader.add("Expires"); exposeHeader.add("x-obs-request-id"); exposeHeader.add("x-obs-id-2"); exposeHeader.add("x-reserved-indicator"); exposeHeader.add("x-obs-api"); exposeHeader.add("x-obs-version-id"); exposeHeader.add("x-obs-copy-source-version-id"); exposeHeader.add("x-obs-storage-class"); exposeHeader.add("x-obs-delete-marker"); exposeHeader.add("x-obs-expiration"); exposeHeader.add("x-obs-website-redirect-location"); exposeHeader.add("x-obs-restore"); exposeHeader.add("x-obs-version"); exposeHeader.add("x-obs-object-type"); exposeHeader.add("x-obs-next-append-position"); rule.setExposeHeader(exposeHeader); rule.setMaxAgeSecond(100); rules.add(rule); cors.setRules(rules); try { obsClient.setBucketCors("bucketname", cors); catch (ObsException e) { System.out.println("HTTP Code: " + e.getResponseCode()); System.out.println("Error Code:" + e.getErrorCode()); System.out.println("Error Message: " + e.getErrorMessage()); System.out.println("Request ID:" + e.getErrorRequestId()); System.out.println("Host ID:" + e.getErrorHostId()); } }
Configure CORS Using OBS Python SDK
You can call ObsClient.setBucketCors of the OBS Python SDK to configure the CORS of a bucket. The sample code is as follows:
# Import the module. from obs import ObsClient # Obtain an AK/SK pair using environment variables or import the AK/SK pair in other ways. Using hard coding may result in leakage. # Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. ak = os.getenv("AccessKeyID") sk = os.getenv("SecretAccessKey") # (Optional) If you use a temporary AK/SK pair and a security token to access OBS, obtain them from environment variables. security_token = os.getenv("SecurityToken") # Set server to the endpoint corresponding to the bucket. CN-Hong Kong is used here as an example. Replace it with the one in your actual situation. server = "https://obs.ap-southeast-1.myhuaweicloud.com" # Create an obsClient instance. # If you use a temporary AK/SK pair and a security token to access OBS, you must specify security_token when creating an instance. obsClient = ObsClient(access_key_id=ak, secret_access_key=sk, server=server) from obs import CorsRule # Specify the origin of the cross-origin request. allowedOrigin = ['*'] # Specify the methods of the cross-origin request. allowedMethod = ['PUT', 'POST', 'GET', 'DELETE', 'HEAD'] # Specify the headers of the cross-origin request. allowedHeader = ['*'] # Specify the additional headers in the response to the cross-origin request. exposeHeader = ['ETag', 'Content-Type', 'Content-Length', 'Cache-Control', 'Content-Disposition', 'Content-Encoding', 'Content-Language', 'Expires', 'x-obs-request-id', 'x-obs-id-2', 'x-reserved-indicator', 'x-obs-api', 'x-obs-version-id', 'x-obs-copy-source-version-id', 'x-obs-storage-class', 'x-obs-delete-marker', 'x-obs-expiration', 'x-obs-website-redirect-location', 'x-obs-restore', 'x-obs-version', 'x-obs-object-type', 'x-obs-next-append-position'] maxAgeSecond = 100 cors = CorsRule(id='rule1', allowedMethod=allowedMethod, allowedOrigin=allowedOrigin, allowedHeader=allowedHeader, maxAgeSecond=maxAgeSecond, exposeHeader=exposeHeader) resp = obsClient.setBucketCors('bucketname', corsList=[cors]) if resp.status < 300: print('requestId:', resp.requestId) else: print('errorCode:', resp.errorCode) print('errorMessage:', resp.errorMessage)
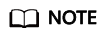
- Except the OBS BrowserJS SDK, all the other OBS SDKs can be used to configure CORS rules for buckets.
- You can also configure CORS for a bucket by referring to Configuring CORS on OBS Console or the CORS rule configuration section in the SDK Developer Guide of a specific language.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot