Performing a Resumable Upload
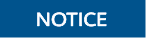
If you have any questions during development, post them on the Issues page of GitHub. For details about parameters and usage of each API, see the API Reference.
The principle of resumable upload is to divide the files to be uploaded into several parts and upload them separately. The upload results are recorded in the resumable upload result in real time. The upload success result is returned only when all parts are successfully uploaded. If not all parts are successfully uploaded, an error code is returned in the callback function to remind the user to call the API again for upload.
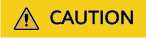
- The total size of files uploaded by the resumable upload API must be larger than 100 KB.
You can call ObsClient.uploadFile to perform a resumable upload. The following table describes the parameters involved in this API.
Parameter |
Description |
---|---|
Bucket |
Bucket name |
Key |
Object name |
RequestDate |
Request time
NOTE:
When the parameter type is String, the value must comply with the ISO8601 or RFC822 standards. |
SourceFile |
Source file to be uploaded (The browser must support FileReader.) |
UploadCheckpoint |
Resumable upload recording object, which can be obtained through ResumeCallback. |
PartSize |
Part size, in bytes. The value range is 100 KB to 5 GB. The default value is 9 MB. |
TaskNum |
Maximum number of parts that can be concurrently uploaded. The default value is 1. |
ProgressCallback |
Callback function for obtaining the upload progress
NOTE:
This callback function contains the following parameters in sequence: Number of uploaded bytes, total bytes, and used time (unit: second). |
EventCallback |
Callback function for obtaining the upload event
NOTE:
The callback function contains the following parameters: Event type, event parameter, and event result. |
ResumeCallback |
Callback function for obtaining the resumable upload control parameters.
NOTE:
|
// Create an instance of ObsClient. var obsClient = new ObsClient({ // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables AccessKeyID and SecretAccessKey. // The front-end code does not have the process environment variable, so you need to use a module bundler like webpack to define the process variable. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. access_key_id: process.env.AccessKeyID, secret_access_key: process.env.SecretAccessKey, // Replace the example endpoint with the actual one in your case. server: 'https://obs.ap-southeast-1.myhuaweicloud.com' }); var cp; var hook; obsClient.uploadFile({ Bucket : 'bucketname', Key : 'objectname', SourceFile : document.getElementById('input-file').files[0], PartSize : 9 * 1024 * 1024, ProgressCallback : function(transferredAmount, totalAmount, totalSeconds){ console.log(transferredAmount * 1.0 / totalSeconds / 1024); console.log(transferredAmount * 100.0 / totalAmount); if(hook && (transferredAmount / totalAmount) > 0.5){ // Suspend a resumable download task. hook.cancel(); } }, EventCallback : function(eventType, eventParam, eventResult){ // Handle event response. }, ResumeCallback : function(resumeHook, uploadCheckpoint){ // Obtain the control parameters for canceling resumable upload. hook = resumeHook; // Record a breakpoint. cp = uploadCheckpoint; } }, function(err, result){ console.error('Error-->' + err); // If an error occurs, call the resumable upload API again to continue the upload task. if(err){ obsClient.uploadFile({ UploadCheckpoint : cp, ProgressCallback : function(transferredAmount, totalAmount, totalSeconds){ console.log(transferredAmount * 1.0 / totalSeconds / 1024); console.log(transferredAmount * 100.0 / totalAmount); }, EventCallback : function(eventType, eventParam, eventResult){ // Handle event response. }, }, function(err, result){ if(err){ console.error('Error-->' + err); }else{ if(result.CommonMsg.Status < 300){ console.log('RequestId-->' + result.InterfaceResult.RequestId); console.log('Bucket-->' + result.InterfaceResult.Bucket); console.log('Key-->' + result.InterfaceResult.Key); console.log('Location-->' + result.InterfaceResult.Location); }else{ console.log('Code-->' + result.CommonMsg.Code); console.log('Message-->' + result.CommonMsg.Message); } } }); }else { console.log('Status-->' + result.CommonMsg.Status); if (result.CommonMsg.Status < 300 && result.InterfaceResult) { console.log('RequestId-->' + result.InterfaceResult.RequestId); } } });
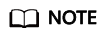
- The API for resumable upload, which is implemented based on multipart upload, is an encapsulated and enhanced version of multipart upload.
- The breakpoint record object can be obtained through ResumeCallback. The sourceFile field in the resumable upload record object indicates the file to be uploaded. This field needs to be set again after the browser is restarted.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot