Creating an Instance of OBSClient
OBSClient functions as the iOS client for accessing OBS. It offers users a series of APIs for interaction with OBS and is used for managing and operating resources, such as buckets and objects, stored in OBS. To use OBS iOS SDK to send a request to OBS, you need to initialize an instance of OBSClient and modify the default configurations in OBSServiceConfiguration based on actual needs.
Sample code for creating an instance of OBSClient using permanent access keys (AK/SK):
NSString *endPoint = @"your-endpoint"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables AccessKeyID and SecretAccessKey. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. char* ak_env = getenv("AccessKeyID"); char* sk_env = getenv("SecretAccessKey"); NSString *AK = [NSString stringWithUTF8String:ak_env]; NSString *SK = [NSString stringWithUTF8String:sk_env]; // Initialize identity authentication. OBSStaticCredentialProvider *credentialProvider = [[OBSStaticCredentialProvider alloc] initWithAccessKey:AK secretKey:SK]; // Initialize service configuration. OBSServiceConfiguration *conf = [[OBSServiceConfiguration alloc] initWithURLString:endPoint credentialProvider:credentialProvider]; // Initialize an instance of OBSClient. OBSClient *client = [[OBSClient alloc] initWithConfiguration:conf];
Sample code for creating an instance of OBSClient using temporary access keys (AK/SK and security token):
NSString *endPoint = @"your-endpoint"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables AccessKeyID and SecretAccessKey. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. char* ak_env = getenv("AccessKeyID"); char* sk_env = getenv("SecretAccessKey"); NSString *AK = [NSString stringWithUTF8String:ak_env]; NSString *SK = [NSString stringWithUTF8String:sk_env]; // Initialize identity authentication. OBSStaticCredentialProvider *credentialProvider = [[OBSStaticCredentialProvider alloc] initWithAccessKey:AK secretKey:SK]; // securityToken char* securityToken_env = getenv("SecurityToken"); NSString *SecurityToken = [NSString stringWithUTF8String:securityToken_env]; credentialProvider.securityToken = SecurityToken; // Initialize service configuration. OBSServiceConfiguration *conf = [[OBSServiceConfiguration alloc] initWithURLString:endPoint credentialProvider:credentialProvider]; // Initialize an instance of OBSClient. OBSClient *client = [[OBSClient alloc] initWithConfiguration:conf];

Currently, when multiple resumable upload tasks need to be executed concurrently, an independent instance of OBSClient needs to be initialized for each upload task to process requests.
Sample code for creating an OBSClient instance using a user-defined domain name:
NSString *endPoint = @"your-custom-domain"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables AccessKeyID and SecretAccessKey. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. char* ak_env = getenv("AccessKeyID"); char* sk_env = getenv("SecretAccessKey"); NSString *AK = [NSString stringWithUTF8String:ak_env]; NSString *SK = [NSString stringWithUTF8String:sk_env]; // Initialize identity authentication. OBSStaticCredentialProvider *credentialProvider = [[OBSStaticCredentialProvider alloc] initWithAccessKey:AK secretKey:SK]; // Initialize service configuration. OBSServiceConfiguration *conf = [[OBSServiceConfiguration alloc] initWithURLString:endPoint credentialProvider:credentialProvider]; // Set to use self-defined domain name to access OBS. conf.defaultDomainMode = OBSDomainModeCustom; // Initialize an instance of OBSClient. OBSClient *client = [[OBSClient alloc] initWithConfiguration:conf];
To use a user-defined domain name to access OBS, set OBSServiceConfiguration.defaultDomainMode to OBSDomainModeCustom, and set endPoint to your user-defined domain name.
Configuring a User-Defined Domain Name
To access an OBS bucket using a user-defined domain name, you need to first bind the user-defined domain name you will use to the domain name of the bucket to access. For details, see User-Defined Domain Name Introduction and User-Defined Domain Name Configuration.
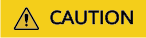
If a user-defined domain name is configured as an acceleration domain name with CDN, you need to complete additional settings on CDN to enable this acceleration domain name to be used to access OBS.
The example shows how to configure the user-defined domain name with CDN on Huawei Cloud.
- Log in to the Huawei Cloud CDN console and select Domains from the navigation pane on the left. You can view all configured CDN domain names.
- Configure the origin server. Click the user-defined domain name to be used to go to the configuration page. Modify the origin server. Set Type of Primary Origin Server to Domain name, and set Origin Server to the domain name of the OBS bucket to be accessed.
- Set the retrieval host to the acceleration domain name, that is, your user-defined domain name. Otherwise, the retrieval authentication may fail.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot