Deleting Versioning Objects
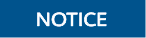
If you have any questions during development, post them on the Issues page of GitHub.
Deleting a Single Versioning Object
You can call delete_object to pass the version ID (version_id) to copy a versioning object. Sample code is as follows:
static void test_delete_object_version() { obs_status ret_status = OBS_STATUS_BUTT; // Create and initialize object information. obs_object_info object_info; memset(&object_info, 0, sizeof(obs_object_info)); object_info.key = key; object_info.version_id = version_id; // Create and initialize option. obs_options option; init_obs_options(&option); option.bucket_options.host_name = "<your-endpoint>"; option.bucket_options.bucket_name = "<Your bucketname>"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. option.bucket_options.access_key = getenv("ACCESS_KEY_ID"); option.bucket_options.secret_access_key = getenv("SECRET_ACCESS_KEY"); // Set response callback function. obs_response_handler resqonseHandler = { &response_properties_callback, &response_complete_callback }; // Delete an object. delete_object(&option,&object_info,&resqonseHandler, &ret_status); if (OBS_STATUS_OK == ret_status) { printf("delete object successfully. \n"); } else { printf("delete object failed(%s).\n", obs_get_status_name(ret_status)); } }
Deleting Versioning Objects in a Batch
You can call batch_delete_objects to pass the version ID (version_id) of each to-be-deleted object to batch delete them. Sample code is as follows:
static void test_batch_delete_object_version() { obs_status ret_status = OBS_STATUS_BUTT; // Create and initialize option. obs_options option; init_obs_options(&option); option.bucket_options.host_name = "<your-endpoint>"; option.bucket_options.bucket_name = "<Your bucketname>"; // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. option.bucket_options.access_key = getenv("ACCESS_KEY_ID"); option.bucket_options.secret_access_key = getenv("SECRET_ACCESS_KEY"); // Initialize information of the objects to be deleted. obs_object_info objectinfo[100]; objectinfo[0].key = "obj1"; objectinfo[0].version_id = "versionid1"; objectinfo[1].key = "obj2"; objectinfo[1].version_id = "versionid2"; obs_delete_object_info delobj; memset_s(&delobj,sizeof(obs_delete_object_info),0,sizeof(obs_delete_object_info)); delobj.keys_number = 2; // Set response callback function. obs_delete_object_handler handler = { {&response_properties_callback, &response_complete_callback}, &delete_objects_data_callback }; // Delete objects in batches. batch_delete_objects(&option, objectinfo, &delobj, 0, &handler, &ret_status); if (OBS_STATUS_OK == ret_status) { printf("test batch_delete_objects successfully. \n"); } else { printf("test batch_delete_objects faied(%s).\n", obs_get_status_name(ret_status)); } }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot