Help Center/
Object Storage Service/
SDK Reference/
BrowserJS/
Object Upload/
Performing a File-Based Upload
Updated on 2024-12-03 GMT+08:00
Performing a File-Based Upload
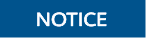
If you have any questions during the development, post them on the Issues page of GitHub. For details about the API parameters and method, see PUT Object.
File-based upload uses local files as the data source of objects. Sample code is as follows:
// Create an instance of ObsClient. var obsClient = new ObsClient({ // Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables AccessKeyID and SecretAccessKey. // The front-end code does not have the process environment variable, so you need to use a module bundler like webpack to define the process variable. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/intl/en-us/usermanual-ca/ca_01_0003.html. access_key_id: process.env.AccessKeyID, secret_access_key: process.env.SecretAccessKey, // Replace the example endpoint with the actual one in your case. server: 'https://obs.ap-southeast-1.myhuaweicloud.com' }); obsClient.putObject({ Bucket: 'bucketname', Key: 'objectname', SourceFile: document.getElementById('input-file').files[0] }, function (err, result) { if(err){ console.error('Error-->' + err); }else{ console.log('Status-->' + result.CommonMsg.Status); } });
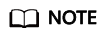
- Use the SourceFile parameter to specify the to-be-uploaded file (a File or Blob object). For example, on an HTML page, use an input tag whose type is file to specify the to-be-uploaded file: <input type="file" id="input-file"/>.
- The SourceFile parameter and the Body parameter cannot be used together.
- The content to be uploaded cannot exceed 5 GB.
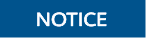
- The browser you use must support the window.File feature. Otherwise, files cannot be uploaded properly.
- For details about how to solve the problem, see Unable to Upload Files Using a Browser that Does Not Support window.File.
Parent topic: Object Upload
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
The system is busy. Please try again later.
For any further questions, feel free to contact us through the chatbot.
Chatbot