Frequently-used Frameworks
This section describes frequently-used AI frameworks supported by ModelArts and how to use these frameworks to compile training code for creating training jobs.
Frequently-used AI Frameworks for Training Management
ModelArts supports the following AI engines and versions.
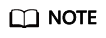
- MoXing is a distributed training acceleration framework developed by the ModelArts team. It is built on the open-source deep learning engines TensorFlow, MXNet, PyTorch, and Keras. If you use MoXing to compile a training script, select the corresponding AI engine and version based on your selected API when you create a training job.
Developing Training Code Using Frequently-used Frameworks
When creating a training job using a common framework, set the code directory, boot file, input path, and output path. These settings enable the interaction between you and ModelArts.
- Code directory
Specify the code directory in the OBS bucket and upload training data such as training code, dependency installation packages, or pre-generated model to the directory. After you create the training job, ModelArts downloads the code directory and its subdirectories to the container.
- Boot file
The boot file in the code directory is used to start the training. Only Python boot files are supported.
- OBS path for storing training data
Do not store training data in the code directory. When the training job starts, the data stored in the code directory will be downloaded to the backend. A large amount of training data may lead to a download failure.
After the training job is started, ModelArts mounts a disk to the /cache directory. You can use this directory to store temporary files. For details about the size of the /cache directory, see What Are Sizes of the /cache Directories for Different Resource Specifications in the Training Environment?
You must upload the training data to another OBS path rather than the code directory and parse data_url to download the training data to the /cache directory. Ensure that you have the read permission to the OBS bucket.
- Output path
You are advised to set an empty directory as the training output path. In the training code, you must parse train_url to upload the training output to the output path. Ensure that you have the write and read permissions to the OBS bucket.
When you use a frequently-used framework to create a training job, develop training code. The following figure shows the process of developing training code.
- (Optional) Introduce dependencies.
If your model references other dependencies, place the corresponding file or installation package in Code Directory you set during training job creation.
Figure 1 Selecting a frequently-used framework and specifying the model boot file - Parse mandatory parameters data_url and train_url.
When using a frequently-used framework to create a training job, set job parameters on the page for creating a training job.
data_url: Training data is mandatory for developing training code. When creating a training job, set Data Source. In the training code, data_url indicates the path of the data source.
train_url: After model training is complete, the trained model and the output information must be stored in an OBS path. When creating a training job, set Training Output Path. In the training code, train_url indicates the OBS path specified for Training Output Path.
Figure 2 Job parametersIn the training code, data_url and train_url must be parsed. Use the following parameter parsing methods for ModelArts:
1 2 3 4 5 6 7 8 9 10
import argparse # Create a parsing task. parser = argparse.ArgumentParser(description="train mnist", formatter_class=argparse.ArgumentDefaultsHelpFormatter) # Add parameters. parser.add_argument('--train_url', type=str, default='obs://obs-test/ckpt/mnist', help='the path model saved') parser.add_argument('--data_url', type=str, default='obs://obs-test/data/', help='the training data') # Parse the parameters. args, unkown = parser.parse_known_args()
- Import training data from data_url.
Training data is stored in data_url. Use the MoXing API to download the data to the cache directory.
1 2
import moxing as mox mox.file.copy_parallel(args.data_url, "/cache")
- Compile training code and save the model.
Training code and the code for saving the model are closely related to the AI engine you use. The following uses the TensorFlow framework as an example. In the training code, the TensorFlow API tf.flags.FLAGS is used to receive CLI parameters.
from __future__ import absolute_import from __future__ import division from __future__ import print_function import os import tensorflow as tf from tensorflow.examples.tutorials.mnist import input_data import moxing as mox tf.flags.DEFINE_integer('max_steps', 1000, 'number of training iterations.') tf.flags.DEFINE_string('data_url', '/home/jnn/nfs/mnist', 'dataset directory.') tf.flags.DEFINE_string('train_url', '/home/jnn/temp/delete', 'saved model directory.') FLAGS = tf.flags.FLAGS def main(*args): mox.file.copy_parallel(FLAGS.data_url, '/cache/data_url') # Train model print('Training model...') mnist = input_data.read_data_sets('/cache/data_url', one_hot=True) sess = tf.InteractiveSession() serialized_tf_example = tf.placeholder(tf.string, name='tf_example') feature_configs = {'x': tf.FixedLenFeature(shape=[784], dtype=tf.float32),} tf_example = tf.parse_example(serialized_tf_example, feature_configs) x = tf.identity(tf_example['x'], name='x') y_ = tf.placeholder('float', shape=[None, 10]) w = tf.Variable(tf.zeros([784, 10])) b = tf.Variable(tf.zeros([10])) sess.run(tf.global_variables_initializer()) y = tf.nn.softmax(tf.matmul(x, w) + b, name='y') cross_entropy = -tf.reduce_sum(y_ * tf.log(y)) tf.summary.scalar('cross_entropy', cross_entropy) train_step = tf.train.GradientDescentOptimizer(0.01).minimize(cross_entropy) correct_prediction = tf.equal(tf.argmax(y, 1), tf.argmax(y_, 1)) accuracy = tf.reduce_mean(tf.cast(correct_prediction, 'float')) tf.summary.scalar('accuracy', accuracy) merged = tf.summary.merge_all() test_writer = tf.summary.FileWriter('/cache/train_url', flush_secs=1) for step in range(FLAGS.max_steps): batch = mnist.train.next_batch(50) train_step.run(feed_dict={x: batch[0], y_: batch[1]}) if step % 10 == 0: summary, acc = sess.run([merged, accuracy], feed_dict={x: mnist.test.images, y_: mnist.test.labels}) test_writer.add_summary(summary, step) print('training accuracy is:', acc) print('Done training!') builder = tf.saved_model.builder.SavedModelBuilder(os.path.join('/cache/train_url', 'model')) tensor_info_x = tf.saved_model.utils.build_tensor_info(x) tensor_info_y = tf.saved_model.utils.build_tensor_info(y) prediction_signature = ( tf.saved_model.signature_def_utils.build_signature_def( inputs={'images': tensor_info_x}, outputs={'scores': tensor_info_y}, method_name=tf.saved_model.signature_constants.PREDICT_METHOD_NAME)) builder.add_meta_graph_and_variables( sess, [tf.saved_model.tag_constants.SERVING], signature_def_map={ 'predict_images': prediction_signature, }, main_op=tf.tables_initializer(), strip_default_attrs=True) builder.save() print('Done exporting!') mox.file.copy_parallel('/cache/train_url', FLAGS.train_url) if __name__ == '__main__': tf.app.run(main=main)
- Export the trained model to train_url.
The training output path is train_url. You are advised to use the MoXing API to export the output from /cache/train_url to train_url.
mox.file.copy_parallel("/cache/train_url", args.train_url)
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot