Configuring the Input and Output Paths of a Workflow
Description
Unified storage is used for workflow directory management. It centrally manages all storage paths of a workflow with these functions:
- Input directory management: When developing a workflow, you can centrally manage all data storage paths. You can store data and configure the root directory based on your own requirements. This function orchestrates directories but does not create them.
- Output directory management: When developing a workflow, you can centrally manage all output paths. You do not need to create output directories. Instead, you only need to configure the root path before the workflow runs and view the output data in the specified directories based on your directory orchestration rules. In addition, multiple executions of the same workflow are output to different directories, isolating data for different executions.
Common Usage
- InputStorage (Path concatenation)
This object is used to centrally manage input directories. The following is an example:
import modelarts.workflow as wf storage = wf.data.InputStorage(name="storage_name", title="title_info", description="description_info") # Only name is mandatory. input_data = wf.data.OBSPath(obs_path = storage.join("directory_path")) # Add a slash (/) after a directory, for example, storage.join("/input/data/"). When a workflow is running, if the root path of the storage object is /root/, the obtained path will be /root/directory_path.
- OutputStorage (Directory creation)
This object is used to centrally manage output directories and ensure that multiple executions of the same workflow are output to different directories. The following is an example:
import modelarts.workflow as wf storage = wf.data.OutputStorage(name="storage_name", title="title_info", description="description_info") # Only name is mandatory. output_path = wf.data.OBSOutputConfig(obs_path = storage.join("directory_path")) # Only a directory can be created but not files. When a workflow is running, if the root path of the storage object is set to /root/, the system will automatically create a relative directory and the obtained path will be /root/Execution ID/directory_path.
Advanced Usage
Storage
This object contains capabilities of InputStorage and OutputStorage and can be flexibly used based on your needs.
Parameter |
Description |
Mandatory |
Data Type |
---|---|---|---|
name |
Name. |
Yes |
str |
title |
If this parameter is left blank, the value of name is used by default. |
No |
str |
description |
Description. |
No |
str |
create_dir |
Whether to create a directory. The default value is False. |
No |
bool |
with_execution_id |
Whether to combine execution_id when a directory is created. The default value is False. This parameter can be set to True only when create_dir is set to True. |
No |
bool |
The following is an example:
- Implementing InputStorage capabilities
import modelarts.workflow as wf # Create a Storage object (with_execution_id=False, create_dir=False). storage = wf.data.Storage(name="storage_name", title="title_info", description="description_info", with_execution_id=False, create_dir=False) input_data = wf.data.OBSPath(obs_path = storage.join("directory_path")) # Add a slash (/) after a directory, for example, storage.join("/input/data/"). When a workflow is running, if the root path of the storage object is /root/, the obtained path will be /root/directory_path.
- Implementing OutputStorage capabilities
import modelarts.workflow as wf # Create a Storage object (with_execution_id=True, create_dir=True). storage = wf.data.Storage(name="storage_name", title="title_info", description="description_info", with_execution_id=True, create_dir=True) output_path = wf.data.OBSOutputConfig(obs_path = storage.join("directory_path")) # Only a directory can be created. When a workflow is running, if the root path of the storage object is set to /root/, the system will automatically create a relative directory and the obtained path will be /root/Execution ID/directory_path.
- Implementing different capabilities of a Storage object through the join method
import modelarts.workflow as wf # Create a Storage object. Assume that the root directory of the Storage object is /root/. storage = wf.data.Storage(name="storage_name", title="title_info", description="description_info", with_execution_id=False, create_dir=False) input_data1 = wf.data.OBSPath(obs_path = storage) # The obtained path is /root/. input_data2 = wf.data.OBSPath(obs_path = storage.join("directory_path")) # The obtained path is /root/directory_path. Ensure that the path exists. output_path1 = wf.data.OBSOutputConfig(obs_path = storage.join(directory="directory_path", with_execution_id=False, create_dir=True)) # The system automatically creates a directory /root/directory_path. output_path2 = wf.data.OBSOutputConfig(obs_path = storage.join(directory="directory_path", with_execution_id=True, create_dir=True)) # The system automatically creates a directory /root/Execution ID/directory_path.
Chain call is supported for Storage.
import modelarts.workflow as wf # Create a base class Storage object. Assume that the root directory of the Storage object is /root/. storage = wf.data.Storage(name="storage_name", title="title_info", description="description_info", with_execution_id=False, create_dir=Fals) input_storage = storage.join("directory_path_1") # The obtained path is /root/directory_path_1. input_storage_next = input_storage.join("directory_path_2") # The obtained path is /root/directory_path_1/directory_path_2.
Examples
Unified storage is mainly used in the job phase. The following code uses a workflow that contains only the training phase as an example.
from modelarts import workflow as wf # Create an InputStorage object. Assume that the root directory of the Storage object is /root/input-data/. input_storage = wf.data.InputStorage(name="input_storage", title="title_info", description="description_info") # Only name is mandatory. # Create an OutputStorage object. Assume that the root directory of the Storage object is /root/output/. output_storage = wf.data.OutputStorage(name="output_storage_name", title="title_info", description="description_info") # Only name is mandatory. # Use JobStep to define a training phase, and set OBS paths for storing inputs and outputs. job_step = wf.steps.JobStep( name="training_job", # Name of a training phase. The name contains a maximum of 64 characters, including only letters, digits, underscores (_), and hyphens (-). It must start with a letter and must be unique in a workflow. title="Image Classification Training", # Title, which defaults to the value of name algorithm=wf.AIGalleryAlgorithm(subscription_id="subscription_ID", item_version_id="item_version_ID"), # Algorithm used for training. In this example, an algorithm subscribed to from AI Gallery is used. inputs=[ wf.steps.JobInput(name="data_url_1", data=wf.data.OBSPath(obs_path = input_storage.join("/dataset1/new.manifest"))), # The obtained path is /root/input-data/dataset1/new.manifest. wf.steps.JobInput(name="data_url_1", data=wf.data.OBSPath(obs_path = input_storage.join("/dataset1/new.manifest"))), # The obtained path is /root/input-data/dataset1/new.manifest. ], outputs=wf.steps.JobOutput(name="train_url", obs_config=wf.data.OBSOutputConfig(obs_path=output_storage.join("/model/"))), # The training output path is /root/output/Execution ID/model/. spec=wf.steps.JobSpec( resource=wf.steps.JobResource( flavor=wf.Placeholder(name="train_flavor", placeholder_type=wf.PlaceholderType.JSON, description="Training flavor") ), log_export_path=wf.steps.job_step.LogExportPath(obs_url=output_storage.join("/logs/")) # The log output path is /root/output/Execution ID/logs/. )# Training flavors ) # Define a workflow that contains only the job phase. workflow = wf.Workflow( name="test-workflow", desc="this is a test workflow", steps=[job_step], storages=[input_storage, output_storage] # Add Storage objects used in this workflow. )
Configuring Root Paths in the Development State
Use the run method of the workflow object, and input root paths in the text box that is displayed when the workflow starts to run.

You must enter a valid path. If the path does not exist, an error will occur. The path format must be /Bucket name/Folder path/.
Configuring Root Paths in the Running State
Use the release method of the workflow object to release the workflow to the running state. On the ModelArts console, go to the Workflow page, find the target workflow, and configure root paths.
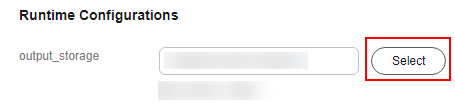
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot