Examples
There are six scenarios:
- Registering models output by JobStep
- Registering a model using OBS data
- Registering a model using a template
- Registering a model using a custom image
- Registering a model using a custom image and OBS
- Registering a model using a subscribed model and OBS
Registering a Model From a Training Job (Model Source: JobStep Output)
import modelarts.workflow as wf # Create an OutputStorage object to centrally manage training output directories. storage = wf.data.OutputStorage(name="storage_name", title="title_info", description="description_info") # Only name is mandatory. # Define the input dataset object. dataset = wf.data.DatasetPlaceholder(name="input_dataset") # Use JobStep to define a training phase. Use a dataset as the input, and use OBS to store the output. job_step = wf.steps.JobStep( name="training_job", # Name of a training phase. The name contains a maximum of 64 characters, including only letters, digits, underscores (_), and hyphens (-). It must start with a letter and must be unique in a workflow. title="Image classification training", # Title, which defaults to the value of name. algorithm=wf.AIGalleryAlgorithm( subscription_id="subscription_id", # algorithm subscription ID. You can also enter the version number. item_version_id="item_version_id", # Algorithm version ID. You can also enter the version number instead. parameters=[ wf.AlgorithmParameters( name="parameter_name", value=wf.Placeholder(name="parameter_name", placeholder_type=wf.PlaceholderType.STR, default="fake_value",description="description_info") ) # Algorithm hyperparameters are represented using placeholders, which can be integer, bool, float, or string. ] ), # Algorithm used for training. An algorithm subscribed to in AI Gallery is used in this example. If the value of an algorithm hyperparameter does not need to be changed, you do not need to configure the hyperparameter in parameters. Hyperparameter values will be automatically filled. inputs=wf.steps.JobInput(name="data_url", data=dataset), # JobStep inputs are configured when the workflow is running. You can also use wf.data.Dataset(dataset_name="fake_dataset_name", version_name="fake_version_name") for the data field. outputs=wf.steps.JobOutput(name="train_url", obs_config=wf.data.OBSOutputConfig(obs_path=storage.join("directory_path"))), # JobStep outputs spec=wf.steps.JobSpec( resource=wf.steps.JobResource( flavor=wf.Placeholder(name="train_flavor", placeholder_type=wf.PlaceholderType.JSON, description="Training flavor") ) )# Training flavors ) # Define a model registration phase using ModelStep. The output of JobStep is used as the input of ModelStep. # Define model name parameters. model_name = wf.Placeholder(name="placeholder_name", placeholder_type=wf.PlaceholderType.STR) model_registration = wf.steps.ModelStep( name="model_registration", # Name of the model registration phase. The name contains a maximum of 64 characters, including only letters, digits, underscores (_), and hyphens (-). It must start with a letter and must be unique in a workflow. title="Model registration", # Title inputs=wf.steps.ModelInput(name='model_input', data=job_step.outputs["train_url"].as_input()), # The output of JobStep is used as the input of ModelStep. outputs=wf.steps.ModelOutput(name='model_output',model_config=wf.steps.ModelConfig(model_name=model_name, model_type="TensorFlow")), # ModelStep outputs depend_steps=job_step # Dependent job phase ) # job_step is an instance object of wf.steps.JobStep and train_url is the value of the name field of wf.steps.JobOutput. workflow = wf.Workflow( name="model-step-demo", desc="this is a demo workflow", steps=[job_step, model_registration], storages=[storage] )
Registering a Model From a Training Job (Model Source: A Trained Model Stored in OBS)
import modelarts.workflow as wf # Define a model registration phase using ModelStep. The input is from OBS. # Define the OBS data. obs = wf.data.OBSPlaceholder(name = "obs_placeholder_name", object_type = "directory" ) # object_type must be file or directory. # Define model name parameters. model_name = wf.Placeholder(name="placeholder_name", placeholder_type=wf.PlaceholderType.STR) model_registration = wf.steps.ModelStep( name="model_registration", # Name of the model registration phase. The name contains a maximum of 64 characters, including only letters, digits, underscores (_), and hyphens (-). It must start with a letter and must be unique in a workflow. title="Model registration", # Title inputs=wf.steps.ModelInput(name='model_input', data=obs), # ModelStep inputs are configured when the workflow is running. You can also use wf.data.OBSPath(obs_path="fake_obs_path") for the data field. outputs=wf.steps.ModelOutput(name='model_output',model_config=wf.steps.ModelConfig(model_name=model_name, model_type="TensorFlow"))# ModelStep outputs ) workflow = wf.Workflow( name="model-step-demo", desc="this is a demo workflow", steps=[model_registration] )
Registering a Model Using a Template
import modelarts.workflow as wf # Define a model registration phase using ModelStep. Register a model using a preset template. # Define a preset template object. Fields in the template object can be represented by placeholders. template = wf.steps.Template( template_id="fake_template_id", infer_format="fake_infer_format", template_inputs=[ wf.steps.TemplateInputs( input_id="fake_input_id", input="fake_input_file" ) ] ) # Define model name parameters. model_name = wf.Placeholder(name="placeholder_name", placeholder_type=wf.PlaceholderType.STR) model_registration = wf.steps.ModelStep( name="model_registration", # Name of the model registration phase. The name contains a maximum of 64 characters, including only letters, digits, underscores (_), and hyphens (-). It must start with a letter and must be unique in a workflow. title="Model registration", # Title outputs=wf.steps.ModelOutput( name='model_output', model_config=wf.steps.ModelConfig( model_name=model_name, model_type="Template", template=template ) ) # ModelStep outputs ) workflow = wf.Workflow( name="model-step-demo", desc="this is a demo workflow", steps=[model_registration] )
Registering a Model From a Custom Image
import modelarts.workflow as wf # Define a model registration phase using ModelStep. The input is from the URL of a custom image. # Define the image data. swr = wf.data.SWRImagePlaceholder(name="placeholder_name") # Define model name parameters. model_name = wf.Placeholder(name="placeholder_name", placeholder_type=wf.PlaceholderType.STR) model_registration = wf.steps.ModelStep( name="model_registration", # Name of the model registration phase. The name contains a maximum of 64 characters, including only letters, digits, underscores (_), and hyphens (-). It must start with a letter and must be unique in a workflow. title="Model registration", # Title inputs=wf.steps.ModelInput(name="input",data=swr), # ModelStep inputs are configured when the workflow is running. You can also use wf.data.SWRImage(swr_path="fake_path") for the data field. outputs=wf.steps.ModelOutput(name='model_output',model_config=wf.steps.ModelConfig(model_name=model_name, model_type="TensorFlow"))# ModelStep outputs ) workflow = wf.Workflow( name="model-step-demo", desc="this is a demo workflow", steps=[model_registration] )
Registering a Model Using a Custom Image and OBS
import modelarts.workflow as wf # Define a model registration phase using ModelStep. The input is from the URL of a custom image. # Define the image data. swr = wf.data.SWRImagePlaceholder(name="placeholder_name") # Define OBS model data. model_obs = wf.data.OBSPlaceholder(name = "obs_placeholder_name", object_type = "directory" ) # object_type must be file or directory. # Define model name parameters. model_name = wf.Placeholder(name="placeholder_name", placeholder_type=wf.PlaceholderType.STR) model_registration = wf.steps.ModelStep( name="model_registration", # Name of the model registration phase. The name contains a maximum of 64 characters, including only letters, digits, underscores (_), and hyphens (-). It must start with a letter and must be unique in a workflow. title="Model registration", # Title inputs=[ wf.steps.ModelInput(name="input",data=swr), # ModelStep inputs are configured when the workflow is running. You can also use wf.data.SWRImage(swr_path="fake_path") for the data field. wf.steps.ModelInput(name="input",data=model_obs) # ModelStep inputs are configured when the workflow is running. You can also use wf.data.OBSPath(obs_path="fake_obs_path") for the data field. ], outputs=wf.steps.ModelOutput( name='model_output', model_config=wf.steps.ModelConfig( model_name=model_name, model_type="Custom", dynamic_load_mode="Single" ) ) # ModelStep outputs ) workflow = wf.Workflow( name="model-step-demo", desc="this is a demo orkflow", steps=[model_registration] )
Registering a Model Using a Subscribed Model and OBS
This mode is similar to the custom image + OBS mode, except that you obtain a custom image from a subscribed model.
Example:
import modelarts.workflow as wf # Define the subscribed model object. base_model = wf.data.GalleryModel(subscription_id="fake_subscription_id", version_num="fake_version") # Model subscribed to from AI Gallery, generally published by a developer # Define OBS model data. model_obs = wf.data.OBSPlaceholder(name = "obs_placeholder_name", object_type = "directory" ) # object_type must be file or directory. # Define model name parameters. model_name = wf.Placeholder(name="placeholder_name", placeholder_type=wf.PlaceholderType.STR) model_registration = wf.steps.ModelStep( name="model_registration", # Name of the model registration phase. The name contains a maximum of 64 characters, including only letters, digits, underscores (_), and hyphens (-). It must start with a letter and must be unique in a workflow. title="Model registration", # Title inputs=[ wf.steps.ModelInput(name="input",data=base_model) # Use a subscribed model as the ModelStep input. wf.steps.ModelInput(name="input",data=model_obs) # ModelStep inputs are configured when the workflow is running. You can also use wf.data.OBSPath(obs_path="fake_obs_path") for the data field. ], outputs=wf.steps.ModelOutput( name='model_output', model_config=wf.steps.ModelConfig( model_name=model_name, model_type="Custom", dynamic_load_mode="Single" ) ) # ModelStep outputs ) workflow = wf.Workflow( name="model-step-demo", desc="this is a demo workflow", steps=[model_registration] )
In the preceding example, the system automatically obtains the custom image from the subscribed model and registers and generates a model based on the entered OBS model path. model_obs can be replaced with the dynamic output of JobStep.
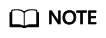
The value of model_type can be TensorFlow, MXNet, Caffe, Spark_MLlib, Scikit_Learn, XGBoost, Image, PyTorch, Template, or Custom.
If model_type is not set for wf.steps.ModelConfig, TensorFlow is used by default.
- If the model type of your workflow does not need to be changed, refer to the preceding examples.
- If the model type of your workflow needs to be changed in multiple executions, write the parameter using placeholders.
model_type = wf.Placeholder(name="placeholder_name", placeholder_type=wf.PlaceholderType.ENUM, default="TensorFlow", enum_list=["TensorFlow", "MXNet", "Caffe", "Spark_MLlib", "Scikit_Learn", "XGBoost", "Image", "PyTorch", "Template", "Custom"], description="Model type")
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot