Data Selection Among Multiple Inputs
Function
This function is only for the scenario where multiple branches are run. When you create a workflow phase, the data input source of the phase is uncertain. The data input source could be the output of any of the phases it depends on. Only after all dependency phases are run, the valid output is automatically selected as the input based on the actual execution situation.
Examples
from modelarts import workflow as wf condition_equal = wf.steps.Condition(condition_type=wf.steps.ConditionTypeEnum.EQ, left=wf.Placeholder(name="is_true", placeholder_type=wf.PlaceholderType.BOOL), right=True) condition_step = wf.steps.ConditionStep( name="condition_step", conditions=[condition_equal], if_then_steps=["training_job_1"], else_then_steps=["training_job_2"], ) # Create an OutputStorage object to centrally manage training output directories. storage = wf.data.OutputStorage(name="storage_name", title="title_info", description="description_info") # Only name is mandatory. # Define the input OBS object. obs_data = wf.data.OBSPlaceholder(name="obs_placeholder_name", object_type="directory") # Use JobStep to define a training phase, and use OBS to store the output. job_step_1 = wf.steps.JobStep( name="training_job_1", # Name of a training phase. The name contains a maximum of 64 characters, including only letters, digits, underscores (_), and hyphens (-). It must start with a letter and must be unique in a workflow. title="Image classification training", # Title, which defaults to the value of name. algorithm=wf.AIGalleryAlgorithm( subscription_id="subscription_id", # Subscription ID of the subscribed algorithm item_version_id="item_version_id", # Algorithm version ID. You can also enter the version number instead. parameters=[] ), # Algorithm used for training. An algorithm subscribed to in AI Gallery is used in this example. If the value of an algorithm hyperparameter does not need to be changed, you do not need to configure the hyperparameter in parameters. Hyperparameter values will be automatically filled. inputs=wf.steps.JobInput(name="data_url", data=obs_data), # JobStep input is configured when the workflow is running. You can also use data=wf.data.OBSPath(obs_path="fake_obs_path") for the data field. outputs=wf.steps.JobOutput(name="train_url", obs_config=wf.data.OBSOutputConfig(obs_path=storage.join("directory_path"))), # JobStep output spec=wf.steps.JobSpec( resource=wf.steps.JobResource( flavor=wf.Placeholder(name="train_flavor", placeholder_type=wf.PlaceholderType.JSON, description="Training flavor") ) ), # Training flavors depend_steps=[condition_step] ) # Use JobStep to define a training phase, and use OBS to store the output. job_step_2 = wf.steps.JobStep( name="training_job_2", # Name of a training phase. The name contains a maximum of 64 characters, including only letters, digits, underscores (_), and hyphens (-). It must start with a letter and must be unique in a workflow. title="Image classification training", # Title, which defaults to the value of name. algorithm=wf.AIGalleryAlgorithm( subscription_id="subscription_id", # Subscription ID of the subscribed algorithm item_version_id="item_version_id", # Algorithm version ID. You can also enter the version number instead. parameters=[] ), # Algorithm used for training. An algorithm subscribed to in AI Gallery is used in this example. If the value of an algorithm hyperparameter does not need to be changed, you do not need to configure the hyperparameter in parameters. Hyperparameter values will be automatically filled. inputs=wf.steps.JobInput(name="data_url", data=obs_data), # JobStep input is configured when the workflow is running. You can also use data=wf.data.OBSPath(obs_path="fake_obs_path") for the data field. outputs=wf.steps.JobOutput(name="train_url", obs_config=wf.data.OBSOutputConfig(obs_path=storage.join("directory_path"))), # JobStep output spec=wf.steps.JobSpec( resource=wf.steps.JobResource( flavor=wf.Placeholder(name="train_flavor", placeholder_type=wf.PlaceholderType.JSON, description="Training flavor") ) ), # Training flavors depend_steps=[condition_step] ) # Define model name parameters. model_name = wf.Placeholder(name="placeholder_name", placeholder_type=wf.PlaceholderType.STR) model_step = wf.steps.ModelStep( name="model_registration", # Name of the model registration phase. The name contains a maximum of 64 characters, including only letters, digits, underscores (_), and hyphens (-). It must start with a letter and must be unique in a workflow. title="Model registration", # Title inputs=wf.steps.ModelInput(name='model_input', data=wf.data.DataConsumptionSelector(data_list=[job_step_1.outputs["train_url"].as_input(), job_step_2.outputs["train_url"].as_input()])), # Select the output of job_step_1 or job_step_2 as the input. outputs=wf.steps.ModelOutput(name='model_output', model_config=wf.steps.ModelConfig(model_name=model_name, model_type="TensorFlow")), # ModelStep outputs depend_steps=[job_step_1, job_step_2] # Preceding job phase )# job_step is an instance object of wf.steps.JobStep and train_url is the value of the name field of wf.steps.JobOutput. workflow = wf.Workflow(name="data-select-demo", desc="this is a test workflow", steps=[condition_step, job_step_1, job_step_2, model_step], storages=storage )
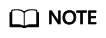
The workflow in this example has two parallel branches, but only one branch runs at a time, depending on the configuration of condition_step. The input source of model_step is either job_step_1 or job_step_2's output. If job_step_1 runs and job_step_2 is skipped, model_step uses job_step_1's output as input, and vice versa.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot