Connecting to the Database (Using SSL)
The Go driver supports SSL connections to the database. After the SSL mode is enabled, if the Go driver connects to the database server in SSL mode, the Go driver uses the standard TLS 1.3 protocol by default, and the TLS version must be 1.2 or later. This section describes how applications configure the client in SSL mode through the Go driver. For details about how to configure the server, contact the administrator. To use the method described in this section, you must have the server certificate, client certificate, and private key files. For details on how to obtain these files, see related documents and commands of OpenSSL.
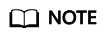
In SSL-based certificate authentication mode, you do not need to specify the user password in the connection string.
Configuring the Client
Upload the client.key, client.crt, and cacert.pem files generated during server configuration to the client. For details about server configuration, contact the administrator.
Example 1:
package main // Set the dependency package based on the dependency package path in the environment. import ( "database/sql" "fmt" _ "gitee.com/opengauss/openGauss-connector-go-pq" "log" ) // Mutual authentication is used as an example. In this example, the username and password are stored in environment variables. Before running this example, set environment variables in the local environment (set the environment variable name based on the actual situation). func main() { hostip := os.Getenv("GOHOSTIP") // GOHOSTIP indicates the IP address written into the environment variable. port := os.Getenv("GOPORT") // GOPORT indicates the port number written into the environment variable. usrname := os.Getenv("GOUSRNAME") // GOUSRNAME indicates the username for writing environment variables. passwd := os.Getenv("GOPASSWD") // GOPASSWDW indicates the user password written into the environment variable. sslpasswd := os.Getenv("GOSSLPASSWD") // GOSSLPASSWDW indicates the passphrase written into the environment variable. dsnStr := "host=" + hostip + " port=" + port + " user=" + usrname + " password=" + passwd + " dbname=gaussdb sslcert=certs/client.crt sslkey=certs/client.key sslpassword=" + sslpasswd parameters := []string{ " sslmode=require", " sslmode=verify-ca sslrootcert=certs/cacert.pem", } for _, param := range parameters { db, err := sql.Open("gaussdb", dsnStr+param) if err != nil { log.Fatal(err) } var f1 int err = db.QueryRow("select 1").Scan(&f1) if err != nil { log.Fatal(err) } else { fmt.Printf("RESULT: select 1: %d\n", f1) } db.Close() } }
Example 2:
package main // Set the dependency package based on the dependency package path in the environment. import ( "database/sql" _ "gitee.com/opengauss/openGauss-connector-go-pq" "log" "strings" ) // For example, verify sslpassword. In this example, the username and password are stored in environment variables. Before running this example, set environment variables in the local environment (set the environment variable name based on the actual situation). func main() { hostip := os.Getenv("GOHOSTIP") // GOHOSTIP indicates the IP address written into the environment variable. port := os.Getenv("GOPORT") // GOPORT indicates the port number written into the environment variable. usrname := os.Getenv("GOUSRNAME") // GOUSRNAME indicates the username for writing environment variables. passwd := os.Getenv("GOPASSWD") // GOPASSWDW indicates the user password written into the environment variable. dsnStr := "host=" + hostip + " port=" + port + " user=" + usrname + " password=" + passwd + " dbname=gaussdb" sslpasswd := os.Getenv("GOSSLPASSWD") // GOSSLPASSWDW indicates the passphrase written into the environment variable. connStrs := []string{ " sslmode=verify-ca sslcert=certs/client_rsa.crt sslkey=certs/client_rsa.key sslpassword=" + sslpasswd + " sslrootcert=certs/cacert_rsa.pem", " sslmode=verify-ca sslcert=certs/client_ecdsa.crt sslkey=certs/client_ecdsa.key sslpassword=" + sslpasswd + " sslrootcert=certs/cacert_ecdsa.pem", } for _, connStr := range connStrs { db, err := sql.Open("gaussdb", dsnStr+connStr) if err != nil { log.Fatal(err) } var f1 int err = db.QueryRow("select 1").Scan(&f1) if err != nil { if !strings.HasPrefix(err.Error(), "connect failed.") { log.Fatal(err) } } db.Close() } }
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot