Variable Definition Statements
This section describes the declaration of variables in the PL/SQL and the scope of this variable in codes.
Variable Declaration
Figure 1 shows the variable declaration syntax.
The syntax is described as follows:
- variable_name indicates the name of a variable.
- type indicates the type of a variable.
- value indicates the initial value of the variable. (If the initial value is not given, NULL is taken as the initial value.) value can also be an expression.
Examples
1 2 3 4 5 6 |
gaussdb=# DECLARE emp_id INTEGER := 7788; -- Define a variable and assign a value to it. BEGIN emp_id := 5*7784; -- Assign a value to the variable. END; / |
In addition to the declaration of basic variable types, %TYPE and %ROWTYPE can be used to declare variables related to table columns or table structures.
%TYPE Attribute
%TYPE declares a variable to be of the same data type as a previously declared variable (for example, a column in a table). For example, if you want to define the my_name variable whose data type is the same as the data type of firstname in employee, you can define the variable as follows:
my_name employee.firstname%TYPE -- Example DROP TABLE IF EXISTS employee; CREATE TABLE employee(firstname varchar,secondname varchar); DECLARE my_name employee.firstname%TYPE; BEGIN my_name = 'abc'; DBE_OUTPUT.PRINT_LINE(my_name); END; /
In this way, you do not need to know the data type of firstname in employee. Even if the data type of firstname changes, you do not need to change the data type of my_name.
%ROWTYPE Attribute
%ROWTYPE declares data types of a set of data. It stores a row of table data or results fetched from a cursor. For example, if you want to define a set of data with the same column names and column data types as the employee table, you can define the data as follows:
my_employee employee%ROWTYPE DROP TABLE IF EXISTS employee; CREATE TABLE employee(firstname varchar,secondname varchar); DECLARE my_employee employee%ROWTYPE; BEGIN my_employee.firstname := 'ab1'; my_employee.secondname := 'ab2'; DBE_OUTPUT.PRINT_LINE(my_employee.firstname); DBE_OUTPUT.PRINT_LINE(my_employee.secondname); END; /
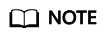
- In the environment with multiple CNs, the %ROWTYPE and %TYPE attributes of the temporary table cannot be declared in a stored procedure. The temporary table is valid only in the current session. During compilation, other CNs cannot view the temporary table of the current CN. Therefore, if there are multiple CNs, the system displays a message indicating that the temporary table does not exist.
- %TYPE cannot reference the cursor variables of a column type.
- View%ROWTYPE or SCHEMA.view%ROWTYPE cannot be used as the input/output parameter type.
- Table/View.column.column%TYPE or SCHEMA.Table/View.column.column%TYPE cannot be nested with one or more layers as variable types or input/output parameter type.
Scope of a Variable
The scope of a variable indicates the accessibility and availability of the variable in code block. In other words, a variable takes effect only within its scope.
- To define a function scope, a variable must declare and create a BEGIN-END block in the declaration section. The necessity of such declaration is also determined by block structure, which requires that a variable has different scopes and lifetime during a process.
- A variable can be defined multiple times in different scopes, and inner definition can cover outer one.
- A variable defined in an outer block can also be used in a nested block. However, the outer block cannot access variables in the nested block.
Example
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
gaussdb=# DECLARE emp_id INTEGER :=7788; -- Define a variable and assign a value to it. outer_var INTEGER :=6688; -- Define a variable and assign a value to it. BEGIN DECLARE emp_id INTEGER :=7799; -- Define a variable and assign a value to it. inner_var INTEGER :=6688; -- Define a variable and assign a value to it. BEGIN dbe_output.print_line('inner emp_id ='||emp_id); -- Display the value 7799. dbe_output.print_line('outer_var ='||outer_var); -- Reference a variable of an outer block. END; dbe_output.print_line('outer emp_id ='||emp_id); -- Display the value 7788. END; / |
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot