Functions Supported by Arrays
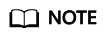
- The following describes the behavior differences of array functions before and after the GUC parameter varray_compat is enabled:
- If the count, extend, trim, delete, first, last, next, or prior function is applied to an uninitialized array, that is, the array is NULL, the "Reference to uninitialized collection" error is reported after the parameter is enabled. If the parameter is disabled, no error is reported.
- For the extend(count) and extend(count, idx) functions, if the sum of count and the number of existing elements in the array exceeds the defined array size, the "Subscript outside of limit" error is reported after the parameter is enabled. If the parameter is disabled, no error is reported.
- For the extend(count, idx) function, if the index idx is invalid, the "Subscript outside of limit" or "Subscript beyond count" error is reported after the parameter is enabled.
- For the trim(n) function, if n is greater than the number of array elements, the "Subscript beyond count" error is reported after the parameter is enabled. If the parameter is disabled, no error is reported.
- For the delete(idx) function, if the index idx is invalid, the "Subscript outside of limit" or "Subscript beyond count" error is reported after the parameter is enabled. If the parameter is disabled, the original array is returned.
- The following is an example in A-compatible mode. In the function definition description, the content in [] is optional. For example, count[()] can be written as count or count().
- If the error "Cannot change the guc status while in the same session" is reported during the execution of the following example, you need to switch the session and execute the example again.
- In an inner expression, functions of the array type cannot be called in nesting mode.
- extend[([count])]
Parameter: count is of the int4 type.
Return value: No value is returned.
Description: Extends one or count elements at the end of a varray variable. The default value is NULL.
Example 1: The extend is used to extend one element. The default value is NULL.
gaussdb=# set behavior_compat_options = ''; SET gaussdb=# declare gaussdb-# type array_integer is varray(10) of integer; gaussdb-# arrint array_integer := array_integer(); gaussdb-# begin gaussdb$# dbe_output.print_line(arrint.count); gaussdb$# arrint.extend; gaussdb$# dbe_output.print_line(arrint.count); gaussdb$# end; gaussdb$# / 0 1 ANONYMOUS BLOCK EXECUTE
Example 2: The extend(count) is used to extend the count elements. The default value is NULL.
gaussdb=# set behavior_compat_options = ''; SET gaussdb=# declare gaussdb-# type array_integer is varray(10) of integer; gaussdb-# arrint array_integer := array_integer(); gaussdb-# begin gaussdb$# dbe_output.print_line(arrint.count); gaussdb$# arrint.extend(3); gaussdb$# dbe_output.print_line(arrint.count); gaussdb$# end; gaussdb$# / 0 3 ANONYMOUS BLOCK EXECUTE
Example 3: When the varray_compat parameter is enabled, an error is reported if the array size exceeds the defined size after the extend is used.
gaussdb=# set behavior_compat_options = 'varray_compat'; SET gaussdb=# declare gaussdb-# type array_integer is varray(10) of integer; gaussdb-# arrint array_integer := array_integer(); gaussdb-# begin gaussdb$# dbe_output.print_line(arrint.count); gaussdb$# arrint.extend(3); gaussdb$# dbe_output.print_line(arrint.count); gaussdb$# arrint.extend(8); -- error gaussdb$# end; gaussdb$# / 0 3 ERROR: Subscript outside of limit CONTEXT: PL/SQL function inline_code_block line 8 at assignment
Example 4: When the varray_compat parameter is enabled, an error is reported if the array is not initialized.gaussdb=# set behavior_compat_options = 'varray_compat'; SET gaussdb=# declare gaussdb-# type array_integer is varray(10) of integer; gaussdb-# arrint array_integer; gaussdb-# begin gaussdb$# arrint.extend(3); gaussdb$# end; gaussdb$# / ERROR: Reference to uninitialized collection CONTEXT: PL/pgSQL function inline_code_block line 4 at assignment
- extend(count, idx)
Parameters: idx and count are of the int4 type.
Return value: No value is returned.
Description: Extends count elements at the end of a varray variable, and the value of the extended element is equal to the value of the given idx index element. This function can be used only after a_format_version is set to 10c and a_format_dev_version is set to s1.
Example 1: The extend(count, idx) is used to extend the element at the idx position.
gaussdb=# set a_format_version='10c'; SET gaussdb=# set a_format_dev_version='s1'; SET gaussdb=# set behavior_compat_options = ''; SET gaussdb=# declare gaussdb-# type array_integer is varray(10) of integer; gaussdb-# arrint array_integer := array_integer(1,2); gaussdb-# begin gaussdb$# arrint.extend(2, 1); gaussdb$# dbe_output.print_line(arrint.count); gaussdb$# for i in 1..arrint.count loop gaussdb$# dbe_output.print_line(arrint(i)); gaussdb$# end loop; gaussdb$# end; gaussdb$# / 4 1 2 1 1 ANONYMOUS BLOCK EXECUTE
Example 2: extend(count, idx). If the varray_compat parameter is enabled and idx is out of range, an error is reported.
gaussdb=# set a_format_version='10c'; SET gaussdb=# set a_format_dev_version='s1'; SET gaussdb=# set behavior_compat_options = 'varray_compat'; SET gaussdb=# declare gaussdb-# type array_integer is varray(10) of integer; gaussdb-# arrint array_integer := array_integer(); gaussdb-# begin gaussdb$# arrint.extend(10, 1); -- error index. gaussdb$# end; gaussdb$# / ERROR: Subscript beyond count CONTEXT: PL/SQL function inline_code_block line 5 at assignment
Example 3: extend(count, idx). If the varray_compat parameter is enabled and the array is not initialized, an error is reported.
gaussdb=# set a_format_version='10c'; SET gaussdb=# set a_format_dev_version='s1'; SET gaussdb=# set behavior_compat_options = 'varray_compat'; SET gaussdb=# declare gaussdb-# type array_integer is varray(10) of integer; gaussdb-# arrint array_integer; gaussdb-# begin gaussdb$# arrint.extend(10, 1); gaussdb$# end; gaussdb$# / ERROR: Reference to uninitialized collection CONTEXT: PL/pgSQL function inline_code_block line 4 at assignment
- count[()]
Return value: int4 type.
Description: Returns the number of elements in an array.
Example 1: View the number of initialized array elements.
gaussdb=# set behavior_compat_options = ''; SET gaussdb=# declare gaussdb-# type array_integer is varray(10) of integer; gaussdb-# arrint array_integer; gaussdb-# len int; gaussdb-# begin gaussdb$# arrint := array_integer(1, 2, 3); gaussdb$# len := arrint.count(); gaussdb$# dbe_output.print_line(len); gaussdb$# end; gaussdb$# / 3 ANONYMOUS BLOCK EXECUTE
Example 2: When the varray_compat parameter is enabled, an error is reported if the array is not initialized.
gaussdb=# set behavior_compat_options = 'varray_compat'; SET gaussdb=# declare gaussdb-# type array_integer is varray(10) of integer; gaussdb-# arrint array_integer; gaussdb-# len int; gaussdb-# begin gaussdb$# len := arrint.count(); gaussdb$# dbe_output.print_line(len); gaussdb$# end; gaussdb$# / ERROR: Reference to uninitialized collection CONTEXT: PL/SQL function inline_code_block line 6 at assignment
- trim[(n)]
Parameter: n is of the int4 type.
Return value: No value is returned.
Description: Deletes an element space at the end of the array if there is no parameter. Alternatively, deletes a specified number of element spaces at the end of the array if the parameter n is specified.
Example 1: Delete n elements from an array without enabling the varray_compat parameter.
gaussdb=# set behavior_compat_options = ''; SET gaussdb=# declare gaussdb-# type array_integer is varray(10) of integer; gaussdb-# arrint array_integer := array_integer(1,2,3); gaussdb-# begin gaussdb$# dbe_output.print_line(arrint.count); gaussdb$# arrint.trim(1); gaussdb$# dbe_output.print_line(arrint.count); gaussdb$# end; gaussdb$# / 3 2 ANONYMOUS BLOCK EXECUTE -- If n is greater than the number of array elements, clear all array elements. gaussdb=# declare gaussdb-# type array_integer is varray(10) of integer; gaussdb-# arrint array_integer := array_integer(1,2,3); gaussdb-# begin gaussdb$# dbe_output.print_line(arrint.count); gaussdb$# arrint.trim(4); gaussdb$# dbe_output.print_line(arrint.count); gaussdb$# end; gaussdb$# / 3 0 ANONYMOUS BLOCK EXECUTE
Example 2: Delete n elements from an array (n > Number of array elements) without enabling the varray_compat parameter.
-- If n is greater than the number of array elements, clear all array elements. gaussdb=# set behavior_compat_options = ''; SET gaussdb=# declare gaussdb-# type array_integer is varray(10) of integer; gaussdb-# arrint array_integer := array_integer(1,2,3); gaussdb-# begin gaussdb$# dbe_output.print_line(arrint.count); gaussdb$# arrint.trim(4); gaussdb$# dbe_output.print_line(arrint.count); gaussdb$# end; gaussdb$# / 3 0 ANONYMOUS BLOCK EXECUTE
Example 3: Delete n elements from an array (n > Number of array elements) with the varray_compat parameter enabled.
-- If n is greater than the number of array elements, an error is reported. gaussdb=# set behavior_compat_options = 'varray_compat'; SET gaussdb=# declare gaussdb-# type array_integer is varray(10) of integer; gaussdb-# arrint array_integer := array_integer(1,2,3); gaussdb-# begin gaussdb$# dbe_output.print_line(arrint.count); -- count > 0. gaussdb$# arrint.trim(4); gaussdb$# end; gaussdb$# / 3 ERROR: Subscript beyond count CONTEXT: PL/SQL function inline_code_block line 6 at assignment -- An error is reported when the array is not initialized. gaussdb=# set behavior_compat_options = 'varray_compat'; SET gaussdb=# declare gaussdb-# type array_integer is varray(10) of integer; gaussdb-# arrint array_integer; gaussdb-# begin gaussdb$# arrint.trim(1); gaussdb$# end; gaussdb$# / ERROR: Reference to uninitialized collection CONTEXT: PL/SQL function inline_code_block line 5 at assignment
Example 4: The array is not initialized, and the varray_compat parameter is enabled.
-- An error is reported when the array is not initialized. gaussdb=# set behavior_compat_options = 'varray_compat'; SET gaussdb=# declare gaussdb-# type array_integer is varray(10) of integer; gaussdb-# arrint array_integer; gaussdb-# begin gaussdb$# arrint.trim(1); gaussdb$# end; gaussdb$# / ERROR: Reference to uninitialized collection CONTEXT: PL/SQL function inline_code_block line 5 at assignment
- delete[()]
Return value: No value is returned.
Description: Clears elements in an array.
Example 1: Clear the elements in the array without enabling the varray_compat parameter.
gaussdb=# set behavior_compat_options = ''; SET gaussdb=# declare gaussdb-# type array_integer is varray(10) of integer; gaussdb-# arrint array_integer := array_integer(1,2,3); gaussdb-# begin gaussdb$# dbe_output.print_line(arrint.count); gaussdb$# arrint.delete(); gaussdb$# dbe_output.print_line(arrint.count); gaussdb$# end; gaussdb$# / 3 0 ANONYMOUS BLOCK EXECUTE -- The array is not initialized. gaussdb=# declare gaussdb-# type array_integer is varray(10) of integer; gaussdb-# arrint array_integer; gaussdb-# begin gaussdb$# arrint.delete(); gaussdb$# end; gaussdb$# / ANONYMOUS BLOCK EXECUTE
Example 2: Clear the elements in the array with the varray_compat parameter enabled.
gaussdb=# set behavior_compat_options = 'varray_compat'; SET gaussdb=# declare gaussdb-# type array_integer is varray(10) of integer; gaussdb-# arrint array_integer := array_integer(1,2,3); gaussdb-# begin gaussdb$# dbe_output.print_line(arrint.count); gaussdb$# arrint.delete(); gaussdb$# dbe_output.print_line(arrint.count); gaussdb$# end; gaussdb$# / 3 0 ANONYMOUS BLOCK EXECUTE -- An error is reported when the array is not initialized. gaussdb=# set behavior_compat_options = 'varray_compat'; SET gaussdb=# declare gaussdb-# type array_integer is varray(10) of integer; gaussdb-# arrint array_integer; gaussdb-# begin gaussdb$# arrint.delete(); gaussdb$# end; gaussdb$# / ERROR: Reference to uninitialized collection CONTEXT: PL/SQL function inline_code_block line 5 at assignment
- delete(idx)
Return value: No value is returned.
Description: If the specified index idx is within the array range, the element with the specified index idx is deleted from the array.
Example 1: The given index idx is within the array range.
gaussdb=# set behavior_compat_options = ''; SET gaussdb=# declare gaussdb-# type array_integer is varray(10) of integer; gaussdb-# arrint array_integer := array_integer(1, 2, 3); gaussdb-# begin gaussdb$# dbe_output.print_line(arrint.count); gaussdb$# arrint.delete(2); gaussdb$# dbe_output.print_line(arrint.count); gaussdb$# for i in 1..arrint.count loop gaussdb$# dbe_output.print_line(arrint(i)); gaussdb$# end loop; gaussdb$# end; gaussdb$# / 3 2 1 3 ANONYMOUS BLOCK EXECUTE
Example 2: The specified idx index is out of the array range, and the varray_compat parameter is not enabled.
-- The varray_compat parameter is not enabled. gaussdb=# set behavior_compat_options = ''; SET gaussdb=# declare gaussdb-# type array_integer is varray(10) of integer; gaussdb-# arrint array_integer := array_integer(1, 2, 3); gaussdb-# begin gaussdb$# dbe_output.print_line(arrint.count); gaussdb$# arrint.delete(4); gaussdb$# raise info '%', arrint; gaussdb$# end; gaussdb$# / 3 INFO: {1,2,3} ANONYMOUS BLOCK EXECUTE gaussdb=# declare gaussdb-# type array_integer is varray(10) of integer; gaussdb-# arrint array_integer:= array_integer(1, 2, 3); gaussdb-# begin gaussdb$# dbe_output.print_line(arrint.count); gaussdb$# arrint.delete(11); gaussdb$# raise info '%', arrint; gaussdb$# end; gaussdb$# / 3 INFO: {1,2,3} ANONYMOUS BLOCK EXECUTE -- An error is reported after the varray_compat parameter is enabled. gaussdb=# set behavior_compat_options = 'varray_compat'; SET gaussdb=# declare gaussdb-# type array_integer is varray(10) of integer; gaussdb-# arrint array_integer := array_integer(1, 2, 3); gaussdb-# begin gaussdb$# dbe_output.print_line(arrint.count); gaussdb$# arrint.delete(4); gaussdb$# raise info '%', arrint; gaussdb$# end; gaussdb$# / 3 ERROR: Subscript beyond count CONTEXT: PL/SQL function inline_code_block line 6 at assignment gaussdb=# set behavior_compat_options = 'varray_compat'; SET gaussdb=# declare gaussdb-# type array_integer is varray(10) of integer; gaussdb-# arrint array_integer:= array_integer(1, 2, 3); gaussdb-# begin gaussdb$# dbe_output.print_line(arrint.count); gaussdb$# arrint.delete(11); gaussdb$# raise info '%', arrint; gaussdb$# end; gaussdb$# / 3 ERROR: Subscript outside of limit CONTEXT: PL/SQL function inline_code_block line 6 at assignment
Example 3: The specified idx index is out of the array range, and the varray_compat parameter is enabled.
-- An error is reported after the varray_compat parameter is enabled. gaussdb=# set behavior_compat_options = 'varray_compat'; SET gaussdb=# declare gaussdb-# type array_integer is varray(10) of integer; gaussdb-# arrint array_integer := array_integer(1, 2, 3); gaussdb-# begin gaussdb$# dbe_output.print_line(arrint.count); gaussdb$# arrint.delete(4); gaussdb$# raise info '%', arrint; gaussdb$# end; gaussdb$# / 3 ERROR: Subscript beyond count CONTEXT: PL/SQL function inline_code_block line 6 at assignment gaussdb=# set behavior_compat_options = 'varray_compat'; SET gaussdb=# declare gaussdb-# type array_integer is varray(10) of integer; gaussdb-# arrint array_integer:= array_integer(1, 2, 3); gaussdb-# begin gaussdb$# dbe_output.print_line(arrint.count); gaussdb$# arrint.delete(11); gaussdb$# raise info '%', arrint; gaussdb$# end; gaussdb$# / 3 ERROR: Subscript outside of limit CONTEXT: PL/SQL function inline_code_block line 6 at assignment
Example 4: When the varray_compat parameter is enabled, an error is reported if the array is not initialized.
gaussdb=# set behavior_compat_options = 'varray_compat'; SET gaussdb=# declare gaussdb-# type array_integer is varray(10) of integer; gaussdb-# arrint array_integer; gaussdb-# begin gaussdb$# arrint.delete(2); gaussdb$# end; gaussdb$# / ERROR: Reference to uninitialized collection CONTEXT: PL/pgSQL function inline_code_block line 4 at assignment
- first[()]
Return value: int4 type.
Description: Returns the index of the first element in an array. If there is no first element, NULL is returned.
Example:
gaussdb=# set behavior_compat_options = ''; SET gaussdb=# declare gaussdb-# type varr is varray(10) of varchar(3); gaussdb-# v varr := varr('asd','zxc'); gaussdb-# begin gaussdb$# raise info 'first is %',v.first(); gaussdb$# end; gaussdb$# / INFO: first is 1 ANONYMOUS BLOCK EXECUTE -- If the array is not initialized, NULL is returned. gaussdb=# declare gaussdb-# type varr is varray(10) of varchar(3); gaussdb-# v varr; gaussdb-# begin gaussdb$# raise info 'first is %',v.first; gaussdb$# end; gaussdb$# / INFO: first is <NULL> ANONYMOUS BLOCK EXECUTE -- After the varray_copmat parameter is enabled, an error is reported when the array is not initialized. gaussdb=# set behavior_compat_options = 'varray_compat'; SET gaussdb=# declare gaussdb-# type varr is varray(10) of varchar(3); gaussdb-# v varr; gaussdb-# begin gaussdb$# raise info 'first is %',v.first; gaussdb$# end; gaussdb$# / ERROR: Reference to uninitialized collection CONTEXT: PL/pgSQL function inline_code_block line 4 at RAISE
- last[()]
Return value: int4 type.
Description: Returns the index of the last element in an array. If there is no last element, NULL is returned.
Example 1:
gaussdb=# set behavior_compat_options = ''; SET gaussdb=# declare gaussdb-# type varr is varray(10) of varchar(3); gaussdb-# v varr := varr('asd','zxc'); gaussdb-# begin gaussdb$# raise info 'last is %',v.last(); gaussdb$# end; gaussdb$# / INFO: last is 2 ANONYMOUS BLOCK EXECUTE -- If the array is not initialized, NULL is returned. gaussdb=# declare gaussdb-# type varr is varray(10) of varchar(3); gaussdb-# v varr; gaussdb-# begin gaussdb$# raise info 'last is %',v.last; gaussdb$# end; gaussdb$# / INFO: last is <NULL> ANONYMOUS BLOCK EXECUTE -- After the varray_copmat parameter is enabled, an error is reported when the array is not initialized. gaussdb=# set behavior_compat_options = 'varray_compat'; SET gaussdb=# declare gaussdb-# type varr is varray(10) of varchar(3); gaussdb-# v varr; gaussdb-# begin gaussdb$# raise info 'first is %',v.last; gaussdb$# end; gaussdb$# / ERROR: Reference to uninitialized collection CONTEXT: PL/pgSQL function inline_code_block line 4 at RAISE
- prior(idx)
Return value: int4 type.
Description: Returns the index of the element before the specified index in an array. If the index of the element cannot be found, NULL is returned.
Example:
gaussdb=# set behavior_compat_options = ''; SET gaussdb=# declare gaussdb-# type varr is varray(10) of varchar(3); gaussdb-# v varr := varr('asd','zxc'); gaussdb-# begin gaussdb$# raise info 'prior is %',v.prior(2); gaussdb$# end; gaussdb$# / INFO: prior is 1 ANONYMOUS BLOCK EXECUTE -- The index is out of range and is greater than the array range. gaussdb=# declare gaussdb-# type varr is varray(10) of varchar(3); gaussdb-# v varr := varr('asd','zxc','qwe'); gaussdb-# begin gaussdb$# raise info 'prior is %',v.prior(10); gaussdb$# end; gaussdb$# / INFO: prior is 3 ANONYMOUS BLOCK EXECUTE -- After the varray_copmat parameter is enabled, an error is reported when the array is not initialized. gaussdb=# set behavior_compat_options = 'varray_compat'; SET gaussdb=# declare gaussdb-# type varr is varray(10) of varchar(3); gaussdb-# v varr; gaussdb-# begin gaussdb$# raise info 'prior is %',v.prior(2); gaussdb$# end; gaussdb$# / ERROR: Reference to uninitialized collection CONTEXT: PL/pgSQL function inline_code_block line 4 at RAISE
- next(idx)
Return value: int4 type.
Description: Returns the index of the element following the specified index in an array. If the index of an element cannot be found, NULL is returned.
Example:
gaussdb=# set behavior_compat_options = ''; SET gaussdb=# declare gaussdb-# type varr is varray(10) of varchar(3); gaussdb-# v varr := varr('asd','zxc'); gaussdb-# begin gaussdb$# raise info 'next is %',v.next(1); gaussdb$# end; gaussdb$# / INFO: next is 2 ANONYMOUS BLOCK EXECUTE -- The index is out of range and is greater than the array range. gaussdb=# declare gaussdb-# type varr is varray(10) of varchar(3); gaussdb-# v varr := varr('asd','zxc','qwe'); gaussdb-# begin gaussdb$# raise info 'next is %',v.next(10); gaussdb$# end; gaussdb$# / INFO: next is <NULL> ANONYMOUS BLOCK EXECUTE -- After the varray_copmat parameter is enabled, an error is reported when the array is not initialized. gaussdb=# set behavior_compat_options = 'varray_compat'; SET gaussdb=# declare gaussdb-# type varr is varray(10) of varchar(3); gaussdb-# v varr; gaussdb-# begin gaussdb$# raise info 'next is %',v.next(1); gaussdb$# end; gaussdb$# / ERROR: Reference to uninitialized collection CONTEXT: PL/pgSQL function inline_code_block line 4 at RAISE
- exists(idx)
Return value: true or false, of the Boolean type.
Description: Checks whether a valid element exists in a specified position.
Example:
gaussdb=# declare gaussdb-# type varr is varray(10) of varchar(3); gaussdb-# v varr := varr('asd','zxc'); gaussdb-# flag bool; gaussdb-# begin gaussdb$# flag := v.exists(1); gaussdb$# raise info '%',flag; gaussdb$# flag := v.exists(3); gaussdb$# raise info '%',flag; gaussdb$# flag := v.exists(7); gaussdb$# raise info '%',flag; gaussdb$# end; gaussdb$# / INFO: t INFO: f INFO: f ANONYMOUS BLOCK EXECUTE -- If the array is not initialized, false is returned. gaussdb=# declare gaussdb-# type varr is varray(10) of varchar(3); gaussdb-# v varr; gaussdb-# flag bool; gaussdb-# begin gaussdb$# flag := v.exists(1); gaussdb$# raise info '%',flag; gaussdb$# flag := v.exists(3); gaussdb$# raise info '%',flag; gaussdb$# flag := v.exists(7); gaussdb$# raise info '%',flag; gaussdb$# end; gaussdb$# / INFO: f INFO: f INFO: f ANONYMOUS BLOCK EXECUTE
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot