Help Center/
GaussDB/
Developer Guide(Centralized_8.x)/
Stored Procedure/
Arrays, Collections, and Records/
Records
Updated on 2024-08-20 GMT+08:00
Records
Record Variables
Perform the following operations to create a record variable:
Define a record type and use this type to declare a variable.
Syntax
For the syntax of the record type, see Figure 1.
The above syntax diagram is explained as follows:
- record_type: record type
- field: record columns
- datatype: record data type
- expression: expression for setting a default value
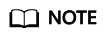
In GaussDB:
- When assigning values to record variables, you can:
- Declare a record type and define member variables of this type when you declare a function or stored procedure.
- Assign the value of a record variable to another record variable.
- Use SELECT INTO or FETCH to assign values to a record type.
- Assign the NULL value to a record variable.
- The record type constructor can use => to assign values. The restrictions are as follows:
- The => function is used when the record type constructor assigns values. This function is supported only when the A-compatible database is used (sql_compatibility is set to 'A').
- The record type can be defined in a package.
- The record type constructor uses the => function to assign values. This function is supported only when => is consecutively used to assign values to input parameters, for example, record_name(elename1 => val1, elename2 => val2, elename3 => val3) or record_name(val1, elename2 => val2, elename3 => val3). Discontinuous use of => or => is not supported. For example, record_name(elename1 => val1, elename2 => val2, val3) or record_name(val1, elename2 => val2, val3), where the last input parameter is not assigned a value.
- The INSERT and UPDATE statements cannot use a record variable to insert or update data.
- The constructor of the record type cannot be used as the default value of a function or stored procedure parameter.
- Just like a variable, a record column of the compound type does not have a default value in the declaration.
- If a member has a record type, the default value of the inner record type cannot be not transferred to the outer record type.
- If set enable_recordtype_check_strict is set to on and the member is of the record type and a column of the record type has the not null or default attribute, an error is reported during stored procedure or package compilation.
- If set enable_recordtype_check_strict is set to on, a package contains the record type, and a column of the record type has the not null or default attribute, an error is reported during creation or compilation.
- If set enable_recordtype_check_strict is set to on, the not null function of the record type in the stored procedure takes effect.
- The data type can be the record type, array type, or collection type defined in a stored procedure (anonymous blocks are not supported).
- After the GUC parameter set behavior_compat_options is set to 'proc_outparam_override':
- Functions that contain the out output parameter and returns data of the record type can be invoked using SELECT and CALL in external SQL statements, and can be invoked using PERFORM and expressions in stored procedures.
- If the function returns data of the undefined record type, at least one out parameter is required. If the function returns data of the defined record type, the out parameter is optional. For details, see the example.
- Functions that contain the out output parameter and return data of the composite or record type, ensure that the expected return type of the function is the same as the actual return type.
Examples
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 |
-- Create a table and insert some data into the table. gaussdb=# create table emp_rec( gaussdb(# empno numeric(4,0) not null, gaussdb(# ename varchar(10) gaussdb(# ); CREATE TABLE gaussdb=# insert into emp_rec values(111, 'aaa'), (222, 'bbb'), (333, 'ccc'); INSERT 0 3 -- The table is defined as follows: gaussdb=# \d emp_rec Table "public.emp_rec" Column | Type | Modifiers --------+-----------------------+----------- empno | numeric(4,0) | not null ename | character varying(10) | -- Perform record operations in the function. gaussdb=# CREATE OR REPLACE FUNCTION regress_record(p_w VARCHAR2) RETURNS VARCHAR2 AS $$ gaussdb$# DECLARE gaussdb$# -- Declare a record type. gaussdb$# type rec_type is record (name varchar2(100), epno int); gaussdb$# employer rec_type; gaussdb$# -- Use %type to declare the record type. gaussdb$# type rec_type1 is record (name emp_rec.ename%type, epno int :=10); gaussdb$# employer1 rec_type1; gaussdb$# -- Declare a record type with the default value. gaussdb$# type rec_type2 is record ( gaussdb$# name varchar2 not null := 'SCOTT', gaussdb$# epno int not null :=10 gaussdb$# ); gaussdb$# employer2 rec_type2; gaussdb$# CURSOR C1 IS select ename,empno from emp_rec order by 1 limit 1; gaussdb$# BEGIN gaussdb$# -- Assign a value to a member record variable. gaussdb$# employer.name := 'WARD'; gaussdb$# employer.epno = 18; gaussdb$# raise info 'employer name: % , epno:%', employer.name, employer.epno; gaussdb$# gaussdb$# -- Assign the value of a record variable to another variable. gaussdb$# employer1 := employer; gaussdb$# raise info 'employer1 name: % , epno: %',employer1.name, employer1.epno; gaussdb$# gaussdb$# -- Assign the NULL value to a record variable. gaussdb$# employer1 := NULL; gaussdb$# raise info 'employer1 name: % , epno: %',employer1.name, employer1.epno; gaussdb$# gaussdb$# -- Obtain the default value of a record variable. gaussdb$# raise info 'employer2 name: % ,epno: %', employer2.name, employer2.epno; gaussdb$# gaussdb$# -- Use a record variable in the FOR loop. gaussdb$# for employer in select ename,empno from emp_rec order by 1 limit 1 loop gaussdb$# raise info 'employer name: % , epno: %', employer.name, employer.epno; gaussdb$# end loop; gaussdb$# gaussdb$# -- Use a record variable in the SELECT INTO statement. gaussdb$# select ename,empno into employer2 from emp_rec order by 1 limit 1; gaussdb$# raise info 'employer name: % , epno: %', employer2.name, employer2.epno; gaussdb$# gaussdb$# -- Use a record variable in a cursor. gaussdb$# OPEN C1; gaussdb$# FETCH C1 INTO employer2; gaussdb$# raise info 'employer name: % , epno: %', employer2.name, employer2.epno; gaussdb$# CLOSE C1; gaussdb$# RETURN employer.name; gaussdb$# END; $$ gaussdb-# LANGUAGE plpgsql; CREATE FUNCTION -- Call this function. gaussdb=# CALL regress_record('abc'); INFO: employer name: WARD , epno:18 INFO: employer1 name: WARD , epno: 18 INFO: employer1 name: <NULL> , epno: <NULL> INFO: employer2 name: SCOTT ,epno: 10 INFO: employer name: aaa , epno: 111 INFO: employer name: aaa , epno: 111 INFO: employer name: aaa , epno: 111 regress_record ---------------- aaa (1 row) -- Delete the function. gaussdb=# DROP FUNCTION regress_record; DROP FUNCTION -- Delete the table. gaussdb=# DROP TABLE emp_rec; DROP TABLE -- If the compatibility parameter proc_outparam_override is enabled, the data of the defined record type is returned. The out parameter is not required for the function. gaussdb=# create type rec_type is (c1 int, c2 int); CREATE TYPE gaussdb=# set behavior_compat_options = 'proc_outparam_override'; SET gaussdb=# create or replace function func(a in int) return rec_type is gaussdb$# r rec_type; gaussdb$# begin gaussdb$# r.c1:=1; gaussdb$# r.c2:=1; gaussdb$# return r; gaussdb$# end; gaussdb$# / CREATE FUNCTION gaussdb=# call func(0); c1 | c2 ----+---- 1 | 1 (1 row) gaussdb=# drop function func; DROP FUNCTION gaussdb=# drop type rec_type; DROP TYPE -- If the compatibility parameter proc_outparam_override is enabled, the data of the undefined record type is returned and at least one out parameter is required for the function gaussdb=# set behavior_compat_options = 'proc_outparam_override'; SET gaussdb=# create or replace function func(a out int) return record is gaussdb$# type rc is record(c1 int, c2 int); gaussdb$# r rc; gaussdb$# begin gaussdb$# r.c1 := 1; gaussdb$# r.c2 := 1; gaussdb$# a := 999; gaussdb$# return r; gaussdb$# end; gaussdb$# / CREATE FUNCTION gaussdb=# call func(1); func | a -------+----- (1,1) | 999 (1 row) gaussdb=# drop function func; DROP FUNCTION -- Create an A-compatible database and switch to this database. gaussdb=# CREATE DATABASE ora_compatible_db DBCOMPATIBILITY 'A'; CREATE DATABASE gaussdb=# \c ora_compatible_db; Non-SSL connection (SSL connection is recommended when requiring high-security) You are now connected to database "ora_compatible_db" as user "omm". -- Define the record in the pkg. Use => to assign values to all input parameters of the record constructor. ora_compatible_db=# CREATE or REPLACE package PA_TEST1 AS PROCEDURE P_DISPLAY1(a NUMBER, b VARCHAR); TYPE t1 IS record(va int,vb int); v1 t1; END PA_TEST1; / ora_compatible_db=# CREATE or REPLACE package body PA_TEST1 AS PROCEDURE P_DISPLAY1(a NUMBER, b VARCHAR) AS BEGIN v1 := t1(va => 1, vb => 2); dbe_output.put_line('pkg test1'); END; END PA_TEST1; / ora_compatible_db=# CALL PA_TEST1.P_DISPLAY1(b => 'm', a => 1); p_display1 ------------ (1 row) -- Define the record in the pkg. The input parameter values of the record constructor are not consecutively used =>, and an error message is displayed. ora_compatible_db=# CREATE or REPLACE package PA_TEST1 AS PROCEDURE P_DISPLAY1(a NUMBER, b VARCHAR); TYPE t1 IS record(va int,vb int); v1 t1; END PA_TEST1; / CREATE or REPLACE package body PA_TEST1 AS PROCEDURE P_DISPLAY1(a NUMBER, b VARCHAR) AS BEGIN v1 := t1(va => 1, 2); dbe_output.put_line('pkg test1'); END; END PA_TEST1; / ora_compatible_db=# CALL PA_TEST1.P_DISPLAY1(b => 'm', a => 1); ERROR: positional argument cannot follow named argument LINE 1: SELECT "17347.t1"(va => 1, 2) ^ QUERY: SELECT "17347.t1"(va => 1, 2) CONTEXT: referenced column: 17347.t1 PL/pgSQL function p_display1(numeric,character varying) line 2 at assignment -- Delete the created database and switch back to the original database. ora_compatible_db=# \c postgres; Non-SSL connection (SSL connection is recommended when requiring high-security) You are now connected to database "postgres" as user "omm". gaussdb=# DROP DATABASE ora_compatible_db; DROP DATABASE |
Parent topic: Arrays, Collections, and Records
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
The system is busy. Please try again later.
For any further questions, feel free to contact us through the chatbot.
Chatbot