如何指定Content-SHA256?
上传对象和上传段支持携带x-obs-content-sha256头域,该头域值为请求消息体256-bit SHA256值转十六进制值,计算方式为Hex(SHA256Hash(<payload>),服务端会对携带此头域的请求计算其消息体的sha256值做校验(性能会有部分下降,在安全上推荐该算法),示例代码如下:
本示例用于删除名为examplebucket桶的异步抓取策略
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 |
package main import ( "crypto/sha256" "fmt" "io" "os" obs "github.com/huaweicloud/huaweicloud-sdk-go-obs/obs" ) func sha256File(path string) string { file, err := os.Open(path) // Open the file for reading if err != nil { panic(err) } defer file.Close() // Be sure to close your file! hash := sha256.New() // Use the Hash in crypto/sha256 if _, err := io.Copy(hash, file); err != nil { panic(err) } return fmt.Sprintf("%x", hash.Sum(nil)) } func main() { //推荐通过环境变量获取AKSK,这里也可以使用其他外部引入方式传入,如果使用硬编码可能会存在泄露风险。 //您可以登录访问管理控制台获取访问密钥AK/SK,获取方式请参见https://support.huaweicloud.com/usermanual-ca/ca_01_0003.html。 ak := os.Getenv("AccessKeyID") sk := os.Getenv("SecretAccessKey") // 【可选】如果使用临时AK/SK和SecurityToken访问OBS,同样建议您尽量避免使用硬编码,以降低信息泄露风险。您可以通过环境变量获取访问密钥AK/SK,也可以使用其他外部引入方式传入。 securityToken := os.Getenv("SecurityToken") // endpoint填写Bucket对应的Endpoint, 这里以华北-北京四为例,其他地区请按实际情况填写。 endPoint := "https://obs.cn-north-4.myhuaweicloud.com" // 创建obsClient实例 // 如果使用临时AKSK和SecurityToken访问OBS,需要在创建实例时通过obs.WithSecurityToken方法指定securityToken值。 obsClient, err := obs.New(ak, sk, endPoint, obs.WithSecurityToken(securityToken), obs.WithSignature(obs.SignatureObs)) if err != nil { fmt.Printf("Create obsClient error, errMsg: %s", err.Error()) } input := &obs.PutObjectInput{} // 指定存储桶名称 input.Bucket = "examplebucket" // 指定下载对象,此处以 example/objectname 为例。 input.Key = "example/objectname" filePath := "localfile" fd, _ := os.Open(filePath) defer fd.Close() input.Body = fd // 流式上传本地文件 output, err := obsClient.PutObject(input, obs.WithCustomHeader("x-obs-content-sha256", sha256File(filePath))) if err == nil { fmt.Printf("Put object(%s) under the bucket(%s) successful!\n", input.Key, input.Bucket) fmt.Printf("StorageClass:%s, ETag:%s\n", output.StorageClass, output.ETag) return } fmt.Printf("Put object(%s) under the bucket(%s) fail!\n", input.Key, input.Bucket) if obsError, ok := err.(obs.ObsError); ok { fmt.Println("An ObsError was found, which means your request sent to OBS was rejected with an error response.") fmt.Println(obsError.Error()) } else { fmt.Println("An Exception was found, which means the client encountered an internal problem when attempting to communicate with OBS, for example, the client was unable to access the network.") fmt.Println(err) } } |
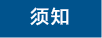
SDK 同时支持MD5与SHA256校验,在安全上更推荐使用SHA256算法。