Java
Scenarios
To use Java to call an API through app authentication, obtain the Java SDK, import the sample code, and then call the API by referring to the API calling example.
This section uses Eclipse 4.5.2 as an example.
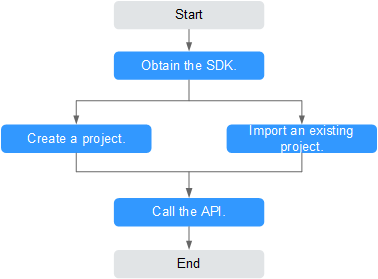
Prerequisites
- You have obtained API calling information. For details, see Preparation.
- You have installed Eclipse 3.6.0 or a later version. If not, download Eclipse from the official Eclipse website and install it.
- You have installed IntelliJ IDEA 2022.2.1. If not, download the installation package from the official IntelliJ IDEA website and install it.
- You have installed Java Development Kit (JDK) 1.8.111 or a later version. If not, download JDK from the official Oracle website and install it. JDK 17 or later is not supported.
Obtaining the SDK
Log in to the APIG console, and download the SDK on the SDKs page by referring to section "SDKs" in the API Gateway User Guide.
Alternatively, download the latest SDK version. Then obtain the ApiGateway-java-sdk.zip package. The following table shows the files decompressed from the package.
Name |
Description |
---|---|
libs\java-sdk-core-x.x.x.jar |
Signing SDK |
pom.xml |
Required for defining dependencies when building Maven projects |
changelog |
Change log |
src |
Demo code of the signature verification SDK
Classes:
|
Configuring IDEA
Use any of the following configuration methods:
- Importing the Sample Project
- Start IDEA and choose File > New > Project from Existing Sources.
Select the decompressed APIGW-java-sdk-x.x.x folder, and click OK to import the sample project.
- On the Import Project page, select Create project from existing sources.
Click Next until Import Project is displayed, select Maven, and click Create.
- You can create a project in the current window or a new window. In this example, click New Window.
- Start IDEA and choose File > New > Project from Existing Sources.
- Creating a Maven Project
- Start IDEA and choose File > New > Project.
- Select New Project, set the following parameters, and click Create.
Name: Enter apig-sdk-maven-demo.
Build System: Select Maven.
JDK: Select the version you use.
- Click New Window. You can also create a project in the current window.
- Copy the src and libs folders in the sample project to the apig-sdk-maven-demo project.
- Configure the pom.xml file of the new Maven project.
Expand the project file on the left, double-click pom.xml, and replace the file content with the following code:
Download the dependency to the local repository for packaging.
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.huawei.apigateway</groupId> <artifactId>java</artifactId> <version>1.0.0</version> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.8.0</version> <configuration> <source>1.8</source> <target>1.8</target> <encoding>UTF-8</encoding> </configuration> </plugin> </plugins> </build> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <maven.compiler.source>1.8</maven.compiler.source> <maven.compiler.target>1.8</maven.compiler.target> </properties> <dependencies> <dependency> <groupId>commons-codec</groupId> <artifactId>commons-codec</artifactId> <version>1.15</version> </dependency> <dependency> <groupId>commons-logging</groupId> <artifactId>commons-logging</artifactId> <version>1.2</version> </dependency> <dependency> <groupId>org.apache.httpcomponents</groupId> <artifactId>httpclient</artifactId> <version>4.5.13</version> </dependency> <dependency> <groupId>com.squareup.okhttp3</groupId> <artifactId>okhttp</artifactId> <version>4.9.1</version> </dependency> <dependency> <groupId>org.apache.httpcomponents</groupId> <artifactId>httpcore</artifactId> <version>4.4.13</version> </dependency> <dependency> <groupId>org.slf4j</groupId> <artifactId>slf4j-api</artifactId> <version>1.7.25</version> <scope>compile</scope> </dependency> <dependency> <groupId>org.slf4j</groupId> <artifactId>slf4j-simple</artifactId> <version>1.7.25</version> </dependency> <dependency> <!--Replace this with the actual path.--> <systemPath>${project.basedir}/libs/java-sdk-core-XXX.jar</systemPath> <groupId>com.huawei.apigateway</groupId> <artifactId>java-sdk-core</artifactId> <version>SDK package version</version> <scope>system</scope> </dependency> <dependency> <groupId>org.openeuler</groupId> <artifactId>bgmprovider</artifactId> <version>1.0.3</version> </dependency> </dependencies> </project>
- Configure the Maven configuration file settings.xml.
- Add the following content to the profiles section:
<profile> <id>MyProfile</id> <repositories> <repository> <id>HuaweiCloudSDK</id> <url>https://mirrors.huaweicloud.com/repository/maven/huaweicloudsdk/</url> <releases> <enabled>true</enabled> </releases> <snapshots> <enabled>false</enabled> </snapshots> </repository> </repositories> <pluginRepositories> <pluginRepository> <id>HuaweiCloudSDK</id> <url>https://mirrors.huaweicloud.com/repository/maven/huaweicloudsdk/</url> <releases> <enabled>true</enabled> </releases> <snapshots> <enabled>false</enabled> </snapshots> </pluginRepository> </pluginRepositories> </profile>
- Add the following content to the mirrors section:
<mirror> <id>huaweicloud</id> <mirrorOf>*,!HuaweiCloudSDK</mirrorOf> <url>https://repo.huaweicloud.com/repository/maven/</url> </mirror>
- Add the activeProfiles tag to activate the configurations.
<activeProfiles> <activeProfile>MyProfile</activeProfile> </activeProfiles>
- Add the following content to the profiles section:
- To download the Maven dependency, right-click pom.xml, and choose Maven > Reload project from the shortcut menu.
- Expand the src file under the project on the left and double-click HttpClientDemo. If a green arrow is displayed, the creation is successful, as shown in the following figure.
API Calling Example
- Replace the API information in the HttpClientDemo.java file.
- In this example, the AK and SK stored in the environment variables are used. Specify the environment variables HUAWEICLOUD_SDK_AK and HUAWEICLOUD_SDK_SK in the local environment first. The following uses Linux as an example to describe how to set the obtained AK/SK as environment variables.
- Open the terminal and run the following command to open the environment variable configuration file:
- Set environment variables, save the file, and exit the editor.
export HUAWEICLOUD_SDK_AK="Obtained AK" export HUAWEICLOUD_SDK_SK="Obtained SK"
- Run the following command to apply the modification:
- Replace the API information and configured environment variables in the HttpClientDemo.java file.
HttpClientDemo references the following classes (view them in the src file mentioned in section "Obtaining the SDK"):
- Constant: constants used in the demo
- SSLCipherSuiteUtil: TLS authentication configuration tool. For example, it can be used to configure skipping certificate verification on clients.
- UnsupportProtocolException: exception handling class
public class HttpClientDemo { private static final Logger LOGGER = LoggerFactory.getLogger(HttpClientDemo.class); public static void main(String[] args) throws Exception { // Create a new request. Request httpClientRequest = new Request(); try { // Set the request parameters. // AppKey, AppSecrect, Method and Url are required parameters. // Directly writing AK/SK in code is risky. For security, encrypt your AK/SK and store them in the configuration file or environment variables. // In this example, the AK/SK are stored in environment variables for identity authentication. // Before running this example, set environment variables HUAWEICLOUD_SDK_AK and HUAWEICLOUD_SDK_SK. httpClientRequest.setKey(System.getenv("HUAWEICLOUD_SDK_AK")); httpClientRequest.setSecret(System.getenv("HUAWEICLOUD_SDK_SK")); httpClientRequest.setMethod("POST"); // Set a request URL in the format of https://{Endpoint}/{URI}. httpClientRequest.setUrl("put your request url here"); httpClientRequest.addHeader("Content-Type", "text/plain"); // Set a body for http request. httpClientRequest.setBody("put your request body here"); } catch (Exception e) { LOGGER.error(e.getMessage()); return; } CloseableHttpClient client = null; try { // Sign the request. HttpRequestBase signedRequest = Client.sign(httpClientRequest, Constant.SIGNATURE_ALGORITHM_SDK_HMAC_SHA256); if (Constant.DO_VERIFY) { // creat httpClient and verify ssl certificate HostName.setUrlHostName(httpClientRequest.getHost()); client = (CloseableHttpClient) SSLCipherSuiteUtil.createHttpClientWithVerify(Constant.INTERNATIONAL_PROTOCOL); } else { // creat httpClient and do not verify ssl certificate client = (CloseableHttpClient) SSLCipherSuiteUtil.createHttpClient(Constant.INTERNATIONAL_PROTOCOL); } HttpResponse response = client.execute(signedRequest); // Print the body of the response. HttpEntity resEntity = response.getEntity(); if (resEntity != null) { LOGGER.info("Processing Body with name: {} and value: {}", System.getProperty("line.separator"), EntityUtils.toString(resEntity, "UTF-8")); } } catch (Exception e) { LOGGER.error(e.getMessage()); } finally { if (client != null) { client.close(); } } } }
- In this example, the AK and SK stored in the environment variables are used. Specify the environment variables HUAWEICLOUD_SDK_AK and HUAWEICLOUD_SDK_SK in the local environment first. The following uses Linux as an example to describe how to set the obtained AK/SK as environment variables.
- Run HttpClientDemo.java to sign the request, access the API, and print the result.
The following is an example output:
[main] INFO com.huawei.apig.sdk.demo.HttpClientDemo - Print the authorization: [Authorization: SDK-HMAC-SHA256 Access=3afe0280a6e1466e9cb6f23bcccdba29, SignedHeaders=host;x-sdk-date, Signature=26b2abfa40a4acf3c38b286cb6cbd9f07c2c22d1285bf0d4f6cf1f02d3bfdbf6] [main] INFO com.huawei.apig.sdk.demo.HttpClientDemo - Print the status line of the response: HTTP/1.1 200 OK [main] INFO com.huawei.apig.sdk.demo.HttpClientDemo - Processing Header with name: Date and value: Fri, 26 Aug 2022 08:58:51 GMT [main] INFO com.huawei.apig.sdk.demo.HttpClientDemo - Processing Header with name: Content-Type and value: application/json [main] INFO com.huawei.apig.sdk.demo.HttpClientDemo - Processing Header with name: Transfer-Encoding and value: chunked [main] INFO com.huawei.apig.sdk.demo.HttpClientDemo - Processing Header with name: Connection and value: keep-alive [main] INFO com.huawei.apig.sdk.demo.HttpClientDemo - Processing Header with name: Server and value: api-gateway [main] INFO com.huawei.apig.sdk.demo.HttpClientDemo - Processing Header with name: X-Request-Id and value: 10955c5346b9512d23f3fd4c1bf2d181 [main] INFO com.huawei.apig.sdk.demo.HttpClientDemo - Processing Body with name: and value: {"200": "sdk success"}
If {"200": "sdk success"} is displayed, the signing is successful and the API request is sent to the backend.
If the AK or SK has changed, APIG returns an error message.
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot