Updated on 2023-11-17 GMT+08:00
Go
Example |
|
---|---|
Environment |
Go 1.11 or later |
Library |
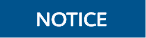
- Sending SMSs shows an example of sending group SMSs with a single template. Sending SMSs in batches shows an example of sending group SMSs with multiple templates.
- The examples described in this document may involve the use of personal data. You are advised to comply with relevant laws and regulations and take measures to ensure that personal data is fully protected.
- The examples described in this document are for demonstration purposes only. Commercial use of the examples is prohibited.
- Information in this document is for your reference only. It does not constitute any offer or commitment.
Sending SMSs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 |
package main
import (
"bytes"
"crypto/sha256"
"crypto/tls"
"encoding/base64"
"fmt"
"github.com/satori/go.uuid"
"io/ioutil"
"net/http"
"net/url"
"strings"
"time"
)
//This parameter does not need to be modified. It is used to format the Authentication header field and assign a value to the X-WSSE parameter.
const WSSE_HEADER_FORMAT = "UsernameToken Username=\"%s\",PasswordDigest=\"%s\",Nonce=\"%s\",Created=\"%s\""
//This parameter does not need to be modified. It is used to format the Authentication header field and assign a value to the Authorization parameter.
const AUTH_HEADER_VALUE = "WSSE realm=\"SDP\",profile=\"UsernameToken\",type=\"Appkey\""
func main() {
//Mandatory. The values here are example values only. Obtain the actual values based on Development Preparation.
apiAddress := "https://smsapi.ap-southeast-1.myhuaweicloud.com:443/sms/batchSendSms/v1" //Application access address (obtain it from the Application Management page on the console) and API access URI.
// Hard-coded or plaintext appKey/appSecret is risky. For security, encrypt your appKey/appSecret and store them in the configuration file or environment variables.
appKey := "c8RWg3ggEcyd4D3p94bf3Y7x1Ile" //APP_Key
appSecret := "q4Ii87Bh************80SfD7Al" //APP_Secret
sender := "csms12345678" //Channel number for the Chinese mainland SMS or international SMS
templateId := "8ff55eac1d0b478ab3c06c3c6a492300" //Template ID
//Mandatory for Chinese mainland SMS. This parameter is valid and mandatory when Template Type corresponding to templateId is Universal template. The signature name must be approved and be the same type as the template.
//This parameter is not required for international SMS.
signature := "Huawei Cloud SMS test" // Signature
// Mandatory. Global number format (including the country code), for example, +8615123456789. Use commas (,) to separate multiple numbers.
receiver := "+8615123456789,+8615234567890" //Recipient numbers
// Optional. Address for receiving SMS status reports. The domain name is recommended. If this parameter is set to an empty value or left unspecified, customers do not receive status reports.
statusCallBack := ""
/*
* Optional. If a non-variable template is used, assign an empty value to this parameter, for example, string templateParas = "";
* Example of a single-variable template: If the template content is "Your verification code is ${1}", templateParas can be set to "[\"369751\"]".
* Example of a dual-variable template: If the template content is "You have ${1} delivered to ${2}", templateParas can be set to "[\"3\",\"main gate of People's Park\"]".
* Each variable in the template must be assigned a value, and the value cannot be empty.
* To view more information, choose Service Overview > Template and Variable Specifications.
*/
templateParas := "[\"369751\"]" //Template variable. The following uses a single-variable verification code SMS message as an example. The customer needs to generate a 6-digit verification code and define it as a character string to prevent the loss of first digits 0 (for example, 002569 is changed to 2569).
body := buildRequestBody(sender,receiver,templateId,templateParas,statusCallBack,signature)
headers := make(map[string]string)
headers["Content-Type"] = "application/x-www-form-urlencoded"
headers["Authorization"] = AUTH_HEADER_VALUE;
headers["X-WSSE"] = buildWsseHeader(appKey, appSecret);
resp, err := post(apiAddress, []byte(body),headers)
if err != nil {
return
}
fmt.Println(resp);
}
/**
* sender, receiver, and templateId cannot be empty.
*/
func buildRequestBody(sender, receiver, templateId, templateParas, statusCallBack, signature string) string {
param := "from=" + url.QueryEscape(sender) + "&to=" + url.QueryEscape(receiver) + "&templateId=" + url.QueryEscape(templateId)
if templateParas != "" {
param += "&templateParas=" + url.QueryEscape(templateParas)
}
if statusCallBack != "" {
param += "&statusCallback=" + url.QueryEscape(statusCallBack)
}
if signature != "" {
param += "&signature=" + url.QueryEscape(signature)
}
return param
}
func post(url string, param []byte, headers map[string]string)(string,error) {
tr := &http.Transport{
TLSClientConfig: &tls.Config{InsecureSkipVerify: true},
}
client := &http.Client{Transport: tr}
req, err := http.NewRequest("POST",url, bytes.NewBuffer(param));
if err != nil {
return "", err
}
for key, header := range headers {
req.Header.Set(key, header)
}
resp, err := client.Do(req)
defer resp.Body.Close()
body, err := ioutil.ReadAll(resp.Body)
if err != nil {
return "", err
}
return string(body), nil;
}
func buildWsseHeader(appKey,appSecret string)string {
var cTime = time.Now().Format("2006-01-02T15:04:05Z")
var nonce = uuid.NewV4().String()
nonce = strings.ReplaceAll(nonce,"-","")
h := sha256.New()
h.Write([]byte(nonce + cTime + appSecret))
passwordDigestBase64Str := base64.StdEncoding.EncodeToString(h.Sum(nil))
return fmt.Sprintf(WSSE_HEADER_FORMAT,appKey,passwordDigestBase64Str,nonce, cTime);
}
|
Sending SMSs in Batches
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 |
package main
import (
"bytes"
"crypto/sha256"
"crypto/tls"
"encoding/base64"
"encoding/json"
"fmt"
"github.com/satori/go.uuid"
"io/ioutil"
"net/http"
"strings"
"time"
)
//This parameter does not need to be modified. It is used to format the Authentication header field and assign a value to the X-WSSE parameter.
const WSSE_HEADER_FORMAT = "UsernameToken Username=\"%s\",PasswordDigest=\"%s\",Nonce=\"%s\",Created=\"%s\""
//This parameter does not need to be modified. It is used to format the Authentication header field and assign a value to the Authorization parameter.
const AUTH_HEADER_VALUE = "WSSE realm=\"SDP\",profile=\"UsernameToken\",type=\"Appkey\""
func main() {
//Mandatory. The values here are example values only. Obtain the actual values based on Development Preparation.
url := "https://smsapi.ap-southeast-1.myhuaweicloud.com:443/sms/batchSendDiffSms/v1" //Application access address (obtain it from the Application Management page on the console) and API access URI.
// Hard-coded or plaintext appKey/appSecret is risky. For security, encrypt your appKey/appSecret and store them in the configuration file or environment variables.
appKey := "c8RWg3ggEcyd4D3p94bf3Y7x1Ile" //APP_Key
appSecret := "q4Ii87Bh************80SfD7Al" //APP_Secret
sender := "csms12345678" //Channel number for the Chinese mainland SMS or international SMS
templateId1 := "8ff55eac1d0b478ab3c06c3c6a492300" //Template ID 1
templateId2 := "8ff55eac1d0b478ab3c06c3c6a492300" //Template ID 2
//Mandatory for Chinese mainland SMS. This parameter is valid and mandatory when Template Type corresponding to templateId is Universal template. The signature name must be approved and be the same type as the template.
//This parameter is not required for international SMS.
signature1 := "Huawei Cloud SMS test" // Signature 1
signature2 := "Huawei Cloud SMS test" // Signature 2
// Mandatory. Global number format (including the country code), for example, +8615123456789. Use commas (,) to separate multiple numbers.
receiver1 := []string{"+8615123456789", "+8615234567890"}; //Recipient number of template 1
receiver2 := []string{"+8615123456789", "+8615234567890"}; //Recipient number of template 2
// Optional. Address for receiving SMS status reports. The domain name is recommended. If this parameter is set to an empty value or left unspecified, customers do not receive status reports.
statusCallBack := "";
/**
* Optional. If a non-variable template is used, assign an empty value to this parameter, for example, String templateParas = {};
* Example of a single-variable template: If the template content is "Your verification code is ${1}", templateParas can be set to {"369751"}.
* Example of a dual-variable template: If the template content is "You have ${1} delivered to ${2}", templateParas can be set to {"3","main gate of People's Park"}.
* ${DATE}${TIME} cannot be empty. You can use spaces or dots (.) to replace the empty value of ${TXT_20} and use 0 to replace the empty value of ${NUM_6}.
* To view more information, choose Service Overview > Template and Variable Specifications.
*/
templateParas1 := []string{"123456"}; //Template 1 variable. The following uses a single-variable verification code SMS message as an example. The customer needs to generate a 6-digit verification code and define it as a character string to prevent the loss of first digits 0 (for example, 002569 is changed to 2569).
templateParas2 := []string{"234567"}; //Template 2 variable. The following uses a single-variable verification code SMS message as an example. The customer needs to generate a 6-digit verification code and define it as a character string to prevent the loss of first digits 0 (for example, 002569 is changed to 2569).
item1 := initDiffSms(receiver1, templateId1, templateParas1, signature1);
item2 := initDiffSms(receiver2, templateId2, templateParas2, signature2);
item := []map[string]interface{}{item1,item2}
body := buildRequestBody(sender, item, statusCallBack)
headers := make(map[string]string)
headers["Content-Type"] = "application/json;charset=utf-8"
headers["Authorization"] = AUTH_HEADER_VALUE;
headers["X-WSSE"] = buildWsseHeader(appKey, appSecret);
resp, err := post(url,body,headers)
if err != nil {
return
}
fmt.Println(resp);
}
func buildRequestBody(sender string, item []map[string]interface{}, statusCallBack string) []byte{
body := make(map[string]interface{})
body["smsContent"] = item
body["from"] = sender
if statusCallBack != "" {
body["statusCallback"] = statusCallBack
}
res, _ := json.Marshal(body)
return res;
}
func initDiffSms(reveiver []string, templateId string, templateParas []string, signature string) map[string]interface{} {
diffSms := make(map[string]interface{});
diffSms["to"] = reveiver
diffSms["templateId"] = templateId
if templateParas != nil && len(templateParas) > 0 {
diffSms["templateParas"] = templateParas
}
if signature != "" {
diffSms["signature"] = signature
}
return diffSms;
}
func post(url string, param []byte, headers map[string]string)(string,error) {
tr := &http.Transport{
TLSClientConfig: &tls.Config{InsecureSkipVerify: true},
}
client := &http.Client{Transport: tr}
req, err := http.NewRequest("POST",url, bytes.NewBuffer(param));
if err != nil {
return "", err
}
for key, header := range headers {
req.Header.Set(key, header)
}
resp, err := client.Do(req)
defer resp.Body.Close()
body, err := ioutil.ReadAll(resp.Body)
if err != nil {
return "", err
}
return string(body), nil;
}
func buildWsseHeader(appKey,appSecret string)string {
var cTime = time.Now().Format("2006-01-02T15:04:05Z")
var nonce = uuid.NewV4().String()
nonce = strings.ReplaceAll(nonce,"-","")
h := sha256.New()
h.Write([]byte(nonce + cTime + appSecret))
passwordDigestBase64Str := base64.StdEncoding.EncodeToString(h.Sum(nil))
return fmt.Sprintf(WSSE_HEADER_FORMAT,appKey,passwordDigestBase64Str,nonce, cTime);
}
|
Receiving Status Reports
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
package main
import (
"fmt"
"net/url"
"strings"
)
func main() {
//Data example (urlencode) of the status report reported by the SMS platform
//success_body := "sequence=1&total=1&updateTime=2018-10-31T08%3A43%3A41Z&source=2&smsMsgId=2ea20735-f856-4376-afbf-570bd70a46ee_11840135&status=DELIVRD";
failed_body := "orgCode=E200027&sequence=1&total=1&updateTime=2018-10-31T08%3A43%3A41Z&source=2&smsMsgId=2ea20735-f856-4376-afbf-570bd70a46ee_11840135&status=RTE_ERR";
//onSmsStatusReport(success_body);
onSmsStatusReport(failed_body);
}
func onSmsStatusReport(data string) {
ss, _ := url.QueryUnescape(data)
params := strings.Split(ss, "&")
keyValues := make(map[string]string)
for i := range params {
temp := strings.Split(params[i],"=")
keyValues[temp[0]] = temp[1];
}
/**
* Example: Parsing status is used as an example.
*
* 'smsMsgId': Unique ID of an SMS
* 'total': Number of SMS segments
* 'sequence': Sequence number of an SMS after splitting
* 'source': Status report source
* 'updateTime': Resource update time
* 'status': Enumeration values of the status report
* 'orgCode': Status code
*/
status := keyValues["status"];
if status == "DELIVRD" {
fmt.Println("Send sms success. smsMsgId: " + keyValues["smsMsgId"])
} else {
fmt.Println("Send sms failed. smsMsgId: " + keyValues["smsMsgId"])
fmt.Println("Failed status: " + keyValues["status"])
fmt.Println("Failed orgCode: " + keyValues["orgCode"])
}
}
|
References
- Code examples: Java, PHP, Python, C#, and Node.js
- APIs: SMS Sending API, Batch SMS Sending API, and Status Report Receiving API
- Troubleshooting Commissioning Problems
Parent topic: X-WSSE Authentication
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
The system is busy. Please try again later.
For any further questions, feel free to contact us through the chatbot.
Chatbot