Python
Example |
Sending SMSs (Example 1), Sending SMSs in Batches (Example 1) Sending SMSs (Example 2), Sending SMSs in Batches (Example 2) |
---|---|
Environment |
Python 3.7 or later versions are required. The examples are developed based on Python 3.7.0. |
Library |
requests 2.18.1 (only for example 1)
|
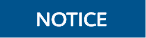
- Sending SMSs shows an example of sending group SMSs with a single template. Sending SMSs in batches shows an example of sending group SMSs with multiple templates.
- The examples described in this document may involve the use of personal data. You are advised to comply with relevant laws and regulations and take measures to ensure that personal data is fully protected.
- The examples described in this document are for demonstration purposes only. Commercial use of the examples is prohibited.
- Information in this document is for your reference only. It does not constitute any offer or commitment.
Sending SMSs (Example 1)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 |
import time import uuid import hashlib import base64 import requests #Run pip install requests to install the dependency. # Mandatory. The values here are example values only. Obtain the actual values based on Development Preparation. url = 'https://smsapi.ap-southeast-1.myhuaweicloud.com:443/sms/batchSendSms/v1' # Application access address and API access URI // Hard-coded or plaintext appKey/appSecret is risky. For security, encrypt your appKey/appSecret and store them in the configuration file or environment variables. APP_KEY = "c8RWg3ggEcyd4D3p94bf3Y7x1Ile" #Application Key APP_SECRET = "q4Ii87Bh************80SfD7Al" #Application Secret sender = "csms12345678" # Channel number for Chinese mainland SMS signature or international SMS TEMPLATE_ID = "8ff55eac1d0b478ab3c06c3c6a492300" # Template ID # Mandatory for Chinese mainland SMS. This parameter is valid and mandatory when Template Type corresponding to templateId is Universal template. The signature name must be approved and be the same type as the template. # This parameter is not required for international SMS. signature = "Huawei Cloud SMS test"; # Signature # Mandatory. Global number format (including the country code), for example, +8615123456789. Use commas (,) to separate multiple numbers. receiver = "+8615123456789,+8615234567890" # Recipient numbers # Optional. Address for receiving SMS status reports. The domain name is recommended. If this parameter is set to an empty value or left unspecified, customers do not receive status reports. statusCallBack = "" ''' Optional. If a template with no variable is used, assign an empty value to this parameter, for example, TEMPLATE_PARAM = ''; Example of a single-variable template: If the template content is "Your verification code is ${NUM_6}", TEMPLATE_PARAM can be set to '["369751"]'. Example of a dual-variable template: If the template content is "You have ${NUM_2} parcels delivered to ${TXT_20}", TEMPLATE_PARAM can be set to '["3","main gate of People's Park"]'. For details, see Template and Variable Specifications. ''' TEMPLATE_PARAM = '["369751"]' # Template variable. The following uses a single-variable verification code SMS message as an example. The customer needs to generate a 6-digit verification code and define it as a character string to prevent the loss of first digits 0 (for example, 002569 is changed to 2569). ''' Construct the value of X-WSSE. @param appKey: string @param appSecret: string @return: string ''' def buildWSSEHeader(appKey, appSecret): now = time.strftime('%Y-%m-%dT%H:%M:%SZ') #Created nonce = str(uuid.uuid4()).replace('-', '') #Nonce digest = hashlib.sha256((nonce + now + appSecret).encode()).hexdigest() digestBase64 = base64.b64encode(digest.encode()).decode() #PasswordDigest return 'UsernameToken Username="{}",PasswordDigest="{}",Nonce="{}",Created="{}"'.format(appKey, digestBase64, nonce, now) def main(): # Request headers header = {'Authorization': 'WSSE realm="SDP",profile="UsernameToken",type="Appkey"', 'X-WSSE': buildWSSEHeader(APP_KEY, APP_SECRET)} # Request body formData = {'from': sender, 'to': receiver, 'templateId': TEMPLATE_ID, 'templateParas': TEMPLATE_PARAM, 'statusCallback': statusCallBack, # 'signature': signature # Uncomment this line if the universal template for Chinese mainland SMS is used. } print(header) # Ignore the certificate trust issues to prevent API calling failures caused by HTTPS certificate authentication failures. r = requests.post(url, data=formData, headers=header, verify=False) print(r.text) #Record the response. if __name__ == '__main__': main() |
Sending SMSs (Example 2)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 |
import time import uuid import hashlib import base64 import ssl import urllib.parse import urllib.request # Mandatory. The values here are example values only. Obtain the actual values based on Development Preparation. url = 'https://smsapi.ap-southeast-1.myhuaweicloud.com:443/sms/batchSendSms/v1' # Application access address and API access URI APP_KEY = "c8RWg3ggEcyd4D3p94bf3Y7x1Ile" #Application Key // Hard-coded or plaintext appKey/appSecret is risky. For security, encrypt your appKey/appSecret and store them in the configuration file or environment variables. APP_SECRET = "q4Ii87Bh************80SfD7Al" #Application Secret sender = "csms12345678" # Channel number for Chinese mainland SMS signature or international SMS TEMPLATE_ID = "8ff55eac1d0b478ab3c06c3c6a492300" # Template ID # Mandatory for Chinese mainland SMS. This parameter is valid and mandatory when Template Type corresponding to templateId is Universal template. The signature name must be approved and be the same type as the template. # This parameter is not required for international SMS. signature = "Huawei Cloud SMS test"; # Signature # Mandatory. Global number format (including the country code), for example, +8615123456789. Use commas (,) to separate multiple numbers. receiver = "+8615123456789,+8615234567890" # Recipient numbers # Optional. Address for receiving SMS status reports. The domain name is recommended. If this parameter is set to an empty value or left unspecified, customers do not receive status reports. statusCallBack = "" ''' Optional. If a template with no variable is used, assign an empty value to this parameter, for example, TEMPLATE_PARAM = ''; Example of a single-variable template: If the template content is "Your verification code is ${NUM_6}", TEMPLATE_PARAM can be set to '["369751"]'. Example of a dual-variable template: If the template content is "You have ${NUM_2} parcels delivered to ${TXT_20}", TEMPLATE_PARAM can be set to '["3","main gate of People's Park"]'. For details, see Template and Variable Specifications. ''' TEMPLATE_PARAM = '["369751"]' # Template variable. The following uses a single-variable verification code SMS message as an example. The customer needs to generate a 6-digit verification code and define it as a character string to prevent the loss of first digits 0 (for example, 002569 is changed to 2569). ''' Construct the value of X-WSSE. @param appKey: string @param appSecret: string @return: string ''' def buildWSSEHeader(appKey, appSecret): now = time.strftime('%Y-%m-%dT%H:%M:%SZ') #Created nonce = str(uuid.uuid4()).replace('-', '') #Nonce digest = hashlib.sha256((nonce + now + appSecret).encode()).hexdigest() digestBase64 = base64.b64encode(digest.encode()).decode() #PasswordDigest return 'UsernameToken Username="{}",PasswordDigest="{}",Nonce="{}",Created="{}"'.format(appKey, digestBase64, nonce, now) def main(): # Request body formData = urllib.parse.urlencode({ 'from': sender, 'to': receiver, 'templateId': TEMPLATE_ID, 'templateParas': TEMPLATE_PARAM, 'statusCallback': statusCallBack, # 'signature': signature # Uncomment this line if the universal template for Chinese mainland SMS is used. }).encode('ascii') req = urllib.request.Request(url=url, data=formData, method='POST') # The request method is POST. # Parameters in the request headers req.add_header('Authorization', 'WSSE realm="SDP",profile="UsernameToken",type="Appkey"') req.add_header('X-WSSE', buildWSSEHeader(APP_KEY, APP_SECRET)) req.add_header('Content-Type', 'application/x-www-form-urlencoded') # Ignore the certificate trust issues to prevent API calling failures caused by HTTPS certificate authentication failures. ssl._create_default_https_context = ssl._create_unverified_context try: r = urllib.request.urlopen(req) # Send a request. print(r.read().decode('utf-8')) # Record the response. except urllib.error.HTTPError as e: print(e.code) print(e.read().decode('utf-8')) except urllib.error.URLError as e: print(e.reason) if __name__ == '__main__': main() |
Sending SMSs in Batches (Example 1)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 |
import time import uuid import hashlib import base64 import requests #Run pip install requests to install the dependency. # Mandatory. The values here are example values only. Obtain the actual values based on Development Preparation. url = 'https://smsapi.ap-southeast-1.myhuaweicloud.com:443/sms/batchSendDiffSms/v1' # Application access address and API access URI // Hard-coded or plaintext appKey/appSecret is risky. For security, encrypt your appKey/appSecret and store them in the configuration file or environment variables. APP_KEY = "c8RWg3ggEcyd4D3p94bf3Y7x1Ile" #Application Key APP_SECRET = "q4Ii87Bh************80SfD7Al" #Application Secret sender = "csms12345678" # Channel number for Chinese mainland SMS signature or international SMS TEMPLATE_ID_1 = "8ff55eac1d0b478ab3c06c3c6a492300" # Template ID 1 TEMPLATE_ID_2 = "8ff55eac1d0b478ab3c06c3c6a492300" # Template ID 2 # Mandatory for Chinese mainland SMS. This parameter is valid and mandatory when Template Type corresponding to templateId is Universal template. The signature name must be approved and be the same type as the template. # This parameter is not required for international SMS. signature_1 = "Huawei Cloud SMS test"; // Signature 1 signature_2 = "Huawei Cloud SMS test"; // Signature 2 # Mandatory. Global number format (including the country code), for example, +8615123456789. Use commas (,) to separate multiple numbers. receiver_1 = ["+8615123456789", "+8615234567890"] # Recipient number of template 1 receiver_2 = ["+8615123456789", "+8615234567890"] # Recipient number of template 2 # Optional. Address for receiving SMS status reports. The domain name is recommended. If this parameter is set to an empty value or left unspecified, customers do not receive status reports. statusCallBack = "" ''' Optional. If a template with no variable is used, assign an empty value to this parameter, for example, TEMPLATE_PARAM = []; Example of a single-variable template: If the template content is "Your verification code is ${NUM_6}", TEMPLATE_PARAM can be set to ["369751"]. Example of a dual-variable template: If the template content is "You have ${NUM_2} parcels delivered to ${TXT_20}", TEMPLATE_PARAM can be set to ["3","main gate of People's Park"]. For details, see Template and Variable Specifications. ''' TEMPLATE_PARAM_1 = ["123456"] # Template 1 variable. The following uses a single-variable verification code SMS message as an example. The customer needs to generate a 6-digit verification code and define it as a character string to prevent the loss of first digits 0 (for example, 002569 is changed to 2569). TEMPLATE_PARAM_2 = ["234567"] # Template 2 variable. The following uses a single-variable verification code SMS message as an example. The customer needs to generate a 6-digit verification code and define it as a character string to prevent the loss of first digits 0 (for example, 002569 is changed to 2569). ''' Construct the value of X-WSSE. @param appKey: string @param appSecret: string @return: string ''' def buildWSSEHeader(appKey, appSecret): now = time.strftime('%Y-%m-%dT%H:%M:%SZ') #Created nonce = str(uuid.uuid4()).replace('-', '') #Nonce digest = hashlib.sha256((nonce + now + appSecret).encode()).hexdigest() digestBase64 = base64.b64encode(digest.encode()).decode() #PasswordDigest return 'UsernameToken Username="{}",PasswordDigest="{}",Nonce="{}",Created="{}"'.format(appKey, digestBase64, nonce, now) def main(): # Request headers header = {'Authorization':'WSSE realm="SDP",profile="UsernameToken",type="Appkey"', 'X-WSSE': buildWSSEHeader(APP_KEY, APP_SECRET)} # Request body jsonData = {'from': sender, 'statusCallback': statusCallBack, 'smsContent':[ {'to':receiver_1, 'templateId':TEMPLATE_ID_1, 'templateParas':TEMPLATE_PARAM_1, # 'signature':signature_1 # Uncomment this line if the universal template for Chinese mainland SMS is used. }, {'to':receiver_2, 'templateId':TEMPLATE_ID_2, 'templateParas':TEMPLATE_PARAM_2, # 'signature':signature_2 # Uncomment this line if the universal template for Chinese mainland SMS is used. }] } print(header) # Ignore the certificate trust issues to prevent API calling failures caused by HTTPS certificate authentication failures. r = requests.post(url, json=jsonData, headers=header, verify=False) print(r.text) # Record the response. if __name__ == '__main__': main() |
Sending SMSs in Batches (Example 2)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 |
import time import uuid import hashlib import base64 import json import ssl import urllib.request # Mandatory. The values here are example values only. Obtain the actual values based on Development Preparation. url = 'https://smsapi.ap-southeast-1.myhuaweicloud.com:443/sms/batchSendDiffSms/v1' # Application access address and API access URI // Hard-coded or plaintext appKey/appSecret is risky. For security, encrypt your appKey/appSecret and store them in the configuration file or environment variables. APP_KEY = "c8RWg3ggEcyd4D3p94bf3Y7x1Ile" #Application Key APP_SECRET = "q4Ii87Bh************80SfD7Al" #Application Secret sender = "csms12345678" # Channel number for Chinese mainland SMS signature or international SMS TEMPLATE_ID_1 = "8ff55eac1d0b478ab3c06c3c6a492300" # Template ID 1 TEMPLATE_ID_2 = "8ff55eac1d0b478ab3c06c3c6a492300" # Template ID 2 # Mandatory for Chinese mainland SMS. This parameter is valid and mandatory when Template Type corresponding to templateId is Universal template. The signature name must be approved and be the same type as the template. # This parameter is not required for international SMS. signature_1 = "Huawei Cloud SMS test"; // Signature 1 signature_2 = "Huawei Cloud SMS test"; // Signature 2 # Mandatory. Global number format (including the country code), for example, +8615123456789. Use commas (,) to separate multiple numbers. receiver_1 = ["+8615123456789", "+8615234567890"] # Recipient number of template 1 receiver_2 = ["+8615123456789", "+8615234567890"] # Recipient number of template 2 # Optional. Address for receiving SMS status reports. The domain name is recommended. If this parameter is set to an empty value or left unspecified, customers do not receive status reports. statusCallBack = "" ''' Optional. If a template with no variable is used, assign an empty value to this parameter, for example, TEMPLATE_PARAM = []; Example of a single-variable template: If the template content is "Your verification code is ${NUM_6}", TEMPLATE_PARAM can be set to ["369751"]. Example of a dual-variable template: If the template content is "You have ${NUM_2} parcels delivered to ${TXT_20}", TEMPLATE_PARAM can be set to ["3","main gate of People's Park"]. For details, see Template and Variable Specifications. ''' TEMPLATE_PARAM_1 = ["123456"] # Template 1 variable. The following uses a single-variable verification code SMS message as an example. The customer needs to generate a 6-digit verification code and define it as a character string to prevent the loss of first digits 0 (for example, 002569 is changed to 2569). TEMPLATE_PARAM_2 = ["234567"] # Template 2 variable. The following uses a single-variable verification code SMS message as an example. The customer needs to generate a 6-digit verification code and define it as a character string to prevent the loss of first digits 0 (for example, 002569 is changed to 2569). ''' Construct the value of X-WSSE. @param appKey: string @param appSecret: string @return: string ''' def buildWSSEHeader(appKey, appSecret): now = time.strftime('%Y-%m-%dT%H:%M:%SZ') #Created nonce = str(uuid.uuid4()).replace('-', '') #Nonce digest = hashlib.sha256((nonce + now + appSecret).encode()).hexdigest() digestBase64 = base64.b64encode(digest.encode()).decode() #PasswordDigest return 'UsernameToken Username="{}",PasswordDigest="{}",Nonce="{}",Created="{}"'.format(appKey, digestBase64, nonce, now) def main(): # Request body jsonData = json.dumps({'from': sender, 'statusCallback': statusCallBack, 'smsContent':[ {'to':receiver_1, 'templateId':TEMPLATE_ID_1, 'templateParas':TEMPLATE_PARAM_1, # 'signature':signature_1 # Uncomment this line if the universal template for Chinese mainland SMS is used. }, {'to':receiver_2, 'templateId':TEMPLATE_ID_2, 'templateParas':TEMPLATE_PARAM_2, # 'signature':signature_2 # Uncomment this line if the universal template for Chinese mainland SMS is used. }] }).encode('ascii') req = urllib.request.Request(url=url, data=jsonData, method='POST') #The request method is POST. # Parameters in the request headers req.add_header('Authorization', 'WSSE realm="SDP",profile="UsernameToken",type="Appkey"') req.add_header('X-WSSE', buildWSSEHeader(APP_KEY, APP_SECRET)) req.add_header('Content-Type', 'application/json') # Ignore the certificate trust issues to prevent API calling failures caused by HTTPS certificate authentication failures. ssl._create_default_https_context = ssl._create_unverified_context try: r = urllib.request.urlopen(req) # Send a request. print(r.read().decode('utf-8')) # Record the response. except urllib.error.HTTPError as e: print(e.code) print(e.read().decode('utf-8')) except urllib.error.URLError as e: print(e.reason) if __name__ == '__main__': main() |
Receiving Status Reports
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
import urllib.parse # Data example (urlencode) of the status report reported by the SMS platform #success_body = "sequence=1&total=1&updateTime=2018-10-31T08%3A43%3A41Z&source=2&smsMsgId=2ea20735-f856-4376-afbf-570bd70a46ee_11840135&status=DELIVRD"; failed_body = "orgCode=E200027&sequence=1&total=1&updateTime=2018-10-31T08%3A43%3A41Z&source=2&smsMsgId=2ea20735-f856-4376-afbf-570bd70a46ee_11840135&status=RTE_ERR"; ''' Parse the status report data. @param data: Status report data reported by the SMS platform. @return: ''' def onSmsStatusReport(data): keyValues = urllib.parse.parse_qs(data); # Parse the status report data. ''' Example: Parsing status is used as an example. 'smsMsgId': Unique ID of an SMS 'total': Number of SMS segments 'sequence': Sequence number of an SMS after splitting 'source': Status report source 'updateTime': Resource update time 'status': Enumeration values of the status report 'orgCode': Status code ''' status = keyValues.get('status'); # Enumerated values of the status report # Check whether the SMS is successfully sent based on the value of status. if 'DELIVRD' == str.upper(status[0]): print('Send sms success. smsMsgId: ', keyValues.get('smsMsgId')[0]); else: # The SMS fails to be sent. The values of status and orgCode are recorded. print('Send sms failed. smsMsgId: ', keyValues.get('smsMsgId')[0]); print('Failed status: ', status[0]); print('Failed orgCode: ', keyValues.get('orgCode')[0]); if __name__ == '__main__': # onSmsStatusReport(success_body) onSmsStatusReport(failed_body) |
References
- Code examples: Java, PHP, C#, Node.js, and Go
- APIs: SMS Sending API, Batch SMS Sending API, Status Report Receiving API
- Troubleshooting Commissioning Problems
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
For any further questions, feel free to contact us through the chatbot.
Chatbot