Updated on 2023-11-17 GMT+08:00
Java
Example |
|
---|---|
Environment |
JDK 1.6 or later |
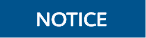
- Sending SMSs shows an example of sending group SMSs with a single template. Sending SMSs in batches shows an example of sending group SMSs with multiple templates.
- The examples described in this document may involve the use of personal data. You are advised to comply with relevant laws and regulations and take measures to ensure that personal data is fully protected.
- The examples described in this document are for demonstration purposes only. Commercial use of the examples is prohibited.
- Information in this document is for your reference only. It does not constitute any offer or commitment.
Sending SMSs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 |
import java.io.BufferedReader;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStreamWriter;
import java.io.UnsupportedEncodingException;
import java.io.Writer;
import java.net.URL;
import java.net.URLEncoder;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.security.cert.CertificateException;
import java.security.cert.X509Certificate;
import java.text.SimpleDateFormat;
// If the JDK version is 1.8, use the native Base64 class.
import java.util.Base64;
import java.util.Date;
import java.util.HashMap;
import java.util.Map;
import java.util.UUID;
import javax.net.ssl.HostnameVerifier;
import javax.net.ssl.HttpsURLConnection;
import javax.net.ssl.SSLContext;
import javax.net.ssl.SSLSession;
import javax.net.ssl.TrustManager;
import javax.net.ssl.X509TrustManager;
// If the JDK version is earlier than 1.8, use a third-party library to provide the Base64 class.
//import org.apache.commons.codec.binary.Base64;
public class SendSms {
// This parameter does not need to be modified. It is used to format the Authentication header field and assign a value to the X-WSSE parameter.
private static final String WSSE_HEADER_FORMAT = "UsernameToken Username=\"%s\",PasswordDigest=\"%s\",Nonce=\"%s\",Created=\"%s\"";
// This parameter does not need to be modified. It is used to format the Authentication header field and assign a value to the Authorization parameter.
private static final String AUTH_HEADER_VALUE = "WSSE realm=\"SDP\",profile=\"UsernameToken\",type=\"Appkey\"";
public static void main(String[] args) throws Exception {
// Mandatory. Obtain the actual values by referring to to replace the following example values.
String url = "https://smsapi.ap-southeast-1.myhuaweicloud.com:443/sms/batchSendSms/v1"; // Application access address and API access URI
// Hard-coded or plaintext appKey/appSecret is risky. For security, encrypt your appKey/appSecret and store them in the configuration file or environment variables.
String appKey = "c8RWg3ggEcyd4D3p94bf3Y7x1Ile"; //Application Key
String appSecret = "q4Ii87Bh************80SfD7Al"; //Application Secret
String sender = "csms12345678"; // Channel number for Chinese mainland SMS or international SMS
String templateId = "8ff55eac1d0b478ab3c06c3c6a492300"; // Template ID
// Mandatory for Chinese mainland SMS. This parameter is valid and mandatory when Template Type corresponding to templateId is Universal template. The signature name must be approved and be the same type as the template.
// This parameter is not required for international SMS.
String signature = "Huawei Cloud SMS test"; // Signature
// Mandatory. Global number format (including the country code), for example, +8615123456789. Use commas (,) to separate multiple numbers.
String receiver = "+8615123456789,+8615234567890"; // Recipient numbers
// Optional. Address for receiving SMS status reports. The domain name is recommended. If this parameter is set to an empty value or left unspecified, customers do not receive status reports.
String statusCallBack = "";
/**
* Optional. If a non-variable template is used, assign an empty value to this parameter, for example, String templateParas = "";
* Example of a single-variable template: If the template content is "Your verification code is ${NUM_6}", templateParas can be set to "[\"369751\"]".
* Example of a dual-variable template: If the template content is "You have ${NUM_2} delivered to ${TXT_20}", templateParas can be set to "[\"3\",\"main gate of People's Park\"]".
* To view more information, choose Service Overview > Template and Variable Specifications.
*/
String templateParas = "[\"369751\"]"; // Template variable. The following uses a single-variable verification code SMS message as an example. The customer needs to generate a 6-digit verification code and define it as a character string to prevent the loss of first digits 0 (for example, 002569 is changed to 2569).
// Request body. If the signature name is not required, set signature to null.
String body = buildRequestBody(sender, receiver, templateId, templateParas, statusCallBack, signature);
if (null == body || body.isEmpty()) {
System.out.println("body is null.");
return;
}
// The value of X-WSSE in the request headers is as follows:
String wsseHeader = buildWsseHeader(appKey, appSecret);
if (null == wsseHeader || wsseHeader.isEmpty()) {
System.out.println("wsse header is null.");
return;
}
Writer out = null;
BufferedReader in = null;
StringBuffer result = new StringBuffer();
HttpsURLConnection connection = null;
InputStream is = null;
// Ignore the certificate trust issues to prevent API calling failures caused by HTTPS certificate authentication failures.
HostnameVerifier hv = new HostnameVerifier() {
@Override
public boolean verify(String hostname, SSLSession session) {
return true;
}
};
trustAllHttpsCertificates();
try {
URL realUrl = new URL(url);
connection = (HttpsURLConnection) realUrl.openConnection();
connection.setHostnameVerifier(hv);
connection.setDoOutput(true);
connection.setDoInput(true);
connection.setUseCaches(true);
// Request method
connection.setRequestMethod("POST");
// Parameters in the request headers
connection.setRequestProperty("Content-Type", "application/x-www-form-urlencoded");
connection.setRequestProperty("Authorization", AUTH_HEADER_VALUE);
connection.setRequestProperty("X-WSSE", wsseHeader);
connection.connect();
out = new OutputStreamWriter(connection.getOutputStream());
out.write(body); // Parameters in the request body
out.flush();
out.close();
int status = connection.getResponseCode();
if (200 == status) { //200
is = connection.getInputStream();
} else { //400/401
is = connection.getErrorStream();
}
in = new BufferedReader(new InputStreamReader(is, "UTF-8"));
String line = "";
while ((line = in.readLine()) != null) {
result.append(line);
}
System.out.println(result.toString()); // Record the response entity.
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (null != out) {
out.close();
}
if (null != is) {
is.close();
}
if (null != in) {
in.close();
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
/**
* Construct the request body.
* @param sender
* @param receiver
* @param templateId
* @param templateParas
* @param statusCallBack
* @param signature | Signature name, which must be specified when the universal template for Chinese mainland SMS is used.
* @return
*/
static String buildRequestBody(String sender, String receiver, String templateId, String templateParas,
String statusCallBack, String signature) {
if (null == sender || null == receiver || null == templateId || sender.isEmpty() || receiver.isEmpty()
|| templateId.isEmpty()) {
System.out.println("buildRequestBody(): sender, receiver or templateId is null.");
return null;
}
Map<String, String> map = new HashMap<String, String>();
map.put("from", sender);
map.put("to", receiver);
map.put("templateId", templateId);
if (null != templateParas && !templateParas.isEmpty()) {
map.put("templateParas", templateParas);
}
if (null != statusCallBack && !statusCallBack.isEmpty()) {
map.put("statusCallback", statusCallBack);
}
if (null != signature && !signature.isEmpty()) {
map.put("signature", signature);
}
StringBuilder sb = new StringBuilder();
String temp = "";
for (String s : map.keySet()) {
try {
temp = URLEncoder.encode(map.get(s), "UTF-8");
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
sb.append(s).append("=").append(temp).append("&");
}
return sb.deleteCharAt(sb.length()-1).toString();
}
/**
* Construct the value of X-WSSE.
* @param appKey
* @param appSecret
* @return
*/
static String buildWsseHeader(String appKey, String appSecret) {
if (null == appKey || null == appSecret || appKey.isEmpty() || appSecret.isEmpty()) {
System.out.println("buildWsseHeader(): appKey or appSecret is null.");
return null;
}
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd'T'HH:mm:ss'Z'");
String time = sdf.format(new Date()); //Created
String nonce = UUID.randomUUID().toString().replace("-", ""); //Nonce
MessageDigest md;
byte[] passwordDigest = null;
try {
md = MessageDigest.getInstance("SHA-256");
md.update((nonce + time + appSecret).getBytes());
passwordDigest = md.digest();
} catch (NoSuchAlgorithmException e) {
e.printStackTrace();
}
// If the JDK version is 1.8, load the native Base64 class and use the following code:
String passwordDigestBase64Str = Base64.getEncoder().encodeToString(passwordDigest); //PasswordDigest
// If the JDK version is earlier than 1.8, load a third-party library to provide the Base64 class and use the following code:
//String passwordDigestBase64Str = Base64.encodeBase64String(passwordDigest); //PasswordDigest
// If passwordDigestBase64Str contains newline characters, run the following code:
//passwordDigestBase64Str = passwordDigestBase64Str.replaceAll("[\\s*\t\n\r]", "");
return String.format(WSSE_HEADER_FORMAT, appKey, passwordDigestBase64Str, nonce, time);
}
/**
* Ignore the certificate trust issues.
* @throws Exception
*/
static void trustAllHttpsCertificates() throws Exception {
TrustManager[] trustAllCerts = new TrustManager[] {
new X509TrustManager() {
public void checkClientTrusted(X509Certificate[] chain, String authType) throws CertificateException {
return;
}
public void checkServerTrusted(X509Certificate[] chain, String authType) throws CertificateException {
return;
}
public X509Certificate[] getAcceptedIssuers() {
return null;
}
}
};
SSLContext sc = SSLContext.getInstance("SSL");
sc.init(null, trustAllCerts, null);
HttpsURLConnection.setDefaultSSLSocketFactory(sc.getSocketFactory());
}
}
|
Sending SMSs in Batches
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 |
import java.io.BufferedReader;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.OutputStreamWriter;
import java.io.Writer;
import java.net.URL;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.security.cert.CertificateException;
import java.security.cert.X509Certificate;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
// If the JDK version is 1.8, use the native Base64 class.
import java.util.Base64;
import java.util.Date;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.UUID;
import javax.net.ssl.HostnameVerifier;
import javax.net.ssl.HttpsURLConnection;
import javax.net.ssl.SSLContext;
import javax.net.ssl.SSLSession;
import javax.net.ssl.TrustManager;
import javax.net.ssl.X509TrustManager;
// If the JDK version is earlier than 1.8, use a third-party library to provide the Base64 class.
//import org.apache.commons.codec.binary.Base64;
import org.json.JSONArray;
import org.json.JSONObject;
public class SendDiffSms {
// This parameter does not need to be modified. It is used to format the Authentication header field and assign a value to the X-WSSE parameter.
private static final String WSSE_HEADER_FORMAT = "UsernameToken Username=\"%s\",PasswordDigest=\"%s\",Nonce=\"%s\",Created=\"%s\"";
// This parameter does not need to be modified. It is used to format the Authentication header field and assign a value to the Authorization parameter.
private static final String AUTH_HEADER_VALUE = "WSSE realm=\"SDP\",profile=\"UsernameToken\",type=\"Appkey\"";
public static void main(String[] args) throws Exception {
// Mandatory. The values here are example values only. Obtain the values based on Development Preparation.
String url = "https://smsapi.ap-southeast-1.myhuaweicloud.com:443/sms/batchSendDiffSms/v1"; // Application access address and API access URI
// Hard-coded or plaintext appKey/appSecret is risky. For security, encrypt your appKey/appSecret and store them in the configuration file or environment variables.
String appKey = "c8RWg3ggEcyd4D3p94bf3Y7x1Ile"; //Application Key
String appSecret = "q4Ii87Bh************80SfD7Al"; //Application Secret
String sender = "csms12345678"; // Channel number for Chinese mainland SMS or international SMS
String templateId1 = "8ff55eac1d0b478ab3c06c3c6a492300"; // Template ID 1
String templateId2 = "8ff55eac1d0b478ab3c06c3c6a492300"; // Template ID 2
// Mandatory for Chinese mainland SMS. This parameter is valid and mandatory when Template Type corresponding to templateId is Universal template. The signature name must be approved and be the same type as the template.
// This parameter is not required for international SMS.
String signature1 = "Huawei Cloud SMS test"; // Signature 1
String signature2 = "Huawei Cloud SMS test"; // Signature 2
// Mandatory. Global number format (including the country code), for example, +8615123456789. Use commas (,) to separate multiple numbers.
String[] receiver1 = {"+8615123456789", "+8615234567890"}; // Recipient number of template 1
String[] receiver2 = {"+8615123456789", "+8615234567890"}; // Recipient number of template 2
// Optional. Address for receiving SMS status reports. The domain name is recommended. If this parameter is set to an empty value or left unspecified, customers do not receive status reports.
String statusCallBack = "";
/**
* Optional. If a non-variable template is used, assign an empty value to this parameter, for example, String templateParas = {};
* Example of a single-variable template: If the template content is "Your verification code is ${NUM_6}", templateParas can be set to {"369751"}.
* Example of a dual-variable template: If the template content is "You have ${NUM_2} parcels delivered to ${TXT_20}", templateParas can be set to {"3","main gate of People's Park"}.
* To view more information, choose Service Overview > Template and Variable Specifications.
*/
String[] templateParas1 = {"123456"}; // Template 1 variable. The following uses a single-variable verification code SMS message as an example. The customer needs to generate a 6-digit verification code and define it as a character string to prevent the loss of first digits 0 (for example, 002569 is changed to 2569).
String[] templateParas2 = {"234567"}; // Template 2 variable. The following uses a single-variable verification code SMS message as an example. The customer needs to generate a 6-digit verification code and define it as a character string to prevent the loss of first digits 0 (for example, 002569 is changed to 2569).
// smsContent. If the signature name is not required, set signature to null.
List<Map<String, Object>> smsContent = new ArrayList<Map<String, Object>>();
Map<String, Object> item1 = initDiffSms(receiver1, templateId1, templateParas1, signature1);
Map<String, Object> item2 = initDiffSms(receiver2, templateId2, templateParas2, signature2);
if (null != item1 && !item1.isEmpty()) {
smsContent.add(item1);
}
if (null != item2 && !item2.isEmpty()) {
smsContent.add(item2);
}
// Request body
String body = buildRequestBody(sender, smsContent, statusCallBack);
if (null == body || body.isEmpty()) {
System.out.println("body is null.");
return;
}
// The value of X-WSSE in the request headers is as follows:
String wsseHeader = buildWsseHeader(appKey, appSecret);
if (null == wsseHeader || wsseHeader.isEmpty()) {
System.out.println("wsse header is null.");
}
Writer out = null;
BufferedReader in = null;
StringBuffer result = new StringBuffer();
HttpsURLConnection connection = null;
InputStream is = null;
// Ignore the certificate trust issues to prevent API calling failures caused by HTTPS certificate authentication failures.
HostnameVerifier hv = new HostnameVerifier() {
@Override
public boolean verify(String hostname, SSLSession session) {
return true;
}
};
trustAllHttpsCertificates();
try {
URL realUrl = new URL(url);
connection = (HttpsURLConnection) realUrl.openConnection();
connection.setHostnameVerifier(hv);
connection.setDoOutput(true);
connection.setDoInput(true);
connection.setUseCaches(true);
// Request method
connection.setRequestMethod("POST");
// Parameters in the request headers
connection.setRequestProperty("Content-Type", "application/json");
connection.setRequestProperty("Authorization", AUTH_HEADER_VALUE);
connection.setRequestProperty("X-WSSE", wsseHeader);
connection.connect();
out = new OutputStreamWriter(connection.getOutputStream());
out.write(body); // Parameters in the request body
out.flush();
out.close();
int status = connection.getResponseCode();
if (200 == status) { //200
is = connection.getInputStream();
} else { //400/401
is = connection.getErrorStream();
}
in = new BufferedReader(new InputStreamReader(is, "UTF-8"));
String line = "";
while ((line = in.readLine()) != null) {
result.append(line);
}
System.out.println(result.toString()); // Record the response entity.
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (null != out) {
out.close();
}
if (null != is) {
is.close();
}
if (null != in) {
in.close();
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
/**
* Construct the value of smsContent.
* @param receiver
* @param templateId
* @param templateParas
* @param signature | Signature name, which must be specified when the universal template for Chinese mainland SMS is used.
* @return
*/
static Map<String, Object> initDiffSms(String[] receiver, String templateId, String[] templateParas,
String signature) {
if (null == receiver || null == templateId || receiver.length == 0 || templateId.isEmpty()) {
System.out.println("initDiffSms(): receiver or templateId is null.");
return null;
}
Map<String, Object> map = new HashMap<String, Object>();
map.put("to", receiver);
map.put("templateId", templateId);
if (null != templateParas && templateParas.length > 0) {
map.put("templateParas", templateParas);
}
if (null != signature && !signature.isEmpty()) {
map.put("signature", signature);
}
return map;
}
/**
* Construct the request body.
* @param sender
* @param smsContent
* @param statusCallBack
* @return
*/
static String buildRequestBody(String sender, List<Map<String, Object>> smsContent,
String statusCallBack) {
if (null == sender || null == smsContent || sender.isEmpty() || smsContent.isEmpty()) {
System.out.println("buildRequestBody(): sender or smsContent is null.");
return null;
}
JSONArray jsonArr = new JSONArray();
for(Map<String, Object> it: smsContent){
jsonArr.put(it);
}
Map<String, Object> data = new HashMap<String, Object>();
data.put("from", sender);
data.put("smsContent", jsonArr);
if (null != statusCallBack && !statusCallBack.isEmpty()) {
data.put("statusCallback", statusCallBack);
}
return new JSONObject(data).toString();
}
static String buildWsseHeader(String appKey, String appSecret) {
if (null == appKey || null == appSecret || appKey.isEmpty() || appSecret.isEmpty()) {
System.out.println("buildWsseHeader(): appKey or appSecret is null.");
return null;
}
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd'T'HH:mm:ss'Z'");
String time = sdf.format(new Date()); //Created
String nonce = UUID.randomUUID().toString().replace("-", ""); //Nonce
MessageDigest md;
byte[] passwordDigest = null;
try {
md = MessageDigest.getInstance("SHA-256");
md.update((nonce + time + appSecret).getBytes());
passwordDigest = md.digest();
} catch (NoSuchAlgorithmException e) {
e.printStackTrace();
}
// If the JDK version is 1.8, load the native Base64 class and use the following code:
String passwordDigestBase64Str = Base64.getEncoder().encodeToString(passwordDigest); //PasswordDigest
// If the JDK version is earlier than 1.8, load a third-party library to provide the Base64 class and use the following code:
//String passwordDigestBase64Str = Base64.encodeBase64String(passwordDigest); //PasswordDigest
// If passwordDigestBase64Str contains newline characters, run the following code:
//passwordDigestBase64Str = passwordDigestBase64Str.replaceAll("[\\s*\t\n\r]", "");
return String.format(WSSE_HEADER_FORMAT, appKey, passwordDigestBase64Str, nonce, time);
}
/**
* Ignore the certificate trust issues.
* @throws Exception
*/
static void trustAllHttpsCertificates() throws Exception {
TrustManager[] trustAllCerts = new TrustManager[] {
new X509TrustManager() {
public void checkClientTrusted(X509Certificate[] chain, String authType) throws CertificateException {
return;
}
public void checkServerTrusted(X509Certificate[] chain, String authType) throws CertificateException {
return;
}
public X509Certificate[] getAcceptedIssuers() {
return null;
}
}
};
SSLContext sc = SSLContext.getInstance("SSL");
sc.init(null, trustAllCerts, null);
HttpsURLConnection.setDefaultSSLSocketFactory(sc.getSocketFactory());
}
}
|
Receiving Status Reports
The Maven dependency to be introduced is org.springframework:spring-web:5.3.21 (example version).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class DemoController {
/**
* Synchronize SMS receipts.
*/
@PostMapping("/report")
public void smsHwReport(@RequestParam String smsMsgId, // Unique SMS identifier returned after an SMS is successfully sent.
@RequestParam(required = false) String total, // Number of SMS segments split from a long SMS message. If the SMS message is not split, set this parameter to 1.
@RequestParam(required = false) String sequence, // Sequence number after a long SMS is split. This parameter is valid only when the value of total is greater than 1. If the SMS is not split, set this parameter to 1.
@RequestParam String status, // Enumerated values of an SMS status report. For details about the values, see API Reference.
@RequestParam(required = false) String source, // Source of the SMS status report. 1: generated by the SMS platform. 2: returned by the SMSC. 3: generated by the cloud platform.
@RequestParam(required = false) String updateTime,// SMS resource update time, which is generally the UTC time when the Message & SMS platform receives the SMS status report. The value is in the format of yyyy-MM-dd'T'HH:mm:ss'Z'. The time is converted to %3a using urlencode. // When the Message & SMS platform does not receive the SMS status report from the SMSC, the platform constructs a status report that does not contain the updateTime parameter.
@RequestParam(required = false) String orgCode, // Status codes of southbound NEs are transparently transmitted. This parameter is contained only in status reports of international SMSs. // When the status code is not returned, the parameter is not used.
@RequestParam(required = false) String extend, // Extended field in the request sent by a user. If the SMS sent by a user does not carry the extend parameter, the status report does not contain the extend parameter.
@RequestParam(required = false) String to) { // Recipient number of the SMS corresponding to the status report. This parameter is carried only when the status report contains the extend parameter.
System.out.println(" ================receive smsStatusReport ======================");
System.out.println("smsMsgId: " + smsMsgId);
System.out.println("total: " + total);
System.out.println("sequence: " + sequence);
System.out.println("status: " + status);
System.out.println("source: " + source);
System.out.println("updateTime: " + updateTime);
System.out.println("orgCode: " + orgCode);
System.out.println("extend: " + extend);
System.out.println("to: " + to);
}
}
|
References
- Code examples: PHP, Python, C#, Node.js, and Go
- APIs: SMS Sending API, Batch SMS Sending API, Status Report Receiving API
- Troubleshooting Commissioning Problems
Parent topic: X-WSSE Authentication
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.See the reply and handling status in My Cloud VOC.
The system is busy. Please try again later.
For any further questions, feel free to contact us through the chatbot.
Chatbot