Generating Authentication Parameters for Browser-Based Uploads (SDK for Python)
Function
This API generates parameters for authentication. The parameters can be used to perform a browser-based upload through POST operations.
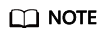
There are two request parameters generated for authentication:
- policy, which corresponds to the policy parameter in the form.
- signature, which corresponds to the signature parameter in the form.
Restrictions
- To upload an object, you must be the bucket owner or have the required permission (obs:object:PutObject in IAM or PutObject in a bucket policy). For details, see Introduction to OBS Access Control, IAM Custom Policies, and Configuring an Object Policy.
Method
ObsClient.createPostSignature(bucketName, objectKey, expires, formParams)
Request Parameters
Parameter |
Type |
Mandatory (Yes/No) |
Description |
---|---|---|---|
bucketName |
str |
No |
Explanation: Bucket name Restrictions:
Default value: None |
objectKey |
str |
No |
Explanation: Object name. An object is uniquely identified by an object name in a bucket. An object name is a complete path that does not contain the bucket name. For example, if the address for accessing the object is examplebucket.obs.eu-west-101.myhuaweicloud.eu/folder/test.txt, the object name is folder/test.txt. Value range: The value must contain 1 to 1,024 characters. Default value: None |
expires |
int |
No |
Explanation: Expiration time of authentication for a browser-based upload Value range: An integer greater than or equal to 0, in seconds Default value: 300 |
formParams |
dict |
No |
Explanation: Parameters of browser-based uploads, not including key, policy, and signature. Value range:
Default value: None |
Responses
Parameter |
Type |
Description |
---|---|---|
originPolicy |
str |
Explanation: policy not encoded by Base64. This parameter can only be used for verification. Example: {"expiration":"2023-09-12T12:52:59Z","conditions":[{"content-type":"text/plain"},{"bucket":"examplebucket"},{"key":"example/objectname"},]}" Default value: None |
policy |
str |
Explanation: Base64-encoded value of policy in the form Example: eyJleHBpcmF0aW9uIjoiMjAyMy0wOS0xMlQxMjo1Mjo1OVoiLCJjb25kaXRpb25zIjpbeyJjb250ZW50LXR5cGUiOiJ0ZXh0L3BsYWluIn0seyJidWNrZXQiOiJleGFtcGxlYnVja2V0In0seyJrZXkiOiJleGFtcGxlL29iamVjdG5hbWUifSxdfQ== Default value: None |
signature |
str |
Explanation: signature in the form Example: g0jQr4v9VWd1Q2FOFDG6LGfV9Cw= Default value: None |
Code Examples
This example generates authentication parameters policy and signature for a browser-based upload.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
from obs import ObsClient import os import traceback # Obtain an AK and SK pair using environment variables or import the AK and SK pair in other ways. Using hard coding may result in leakage. # Obtain an AK and SK pair on the management console. For details, see https://support.huaweicloud.com/eu/usermanual-ca/ca_01_0003.html. ak = os.getenv("AccessKeyID") sk = os.getenv("SecretAccessKey") # (Optional) If you use a temporary AK and SK pair and a security token to access OBS, obtain them from environment variables. security_token = os.getenv("SecurityToken") # Set server to the endpoint corresponding to the bucket. Here uses EU-Dublin as an example. Replace it with the one in use. server = "https://obs.eu-west-101.myhuaweicloud.eu" # Create an obsClient instance. # If you use a temporary AK and SK pair and a security token to access OBS, you must specify security_token when creating an instance. obsClient = ObsClient(access_key_id=ak, secret_access_key=sk, server=server) try: bucketName = "examplebucket" objectKey = "objectname" # Configure the validity period (in seconds) for a browser-based upload request. 3600 is used as an example. expires = 3600 # Specify parameters for a browser-based upload except key, policy, and signature. In this example, x-obs-acl is set to private and content-type is set to text/plain. formParams = {'x-obs-acl': 'private', 'content-type': 'text/plain'} # Create parameters for a browser-based upload. resp = obsClient.createPostSignature(bucketName, objectKey, expires, formParams) print('originPolicy:', resp.originPolicy) print('policy:', resp.policy) print('signature:', resp.signature) except: print(traceback.format_exc()) |
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.