Listing Buckets (SDK for Node.js)
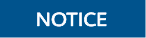
If you have any questions during development, post them on the Issues page of GitHub.
Function
OBS buckets are containers for storing objects you upload to OBS. This API returns a list of all buckets that meet the specified conditions in all regions of the current account. Returned buckets are listed in alphabetical order by bucket name.
Restrictions
- To list buckets, you must have the obs:bucket:ListAllMyBuckets permission. IAM is recommended for granting permissions. For details, see IAM Custom Policies.
Method
ObsClient.listBuckets(params)
Request Parameters
Parameter |
Type |
Mandatory (Yes/No) |
Description |
---|---|---|---|
QueryLocation |
boolean |
No |
Explanation: Whether to query the bucket location Restrictions: None Value range:
Default value: false |
Constant |
Description |
---|---|
OBJECT |
An object bucket |
POSIX |
A parallel file system (POSIX) |
Responses
Type |
Description |
---|---|
NOTE:
This API returns a Promise response, which requires the Promise or async/await syntax. |
Explanation: Returned results. For details, see Table 4. |
Parameter |
Type |
Description |
---|---|---|
CommonMsg |
Explanation: Common information generated after an API call is complete, including the HTTP status code and error code. For details, see Table 5. |
|
InterfaceResult |
Explanation: Results outputted for a successful call. For details, see Table 6. Restrictions: This parameter is not included if the value of Status is greater than 300. |
Parameter |
Type |
Description |
Status |
number |
Explanation: HTTP status code returned by the OBS server. Value range: A status code is a group of digits indicating the status of a response. It ranges from 2xx (indicating successes) to 4xx or 5xx (indicating errors). For details, see Status Codes. |
Code |
string |
Explanation: Error code returned by the OBS server. |
Message |
string |
Explanation: Error description returned by the OBS server. |
HostId |
string |
Explanation: Request server ID returned by the OBS server. |
RequestId |
string |
Explanation: Request ID returned by the OBS server. |
Id2 |
string |
Explanation: Request ID2 returned by the OBS server. |
Indicator |
string |
Explanation: Error code details returned by the OBS server. |
Parameter |
Type |
Description |
---|---|---|
RequestId |
string |
Explanation: Request ID returned by the OBS server Default value: None |
Owner |
Explanation: Bucket owner Value range: See Table 7. |
|
Buckets |
Bucket[] |
Explanation: Bucket information list Value range: See Table 8. |
Parameter |
Type |
Mandatory (Yes/No) |
Description |
---|---|---|---|
ID |
string |
Yes if used as a request parameter |
Explanation: Account (domain) ID of the owner Value range: To obtain the account ID, see How Do I Get My Account ID and User ID? (SDK for Node.js). Default value: None |
DisplayName |
string |
No |
Explanation: Account name of the owner Default value: None |
Parameter |
Type |
Description |
---|---|---|
BucketName |
string |
Explanation: Bucket name. Restrictions:
Default value: None |
CreationDate |
string |
Explanation: When the bucket was created. Default value: None |
Location |
string |
Explanation: Where a bucket is located. Restrictions: If the endpoint used is obs.myhuaweicloud.eu, this parameter is not required. If any other endpoints are used, this parameter is required. Default value: If obs.myhuaweicloud.eu is used as the endpoint and no region is specified, eu-west-101 (the EU-Dublin region) is used by default. |
Code Examples
You can call ObsClient.listBuckets to list buckets. This example lists all buckets.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 |
// Import the OBS library. // Use npm to install the client. const ObsClient = require("esdk-obs-nodejs"); // Use the source code to install the client. // var ObsClient = require('./lib/obs'); // Create an instance of ObsClient. const obsClient = new ObsClient({ // Obtain an AK/SK pair using environment variables or import an AK/SK pair in other ways. Using hard coding may result in leakage. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/eu/usermanual-ca/ca_01_0003.html. access_key_id: process.env.ACCESS_KEY_ID, secret_access_key: process.env.SECRET_ACCESS_KEY, // (Optional) If you use a temporary AK/SK pair and a security token to access OBS, you are advised not to use hard coding, which may result in information leakage. You can obtain an AK/SK pair using environment variables or import an AK/SK pair in other ways. // security_token: process.env.SECURITY_TOKEN, // Enter the endpoint corresponding to the region where the bucket is located. EU-Dublin is used here in this example. Replace it with the one currently in use. server: "https://obs.eu-west-101.myhuaweicloud.eu" }); async function listBuckets() { try { const params = { // Specify whether to query the bucket location in the listing. The default value is false. true is used in this example. QueryLocation: true, // Specify a bucket type (object buckets in this example). If this parameter is not specified, object buckets and parallel file systems are listed by default. BucketType: "OBJECT", }; // List buckets. const result = await obsClient.listBuckets(params); if (result.CommonMsg.Status <= 300) { console.log("List buckets successful!", params.Bucket); console.log("RequestId: %s", result.CommonMsg.RequestId); console.log('Owner:'); console.log('ID: %s', result.InterfaceResult.Owner.ID); console.log('Name: %s', result.InterfaceResult.Owner.Name); console.log('Buckets:'); for (let i = 0; i < result.InterfaceResult.Buckets.length; i++) { const val = result.InterfaceResult.Buckets[i]; console.log("Bucket[%d]-Name:%s,CreationDate:%s,Location: %s", i, val.BucketName, val.CreationDate, val.Location); }; return; }; console.log("An ObsError was found, which means your request sent to OBS was rejected with an error response."); console.log("Status: %d", result.CommonMsg.Status); console.log("Code: %s", result.CommonMsg.Code); console.log("Message: %s", result.CommonMsg.Message); console.log("RequestId: %s", result.CommonMsg.RequestId); } catch (error) { console.log("An Exception was found, which means the client encountered an internal problem when attempting to communicate with OBS, for example, the client was unable to access the network."); console.log(error); }; }; listBuckets(); |
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.