Setting Website Hosting
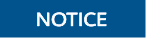
If you have any questions during development, post them on the Issues page of GitHub. For details about parameters and usage of each API, see the .
You can call ObsClient.setBucketWebsite to set website hosting for a bucket.
Configuring the Default Homepage and Error Pages
Sample code:
// Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY_ID. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/eu/usermanual-ca/ca_01_0003.html. String ak = System.getenv("ACCESS_KEY_ID"); String sk = System.getenv("SECRET_ACCESS_KEY_ID"); String endPoint = "https://your-endpoint"; // Create an ObsClient instance. ObsClient obsClient = new ObsClient(ak, sk, endPoint); WebsiteConfiguration config = new WebsiteConfiguration(); // Configure the default homepage. config.setSuffix("index.html"); // Configure the error pages. config.setKey("error.html"); obsClient.setBucketWebsite("bucketname", config);
Configuring the Redirection Rules
Sample code:
// Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY_ID. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/eu/usermanual-ca/ca_01_0003.html. String ak = System.getenv("ACCESS_KEY_ID"); String sk = System.getenv("SECRET_ACCESS_KEY_ID"); String endPoint = "https://your-endpoint"; // Create an ObsClient instance. ObsClient obsClient = new ObsClient(ak, sk, endPoint); WebsiteConfiguration config = new WebsiteConfiguration(); // Configure the default homepage. config.setSuffix("index.html"); // Configure the error pages. config.setKey("error.html"); RouteRule rule = new RouteRule(); Redirect r = new Redirect(); r.setHostName("www.example.com"); r.setHttpRedirectCode("305"); r.setRedirectProtocol(ProtocolEnum.HTTP); r.setReplaceKeyPrefixWith("replacekeyprefix"); rule.setRedirect(r); RouteRuleCondition condition = new RouteRuleCondition(); condition.setHttpErrorCodeReturnedEquals("404"); condition.setKeyPrefixEquals("keyprefix"); rule.setCondition(condition); config.getRouteRules().add(rule); obsClient.setBucketWebsite("bucketname", config);
Configuring Redirection for All Requests
Sample code:
// Hard-coded or plaintext AK/SK are risky. For security purposes, encrypt your AK/SK and store them in the configuration file or environment variables. In this example, the AK/SK are stored in environment variables for identity authentication. Before running this example, configure environment variables ACCESS_KEY_ID and SECRET_ACCESS_KEY_ID. // Obtain an AK/SK pair on the management console. For details, see https://support.huaweicloud.com/eu/usermanual-ca/ca_01_0003.html. String ak = System.getenv("ACCESS_KEY_ID"); String sk = System.getenv("SECRET_ACCESS_KEY_ID"); String endPoint = "https://your-endpoint"; // Create an ObsClient instance. ObsClient obsClient = new ObsClient(ak, sk, endPoint); WebsiteConfiguration config = new WebsiteConfiguration(); RedirectAllRequest redirectAll = new RedirectAllRequest(); redirectAll.setHostName("www.example.com"); // Configure the support for both HTTP and HTTPS. redirectAll.setRedirectProtocol(ProtocolEnum.HTTP); config.setRedirectAllRequestsTo(redirectAll); obsClient.setBucketWebsite("bucketname", config);
Feedback
Was this page helpful?
Provide feedbackThank you very much for your feedback. We will continue working to improve the documentation.